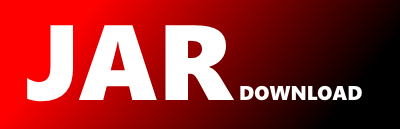
org.unitils.ftp.FtpModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unitils-ftp Show documentation
Show all versions of unitils-ftp Show documentation
A fake FTP server where you can easily test if a file is correctly retrieved from the server.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy