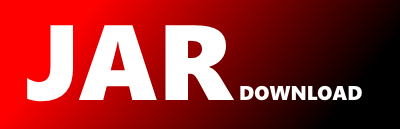
org.vectomatic.dom.svg.OMSVGDefsElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lib-gwt-svg Show documentation
Show all versions of lib-gwt-svg Show documentation
A GWT library to access the SVG functionalities of web browsers
/**********************************************
* Copyright (C) 2010-2011 Lukas Laag
* This file is part of lib-gwt-svg.
*
* libgwtsvg is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* libgwtsvg is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with libgwtsvg. If not, see http://www.gnu.org/licenses/
**********************************************/
/*
* Copyright (c) 2004 World Wide Web Consortium,
*
* (Massachusetts Institute of Technology, European Research Consortium for
* Informatics and Mathematics, Keio University). All Rights Reserved. This
* work is distributed under the W3C(r) Software License [1] in the hope that
* it will be useful, but WITHOUT ANY WARRANTY; without even the implied
* warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
*
* [1] http://www.w3.org/Consortium/Legal/2002/copyright-software-20021231
*/
package org.vectomatic.dom.svg;
import org.vectomatic.dom.svg.events.ActivateEvent;
import org.vectomatic.dom.svg.events.ActivateHandler;
import org.vectomatic.dom.svg.events.FocusInEvent;
import org.vectomatic.dom.svg.events.FocusInHandler;
import org.vectomatic.dom.svg.events.FocusOutEvent;
import org.vectomatic.dom.svg.events.FocusOutHandler;
import org.vectomatic.dom.svg.events.HasGraphicalHandlers;
import org.vectomatic.dom.svg.impl.SVGDefsElement;
import org.vectomatic.dom.svg.impl.SVGElement;
import org.vectomatic.dom.svg.itf.ISVGContainerElement;
import org.vectomatic.dom.svg.itf.ISVGExternalResourcesRequired;
import org.vectomatic.dom.svg.itf.ISVGLangSpace;
import org.vectomatic.dom.svg.itf.ISVGStylable;
import org.vectomatic.dom.svg.itf.ISVGTests;
import org.vectomatic.dom.svg.itf.ISVGTransformable;
import org.vectomatic.dom.svg.utils.DOMHelper;
import org.vectomatic.dom.svg.utils.SVGConstants;
import com.google.gwt.core.client.JavaScriptException;
import com.google.gwt.dom.client.TagName;
import com.google.gwt.event.dom.client.ClickEvent;
import com.google.gwt.event.dom.client.ClickHandler;
import com.google.gwt.event.dom.client.DragEndEvent;
import com.google.gwt.event.dom.client.DragEndHandler;
import com.google.gwt.event.dom.client.DragEnterEvent;
import com.google.gwt.event.dom.client.DragEnterHandler;
import com.google.gwt.event.dom.client.DragEvent;
import com.google.gwt.event.dom.client.DragHandler;
import com.google.gwt.event.dom.client.DragLeaveEvent;
import com.google.gwt.event.dom.client.DragLeaveHandler;
import com.google.gwt.event.dom.client.DragOverEvent;
import com.google.gwt.event.dom.client.DragOverHandler;
import com.google.gwt.event.dom.client.DragStartEvent;
import com.google.gwt.event.dom.client.DragStartHandler;
import com.google.gwt.event.dom.client.DropEvent;
import com.google.gwt.event.dom.client.DropHandler;
import com.google.gwt.event.dom.client.LoadEvent;
import com.google.gwt.event.dom.client.LoadHandler;
import com.google.gwt.event.dom.client.MouseDownEvent;
import com.google.gwt.event.dom.client.MouseDownHandler;
import com.google.gwt.event.dom.client.MouseMoveEvent;
import com.google.gwt.event.dom.client.MouseMoveHandler;
import com.google.gwt.event.dom.client.MouseOutEvent;
import com.google.gwt.event.dom.client.MouseOutHandler;
import com.google.gwt.event.dom.client.MouseOverEvent;
import com.google.gwt.event.dom.client.MouseOverHandler;
import com.google.gwt.event.dom.client.MouseUpEvent;
import com.google.gwt.event.dom.client.MouseUpHandler;
import com.google.gwt.event.dom.client.TouchCancelEvent;
import com.google.gwt.event.dom.client.TouchCancelHandler;
import com.google.gwt.event.dom.client.TouchEndEvent;
import com.google.gwt.event.dom.client.TouchEndHandler;
import com.google.gwt.event.dom.client.TouchMoveEvent;
import com.google.gwt.event.dom.client.TouchMoveHandler;
import com.google.gwt.event.dom.client.TouchStartEvent;
import com.google.gwt.event.dom.client.TouchStartHandler;
import com.google.gwt.event.shared.HandlerRegistration;
/**
* The {@link org.vectomatic.dom.svg.OMSVGDefsElement} interface corresponds
* to the defs element.
*/
@TagName("defs")
public class OMSVGDefsElement extends OMSVGElement implements HasGraphicalHandlers, ISVGTests, ISVGLangSpace, ISVGExternalResourcesRequired, ISVGStylable, ISVGTransformable, ISVGContainerElement {
public OMSVGDefsElement() {
this((SVGDefsElement)DOMHelper.createElementNS(DOMHelper.getCurrentDocument(), SVGConstants.SVG_NAMESPACE_URI, SVGConstants.SVG_DEFS_TAG).cast());
}
protected OMSVGDefsElement(SVGDefsElement ot) {
super(ot);
}
// Implementation of the svg::SVGDefsElement W3C IDL interface
// Implementation of the svg::SVGExternalResourcesRequired W3C IDL interface
/**
* Corresponds to attribute {@link org.vectomatic.dom.svg.itf.ISVGExternalResourcesRequired#getExternalResourcesRequired()}
* on the given element. Note that the SVG DOM defines the attribute {@link
* org.vectomatic.dom.svg.itf.ISVGExternalResourcesRequired#getExternalResourcesRequired()}
* as being of type {@link org.vectomatic.dom.svg.OMSVGAnimatedBoolean}, whereas
* the SVG language definition says that {@link org.vectomatic.dom.svg.itf.ISVGExternalResourcesRequired#getExternalResourcesRequired()}
* is not animated. Because the SVG language definition states that {@link
* org.vectomatic.dom.svg.itf.ISVGExternalResourcesRequired#getExternalResourcesRequired()}
* cannot be animated, the {@link org.vectomatic.dom.svg.OMSVGAnimatedBoolean#getAnimVal()}
* will always be the same as the {@link org.vectomatic.dom.svg.OMSVGAnimatedBoolean#getBaseVal()}.
*/
public final OMSVGAnimatedBoolean getExternalResourcesRequired() {
return ((SVGDefsElement)ot).getExternalResourcesRequired();
}
// Implementation of the svg::SVGLangSpace W3C IDL interface
/**
* Corresponds to attribute xml:lang
on the given element.
*/
public final String getXmllang() {
return ((SVGDefsElement)ot).getXmllang();
}
/**
* Corresponds to attribute xml:lang
on the given element.
* @throws DOMException(NO_MODIFICATION_ALLOWED_ERR) Raised on an attempt
* to change the value of a read only
* attribute.
*/
public final void setXmllang(java.lang.String value) throws JavaScriptException {
((SVGDefsElement)ot).setXmllang(value);
}
/**
* Corresponds to attribute xml:space
on the given element.
*/
public final String getXmlspace() {
return ((SVGDefsElement)ot).getXmlspace();
}
/**
* Corresponds to attribute xml:space
on the given element.
* @throws DOMException(NO_MODIFICATION_ALLOWED_ERR) Raised on an attempt
* to change the value of a read only
* attribute.
*/
public final void setXmlspace(java.lang.String value) throws JavaScriptException {
((SVGDefsElement)ot).setXmlspace(value);
}
// Implementation of the svg::SVGLocatable W3C IDL interface
/**
* The element which established the current viewport. Often, the nearest
* ancestor svg element. Null if the current element is
* the outermost svg element.
*/
public final OMSVGElement getNearestViewportElement() {
SVGElement elt = ((SVGDefsElement)ot).getNearestViewportElement();
return elt != null ? (OMSVGElement)convert(elt) : null;
}
/**
* The farthest ancestor svg element. Null if the current
* element is the outermost svg element.
*/
public final OMSVGElement getFarthestViewportElement() {
SVGElement elt = ((SVGDefsElement)ot).getFarthestViewportElement();
return elt != null ? (OMSVGElement)convert(elt) : null;
}
/**
* Returns the tight bounding box in current user space (i.e., after application
* of the transform
attribute, if any) on the geometry of all
* contained graphics elements, exclusive of stroking, clipping, masking and
* filter effects). Note that getBBox must return the actual bounding box
* at the time the method was called, even in case the element has not yet
* been rendered.
* @return An {@link org.vectomatic.dom.svg.OMSVGRect} object that defines
* the bounding box.
*/
public final OMSVGRect getBBox() {
return ((SVGDefsElement)ot).getBBox();
}
/**
* Returns the transformation matrix from current user units (i.e., after
* application of the transform
attribute, if any) to the viewport
* coordinate system for the {@link org.vectomatic.dom.svg.itf.ISVGLocatable#getNearestViewportElement()}.
* @return An {@link org.vectomatic.dom.svg.OMSVGMatrix} object that defines
* the CTM.
*/
public final OMSVGMatrix getCTM() {
return ((SVGDefsElement)ot).getCTM();
}
/**
* Returns the transformation matrix from current user units (i.e., after
* application of the transform
attribute, if any) to the parent
* user agent's notice of a "pixel". For display devices, ideally this represents
* a physical screen pixel. For other devices or environments where physical
* pixel sizes are not known, then an algorithm similar to the CSS2 definition
* of a "pixel" can be used instead. Note that null is returned if this element
* is not hooked into the document tree. This method would have been more
* aptly named as getClientCTM
, but the name getScreenCTM
* is kept for historical reasons.
* @return An {@link org.vectomatic.dom.svg.OMSVGMatrix} object that defines
* the given transformation matrix.
*/
public final OMSVGMatrix getScreenCTM() {
return ((SVGDefsElement)ot).getScreenCTM();
}
/**
* Returns the transformation matrix from the user coordinate system on the
* current element (after application of the transform
attribute,
* if any) to the user coordinate system on parameter element (after
* application of its transform
attribute, if any).
* @param element The target element.
* @return An {@link org.vectomatic.dom.svg.OMSVGMatrix} object that defines
* the transformation.
* @throws SVGException(SVG_MATRIX_NOT_INVERTABLE) Raised if the currently
* defined transformation matrices make it impossible to compute the given
* matrix (e.g., because one of the transformations is singular).
*/
public final OMSVGMatrix getTransformToElement(OMSVGElement element) throws JavaScriptException {
return ((SVGDefsElement)ot).getTransformToElement(((SVGElement)element.ot));
}
// Implementation of the svg::SVGTests W3C IDL interface
/**
* Corresponds to attribute {@link org.vectomatic.dom.svg.itf.ISVGTests#getRequiredFeatures()}
* on the given element.
*/
public final OMSVGStringList getRequiredFeatures() {
return ((SVGDefsElement)ot).getRequiredFeatures();
}
/**
* Corresponds to attribute {@link org.vectomatic.dom.svg.itf.ISVGTests#getRequiredExtensions()}
* on the given element.
*/
public final OMSVGStringList getRequiredExtensions() {
return ((SVGDefsElement)ot).getRequiredExtensions();
}
/**
* Corresponds to attribute {@link org.vectomatic.dom.svg.itf.ISVGTests#getSystemLanguage()}
* on the given element.
*/
public final OMSVGStringList getSystemLanguage() {
return ((SVGDefsElement)ot).getSystemLanguage();
}
/**
* Returns true if the user agent supports the given extension, specified
* by a URI.
* @param extension The name of the extension, expressed as a URI.
* @return True or false, depending on whether the given extension is supported.
*/
public final boolean hasExtension(String extension) {
return ((SVGDefsElement)ot).hasExtension(extension);
}
// Implementation of the svg::SVGTransformable W3C IDL interface
/**
* Corresponds to attribute {@link org.vectomatic.dom.svg.itf.ISVGTransformable#getTransform()}
* on the given element.
*/
public final OMSVGAnimatedTransformList getTransform() {
return ((SVGDefsElement)ot).getTransform();
}
@Override
public final HandlerRegistration addClickHandler(ClickHandler handler) {
return addDomHandler(handler, ClickEvent.getType());
}
@Override
public final HandlerRegistration addDragEndHandler(DragEndHandler handler) {
return addDomHandler(handler, DragEndEvent.getType());
}
@Override
public final HandlerRegistration addDragEnterHandler(DragEnterHandler handler) {
return addDomHandler(handler, DragEnterEvent.getType());
}
@Override
public final HandlerRegistration addDragHandler(DragHandler handler) {
return addDomHandler(handler, DragEvent.getType());
}
@Override
public final HandlerRegistration addDragLeaveHandler(DragLeaveHandler handler) {
return addDomHandler(handler, DragLeaveEvent.getType());
}
@Override
public final HandlerRegistration addDragOverHandler(DragOverHandler handler) {
return addDomHandler(handler, DragOverEvent.getType());
}
@Override
public final HandlerRegistration addDragStartHandler(DragStartHandler handler) {
return addDomHandler(handler, DragStartEvent.getType());
}
@Override
public final HandlerRegistration addDropHandler(DropHandler handler) {
return addDomHandler(handler, DropEvent.getType());
}
@Override
public final HandlerRegistration addLoadHandler(LoadHandler handler) {
return addDomHandler(handler, LoadEvent.getType());
}
@Override
public final HandlerRegistration addMouseDownHandler(MouseDownHandler handler) {
return addDomHandler(handler, MouseDownEvent.getType());
}
@Override
public final HandlerRegistration addMouseMoveHandler(MouseMoveHandler handler) {
return addDomHandler(handler, MouseMoveEvent.getType());
}
@Override
public final HandlerRegistration addMouseOutHandler(MouseOutHandler handler) {
return addDomHandler(handler, MouseOutEvent.getType());
}
@Override
public final HandlerRegistration addMouseOverHandler(MouseOverHandler handler) {
return addDomHandler(handler, MouseOverEvent.getType());
}
@Override
public final HandlerRegistration addMouseUpHandler(MouseUpHandler handler) {
return addDomHandler(handler, MouseUpEvent.getType());
}
@Override
public final HandlerRegistration addTouchCancelHandler(TouchCancelHandler handler) {
return addDomHandler(handler, TouchCancelEvent.getType());
}
@Override
public final HandlerRegistration addTouchEndHandler(TouchEndHandler handler) {
return addDomHandler(handler, TouchEndEvent.getType());
}
@Override
public final HandlerRegistration addTouchMoveHandler(TouchMoveHandler handler) {
return addDomHandler(handler, TouchMoveEvent.getType());
}
@Override
public final HandlerRegistration addTouchStartHandler(TouchStartHandler handler) {
return addDomHandler(handler, TouchStartEvent.getType());
}
@Override
public final HandlerRegistration addActivateHandler(ActivateHandler handler) {
return addDomHandler(handler, ActivateEvent.getType());
}
@Override
public final HandlerRegistration addFocusInHandler(FocusInHandler handler) {
return addDomHandler(handler, FocusInEvent.getType());
}
@Override
public final HandlerRegistration addFocusOutHandler(FocusOutHandler handler) {
return addDomHandler(handler, FocusOutEvent.getType());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy