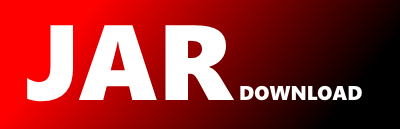
org.vectomatic.dom.svg.OMElement Maven / Gradle / Ivy
/**********************************************
* Copyright (C) 2010 Lukas Laag
* This file is part of lib-gwt-svg.
*
* libgwtsvg is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* libgwtsvg is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with libgwtsvg. If not, see http://www.gnu.org/licenses/
**********************************************/
/*
* Copyright (c) 2004 World Wide Web Consortium,
*
* (Massachusetts Institute of Technology, European Research Consortium for
* Informatics and Mathematics, Keio University). All Rights Reserved. This
* work is distributed under the W3C(r) Software License [1] in the hope that
* it will be useful, but WITHOUT ANY WARRANTY; without even the implied
* warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
*
* [1] http://www.w3.org/Consortium/Legal/2002/copyright-software-20021231
*/
package org.vectomatic.dom.svg;
import org.vectomatic.dom.svg.utils.DOMHelper;
import org.w3c.dom.DOMException;
import com.google.gwt.core.client.JavaScriptException;
import com.google.gwt.dom.client.Element;
/**
* Wrapper class for DOM Element
* @author laaglu
*/
public class OMElement extends OMNode {
/**
* Constructor
* @param element The wrapped element
*/
protected OMElement(Element element) {
super(element);
}
/**
* Returns the wrapped Element
* @return the wrapped Element
*/
public Element getElement() {
return ot.cast();
}
// Implementation of the dom::Element W3C IDL interface
/**
* Returns a OMNodeList
of all descendant OMElements
* with a given tag name, in document order.
* @param name The name of the tag to match on. The special value "*"
* matches all tags.
* @return A list of matching OMElement
nodes.
*/
public final OMNodeList getElementsByTagName(String name) {
return new OMNodeList(((Element) ot).getElementsByTagName(name));
}
/**
* Returns a OMNodeList
of all the descendant
* OMElements
with a given local name and namespace URI in
* document order.
* @param namespaceURI The namespace URI of the elements to match on. The
* special value "*" matches all namespaces.
* @param localName The local name of the elements to match on. The
* special value "*" matches all local names.
* @return A new OMNodeList
object containing all the matched
* OMElements
.
* @exception DOMException
* NOT_SUPPORTED_ERR: May be raised if the implementation does not
* support the feature "XML"
and the language exposed
* through the Document does not support XML Namespaces (such as [HTML 4.01]).
*/
public final OMNodeList getElementsByTagNameNS(String namespaceURI, String localName) throws JavaScriptException {
return new OMNodeList(DOMHelper.getElementsByTagNameNS(((Element) ot), namespaceURI, localName));
}
/**
* Returns the element id
* @return the element id
*/
public final String getId() {
return ((Element) ot).getId();
}
/**
* The name of the element. If Node.localName
is different
* from null
, this attribute is a qualified name. For
* example, in:
* <elementExample id="demo"> ...
* </elementExample> ,
* tagName
has the value
* "elementExample"
. Note that this is case-preserving in
* XML, as are all of the operations of the DOM. The HTML DOM returns
* the tagName
of an HTML element in the canonical
* uppercase form, regardless of the case in the source HTML document.
* @return the name of the element
*/
public final String getTagName() {
return ((Element) ot).getTagName();
}
/**
* Determines whether an element has an attribute with a given name.
*
* Note that IE, prior to version 8, will return false-positives for names
* that collide with element properties (e.g., style, width, and so forth).
*
*
* @param name
* the name of the attribute
* @return true
if this element has the specified attribute
*/
public final boolean hasAttribute(String name) {
return ((Element) ot).hasAttribute(name);
}
/**
* Returns true
when an attribute with a given local name and
* namespace URI is specified on the specified element or has a default value,
* false
otherwise.
*
Per [XML Namespaces]
* , applications must use the value null
as the
* namespaceURI
parameter for methods if they wish to have
* no namespace.
* @param namespaceURI The namespace URI of the attribute to look for.
* @param localName The local name of the attribute to look for.
* @return true
if an attribute with the given local name
* and namespace URI is specified or has a default value on this
* element, false
otherwise.
* @exception DOMException
* NOT_SUPPORTED_ERR: May be raised if the implementation does not
* support the feature "XML"
and the language exposed
* through the Document does not support XML Namespaces (such as [HTML 4.01]).
*/
public final boolean hasAttributeNS(String namespaceURI, String localName) throws JavaScriptException {
return DOMHelper.hasAttributeNS((Element) ot, namespaceURI, localName);
}
/**
* Retrieves an attribute value by name.
* @param name The name of the attribute to retrieve.
* @return The OMAttr
value as a string, or the empty string
* if that attribute does not have a specified or default value.
*/
public final String getAttribute(String name) {
return ((Element) ot).getAttribute(name);
}
/**
* Retrieves an attribute node by name on the specified element.
*
To retrieve an attribute node by qualified name and namespace URI,
* use the getAttributeNodeNS
method.
* @param attrName The name (nodeName
) of the attribute to
* retrieve.
* @return The {@link OMAttr} node with the specified name (
* nodeName
) or null
if there is no such
* attribute.
*/
public final OMAttr getAttributeNode(String attrName) {
return OMNode.convert(DOMHelper.getAttributeNode((Element) ot, attrName));
}
/**
* Retrieves an attribute value by local name and namespace URI.
*
Per [XML Namespaces]
* , applications must use the value null
as the
* namespaceURI
parameter for methods if they wish to have
* no namespace.
* @param namespaceURI The namespace URI of the attribute to retrieve.
* @param localName The local name of the attribute to retrieve.
* @return The OMAttr
value as a string, or the empty string
* if that attribute does not have a specified or default value.
* @exception DOMException
* NOT_SUPPORTED_ERR: May be raised if the implementation does not
* support the feature "XML"
and the language exposed
* through the Document does not support XML Namespaces (such as [HTML 4.01]).
*/
public final String getAttributeNS(String namespaceURI, String localName) throws JavaScriptException {
return DOMHelper.getAttributeNS((Element) ot, namespaceURI, localName);
}
/**
* Returns a {@link OMNamedNodeMap} containing the attributes of the
* specified element.
* @return a {@link OMNamedNodeMap} containing the attributes of the
* specified element
*/
public final OMNamedNodeMap getAttributes() {
return new OMNamedNodeMap(DOMHelper.getAttributes((Element) ot));
}
/**
* Adds a new attribute. If an attribute with that name is already present
* in the element, its value is changed to be that of the value
* parameter. This value is a simple string; it is not parsed as it is
* being set. So any markup (such as syntax to be recognized as an
* entity reference) is treated as literal text, and needs to be
* appropriately escaped by the implementation when it is written out.
*
To set an attribute with a qualified name and namespace URI, use
* the setAttributeNS
method.
* @param name The name of the attribute to create or alter.
* @param value Value to set in string form.
* @exception DOMException
* INVALID_CHARACTER_ERR: Raised if the specified name is not an XML
* name according to the XML version in use specified in the
* Document.xmlVersion
attribute.
*
NO_MODIFICATION_ALLOWED_ERR: Raised if this node is readonly.
*/
public final void setAttribute(String name, String value) throws JavaScriptException {
((Element) ot).setAttribute(name, value);
}
/**
* Adds a new attribute to the specified element. If an attribute with the same local name and
* namespace URI is already present on the element, its prefix is
* changed to be the prefix part of the qualifiedName
, and
* its value is changed to be the value
parameter. This
* value is a simple string; it is not parsed as it is being set. So any
* markup (such as syntax to be recognized as an entity reference) is
* treated as literal text, and needs to be appropriately escaped by the
* implementation when it is written out. In order to assign an
* attribute value that contains entity references, the user must create
* an {@link OMAttr} node plus any {@link OMText} and
* EntityReference
nodes, build the appropriate subtree,
* and use setAttributeNodeNS
or
* setAttributeNode
to assign it as the value of an
* attribute.
*
Per [XML Namespaces]
* , applications must use the value null
as the
* namespaceURI
parameter for methods if they wish to have
* no namespace.
* @param namespaceURI The namespace URI of the attribute to create or
* alter.
* @param localName The local name of the attribute to create or
* alter.
* @param value The value to set in string form.
* @exception DOMException
* INVALID_CHARACTER_ERR: Raised if the specified qualified name is not
* an XML name according to the XML version in use specified in the
* Document.xmlVersion
attribute.
*
NO_MODIFICATION_ALLOWED_ERR: Raised if this node is readonly.
*
NAMESPACE_ERR: Raised if the qualifiedName
is
* malformed per the Namespaces in XML specification, if the
* qualifiedName
has a prefix and the
* namespaceURI
is null
, if the
* qualifiedName
has a prefix that is "xml" and the
* namespaceURI
is different from "
* http://www.w3.org/XML/1998/namespace", if the qualifiedName
or its prefix is "xmlns" and the
* namespaceURI
is different from "http://www.w3.org/2000/xmlns/", or if the namespaceURI
is "http://www.w3.org/2000/xmlns/" and neither the qualifiedName
nor its prefix is "xmlns".
*
NOT_SUPPORTED_ERR: May be raised if the implementation does not
* support the feature "XML"
and the language exposed
* through the Document does not support XML Namespaces (such as [HTML 4.01]).
*/
public final void setAttributeNS(String namespaceURI, String localName, String value) throws JavaScriptException {
DOMHelper.setAttributeNS((Element) ot, namespaceURI, localName, value);
}
/**
* Adds a new attribute node to the specified element. If an attribute with that name (
* nodeName
) is already present in the element, it is
* replaced by the new one. Replacing an attribute node by itself has no
* effect.
*
To add a new attribute node with a qualified name and namespace
* URI, use the setAttributeNodeNS
method.
* @param attr The {@link OMAttr} node to add to the attribute list.
* @return If the attr
attribute replaces an existing
* attribute, the replaced {@link OMAttr} node is returned,
* otherwise null
is returned.
* @exception DOMException
* WRONG_DOCUMENT_ERR: Raised if attr
was created from a
* different document than the one that created the element.
*
NO_MODIFICATION_ALLOWED_ERR: Raised if this node is readonly.
*
INUSE_ATTRIBUTE_ERR: Raised if attr
is already an
* attribute of another Element
object. The DOM user must
* explicitly clone {@link OMAttr} nodes to re-use them in other
* elements.
*/
public final OMAttr setAttributeNode(OMAttr attr) throws JavaScriptException {
return OMNode.convert(DOMHelper.setAttributeNode((Element) ot, attr.getAttr()));
}
/**
* Removes an attribute by name. If a default value for the removed
* attribute is defined in the DTD, a new attribute immediately appears
* with the default value as well as the corresponding namespace URI,
* local name, and prefix when applicable. The implementation may handle
* default values from other schemas similarly but applications should
* use OMDocument.normalizeDocument()
to guarantee this
* information is up-to-date.
*
If no attribute with this name is found, this method has no effect.
*
To remove an attribute by local name and namespace URI, use the
* removeAttributeNS
method.
* @param name The name of the attribute to remove.
* @exception DOMException
* NO_MODIFICATION_ALLOWED_ERR: Raised if this node is readonly.
*/
public final void removeAttribute(String name) throws JavaScriptException {
((Element) ot).removeAttribute(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy