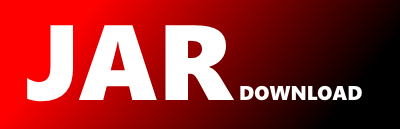
org.vertexium.FetchHint Maven / Gradle / Ivy
package org.vertexium;
import java.util.ArrayList;
import java.util.EnumSet;
import java.util.List;
public enum FetchHint {
PROPERTIES,
PROPERTY_METADATA,
IN_EDGE_REFS,
OUT_EDGE_REFS,
INCLUDE_HIDDEN,
IN_EDGE_LABELS,
OUT_EDGE_LABELS,
EXTENDED_DATA_TABLE_NAMES;
public static final EnumSet NONE = EnumSet.noneOf(FetchHint.class);
public static final EnumSet ALL = EnumSet.of(PROPERTIES, PROPERTY_METADATA, IN_EDGE_REFS, OUT_EDGE_REFS, EXTENDED_DATA_TABLE_NAMES);
public static final EnumSet ALL_INCLUDING_HIDDEN = EnumSet.allOf(FetchHint.class);
public static final EnumSet EDGE_REFS = EnumSet.of(IN_EDGE_REFS, OUT_EDGE_REFS);
public static final EnumSet EDGE_LABELS = EnumSet.of(IN_EDGE_LABELS, OUT_EDGE_LABELS);
public static String toString(EnumSet fetchHints) {
StringBuilder result = new StringBuilder();
boolean first = true;
for (FetchHint fetchHint : fetchHints) {
if (!first) {
result.append(",");
}
result.append(fetchHint.name());
first = false;
}
return result.toString();
}
public static EnumSet parse(String fetchHintsString) {
if (fetchHintsString == null) {
throw new NullPointerException("fetchHintsString cannot be null");
}
String[] parts = fetchHintsString.split(",");
List results = new ArrayList<>();
for (String part : parts) {
String name = part.toUpperCase();
if (name.trim().length() == 0) {
continue;
}
try {
results.add(FetchHint.valueOf(name));
} catch (IllegalArgumentException ex) {
throw new IllegalArgumentException("Could not find enum value: '" + name + "'", ex);
}
}
return create(results);
}
public static EnumSet create(List results) {
if (results.size() == 0) {
return EnumSet.noneOf(FetchHint.class);
} else {
return EnumSet.copyOf(results);
}
}
public static void checkFetchHints(EnumSet fetchHints, FetchHint neededFetchHint) {
checkFetchHints(fetchHints, EnumSet.of(neededFetchHint));
}
public static void checkFetchHints(EnumSet fetchHints, EnumSet neededFetchHints) {
for (FetchHint neededFetchHint : neededFetchHints) {
if (!fetchHints.contains(neededFetchHint)) {
throw new VertexiumMissingFetchHintException(fetchHints, neededFetchHints);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy