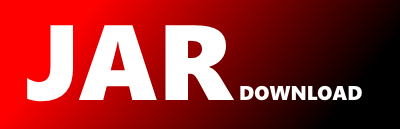
org.vertexium.accumulo.AccumuloEdge Maven / Gradle / Ivy
package org.vertexium.accumulo;
import com.google.common.base.Function;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Iterables;
import org.apache.accumulo.core.data.Key;
import org.apache.accumulo.core.data.Value;
import org.apache.hadoop.io.Text;
import org.vertexium.*;
import org.vertexium.accumulo.iterator.EdgeIterator;
import org.vertexium.accumulo.iterator.model.ElementData;
import org.vertexium.accumulo.util.DataInputStreamUtils;
import org.vertexium.mutation.ExistingEdgeMutation;
import org.vertexium.mutation.PropertyDeleteMutation;
import org.vertexium.mutation.PropertySoftDeleteMutation;
import javax.annotation.Nullable;
import java.io.ByteArrayInputStream;
import java.io.DataInputStream;
import java.io.IOException;
import java.util.List;
public class AccumuloEdge extends AccumuloElement implements Edge {
public static final Text CF_SIGNAL = EdgeIterator.CF_SIGNAL;
public static final Text CF_OUT_VERTEX = EdgeIterator.CF_OUT_VERTEX;
public static final Text CF_IN_VERTEX = EdgeIterator.CF_IN_VERTEX;
private final String outVertexId;
private final String inVertexId;
private final String label;
private final String newEdgeLabel;
public AccumuloEdge(
Graph graph,
String id,
String outVertexId,
String inVertexId,
String label,
String newEdgeLabel,
Visibility visibility,
Iterable properties,
Iterable propertyDeleteMutations,
Iterable propertySoftDeleteMutations,
Iterable hiddenVisibilities,
ImmutableSet extendedDataTableNames,
long timestamp,
FetchHints fetchHints,
Authorizations authorizations
) {
super(
graph,
id,
visibility,
properties,
propertyDeleteMutations,
propertySoftDeleteMutations,
hiddenVisibilities,
extendedDataTableNames,
timestamp,
fetchHints,
authorizations
);
this.outVertexId = outVertexId;
this.inVertexId = inVertexId;
this.label = label;
this.newEdgeLabel = newEdgeLabel;
}
public static Edge createFromIteratorValue(
AccumuloGraph graph,
Key key,
Value value,
FetchHints fetchHints,
Authorizations authorizations
) {
try {
String edgeId;
Visibility vertexVisibility;
Iterable properties;
Iterable hiddenVisibilities;
long timestamp;
ByteArrayInputStream bain = new ByteArrayInputStream(value.get());
final DataInputStream in = new DataInputStream(bain);
DataInputStreamUtils.decodeHeader(in, ElementData.TYPE_ID_EDGE);
edgeId = DataInputStreamUtils.decodeString(in);
timestamp = in.readLong();
vertexVisibility = new Visibility(DataInputStreamUtils.decodeString(in));
hiddenVisibilities = Iterables.transform(DataInputStreamUtils.decodeStringSet(in), new Function() {
@Nullable
@Override
public Visibility apply(String input) {
return new Visibility(input);
}
});
List metadataEntries = DataInputStreamUtils.decodeMetadataEntries(in);
properties = DataInputStreamUtils.decodeProperties(graph, in, metadataEntries, fetchHints);
ImmutableSet extendedDataTableNames = DataInputStreamUtils.decodeStringSet(in);
String inVertexId = DataInputStreamUtils.decodeString(in);
String outVertexId = DataInputStreamUtils.decodeString(in);
String label = graph.getNameSubstitutionStrategy().inflate(DataInputStreamUtils.decodeString(in));
return new AccumuloEdge(
graph,
edgeId,
outVertexId,
inVertexId,
label,
null,
vertexVisibility,
properties,
null,
null,
hiddenVisibilities,
extendedDataTableNames,
timestamp,
fetchHints,
authorizations
);
} catch (IOException ex) {
throw new VertexiumException("Could not read vertex", ex);
}
}
String getNewEdgeLabel() {
return newEdgeLabel;
}
@Override
public String getLabel() {
return label;
}
@Override
public String getVertexId(Direction direction) {
switch (direction) {
case OUT:
return outVertexId;
case IN:
return inVertexId;
default:
throw new IllegalArgumentException("Unexpected direction: " + direction);
}
}
@Override
public Vertex getVertex(Direction direction, Authorizations authorizations) {
return getVertex(direction, getGraph().getDefaultFetchHints(), authorizations);
}
@Override
public String getOtherVertexId(String myVertexId) {
if (inVertexId.equals(myVertexId)) {
return outVertexId;
} else if (outVertexId.equals(myVertexId)) {
return inVertexId;
}
throw new VertexiumException("myVertexId(" + myVertexId + ") does not appear on edge (" + getId() + ") in either the in (" + inVertexId + ") or the out (" + outVertexId + ").");
}
@Override
public Vertex getOtherVertex(String myVertexId, Authorizations authorizations) {
return getOtherVertex(myVertexId, getGraph().getDefaultFetchHints(), authorizations);
}
@Override
public Vertex getOtherVertex(String myVertexId, FetchHints fetchHints, Authorizations authorizations) {
return getGraph().getVertex(getOtherVertexId(myVertexId), fetchHints, authorizations);
}
@Override
public EdgeVertices getVertices(Authorizations authorizations) {
return getVertices(getGraph().getDefaultFetchHints(), authorizations);
}
@Override
public Vertex getVertex(Direction direction, FetchHints fetchHints, Authorizations authorizations) {
return getGraph().getVertex(getVertexId(direction), fetchHints, authorizations);
}
@Override
@SuppressWarnings("unchecked")
public ExistingEdgeMutation prepareMutation() {
return new ExistingEdgeMutation(this) {
@Override
public Edge save(Authorizations authorizations) {
saveExistingElementMutation(this, authorizations);
return getElement();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy