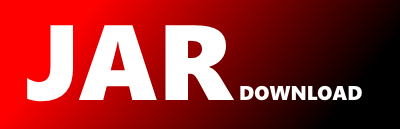
org.unix4j.convert.ListConverters Maven / Gradle / Ivy
package org.unix4j.convert;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
public class ListConverters {
public static final ValueConverter> COLLECTION_TO_LIST = new ValueConverter>() {
@Override
public List> convert(Object value) throws IllegalArgumentException {
if (value instanceof Collection) {
if (value instanceof List) {
return (List>)value;
}
return new ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy