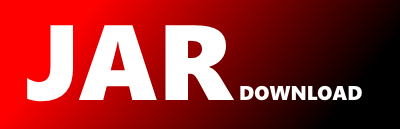
org.vertexium.EdgesSummary Maven / Gradle / Ivy
package org.vertexium;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import java.util.HashMap;
import java.util.Map;
public class EdgesSummary {
private final ImmutableMap outEdgeCountsByLabels;
private final ImmutableMap inEdgeCountsByLabels;
public EdgesSummary(
ImmutableMap outEdgeCountsByLabels,
ImmutableMap inEdgeCountsByLabels
) {
this.outEdgeCountsByLabels = outEdgeCountsByLabels;
this.inEdgeCountsByLabels = inEdgeCountsByLabels;
}
public EdgesSummary(Map outEdgeCountsByLabels, Map inEdgeCountsByLabels) {
this(
ImmutableMap.copyOf(outEdgeCountsByLabels),
ImmutableMap.copyOf(inEdgeCountsByLabels)
);
}
public ImmutableMap getOutEdgeCountsByLabels() {
return outEdgeCountsByLabels;
}
public ImmutableMap getInEdgeCountsByLabels() {
return inEdgeCountsByLabels;
}
public ImmutableMap getEdgeCountsByLabels() {
Map m = new HashMap<>(getOutEdgeCountsByLabels());
for (Map.Entry entry : getInEdgeCountsByLabels().entrySet()) {
Integer v = m.get(entry.getKey());
if (v == null) {
m.put(entry.getKey(), entry.getValue());
} else {
m.put(entry.getKey(), v + entry.getValue());
}
}
return ImmutableMap.copyOf(m);
}
public ImmutableSet getOutEdgeLabels() {
return outEdgeCountsByLabels.keySet();
}
public ImmutableSet getInEdgeLabels() {
return inEdgeCountsByLabels.keySet();
}
public ImmutableSet getEdgeLabels() {
return ImmutableSet.builder()
.addAll(getOutEdgeLabels())
.addAll(getInEdgeLabels())
.build();
}
public ImmutableSet getEdgeLabels(Direction direction) {
if (direction == Direction.IN) {
return getInEdgeLabels();
}
if (direction == Direction.OUT) {
return getOutEdgeLabels();
}
if (direction == Direction.BOTH) {
return getEdgeLabels();
}
throw new VertexiumException("Unsupported direction: " + direction);
}
public int getCountOfOutEdges() {
return outEdgeCountsByLabels.values().stream().mapToInt(l -> l).sum();
}
public int getCountOfInEdges() {
return inEdgeCountsByLabels.values().stream().mapToInt(l -> l).sum();
}
public int getCountOfEdges() {
return getCountOfOutEdges() + getCountOfInEdges();
}
public int getCountOfEdges(Direction direction) {
if (direction == Direction.IN) {
return getCountOfInEdges();
}
if (direction == Direction.OUT) {
return getCountOfOutEdges();
}
if (direction == Direction.BOTH) {
return getCountOfEdges();
}
throw new VertexiumException("Unsupported direction: " + direction);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy