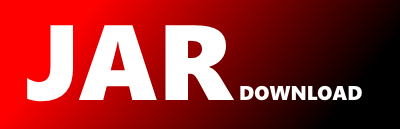
org.usergrid.security.tokens.TokenInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of usergrid-services Show documentation
Show all versions of usergrid-services Show documentation
Service layer for Usergrid system.
package org.usergrid.security.tokens;
import java.util.Map;
import java.util.UUID;
import org.usergrid.security.AuthPrincipalInfo;
public class TokenInfo {
UUID uuid;
String type;
long created;
long accessed;
long inactive;
// total duration, in milliseconds
long duration;
AuthPrincipalInfo principal;
Map state;
public TokenInfo(UUID uuid, String type, long created, long accessed, long inactive, long duration,
AuthPrincipalInfo principal, Map state) {
this.uuid = uuid;
this.type = type;
this.created = created;
this.accessed = accessed;
this.inactive = inactive;
this.principal = principal;
this.duration = duration;
this.state = state;
}
public UUID getUuid() {
return uuid;
}
public void setUuid(UUID uuid) {
this.uuid = uuid;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public long getCreated() {
return created;
}
public void setCreated(long created) {
this.created = created;
}
/**
* @return the expiration
*/
public long getDuration() {
return duration;
}
/**
* If the expiration is undefined, return the default that's been passed in
*
* @param defaultExpiration
* @return
*/
public long getExpiration(long defaultExpiration) {
if (duration == 0) {
return defaultExpiration;
}
return duration;
}
/**
* @param expiration
* the expiration to set
*/
public void setDuration(long expiration) {
this.duration = expiration;
}
public long getAccessed() {
return accessed;
}
public void setAccessed(long accessed) {
this.accessed = accessed;
}
public long getInactive() {
return inactive;
}
public void setInactive(long inactive) {
this.inactive = inactive;
}
public AuthPrincipalInfo getPrincipal() {
return principal;
}
public void setPrincipal(AuthPrincipalInfo principal) {
this.principal = principal;
}
public Map getState() {
return state;
}
public void setState(Map state) {
this.state = state;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy