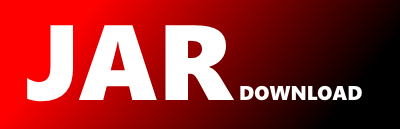
org.unitils.database.transaction.impl.DefaultUnitilsTransactionManager Maven / Gradle / Ivy
/*
* Copyright 2008, Unitils.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.unitils.database.transaction.impl;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.jdbc.datasource.TransactionAwareDataSourceProxy;
import org.springframework.transaction.PlatformTransactionManager;
import org.springframework.transaction.TransactionDefinition;
import org.springframework.transaction.TransactionStatus;
import org.springframework.transaction.support.DefaultTransactionDefinition;
import org.unitils.core.UnitilsException;
import org.unitils.database.transaction.UnitilsTransactionManager;
import javax.sql.DataSource;
import java.util.*;
/**
* Implements transactions for unit tests, by delegating to a spring
* PlatformTransactionManager
. The concrete implementation of
* PlatformTransactionManager
that is used depends on the test
* class. If a custom PlatformTransactionManager
was configured
* in a spring ApplicationContext
, this one is used. If not, a
* suitable subclass of PlatformTransactionManager
is created,
* depending on the configuration of a test. E.g. if some ORM persistence unit
* was configured on the test, a PlatformTransactionManager
that
* can offer transactional behavior for such a persistence unit is used. If no
* such configuration is found, a DataSourceTransactionManager
is
* used.
*
* @author Filip Neven
* @author Tim Ducheyne
*/
public class DefaultUnitilsTransactionManager implements UnitilsTransactionManager {
/**
* The logger instance for this class
*/
private static Log logger = LogFactory.getLog(DefaultUnitilsTransactionManager.class);
protected Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy