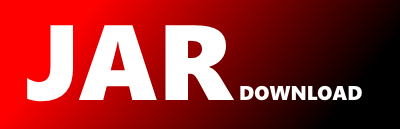
org.vertexium.GraphBaseWithSearchIndex Maven / Gradle / Ivy
package org.vertexium;
import org.vertexium.id.IdGenerator;
import org.vertexium.mutation.ElementMutation;
import org.vertexium.mutation.ExistingElementMutation;
import org.vertexium.query.GraphQuery;
import org.vertexium.query.MultiVertexQuery;
import org.vertexium.query.SimilarToGraphQuery;
import org.vertexium.search.IndexHint;
import org.vertexium.search.SearchIndex;
import org.vertexium.search.SearchIndexWithVertexPropertyCountByValue;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public abstract class GraphBaseWithSearchIndex extends GraphBase implements Graph, GraphWithSearchIndex {
public static final String METADATA_ID_GENERATOR_CLASSNAME = "idGenerator.classname";
private final GraphConfiguration configuration;
private final IdGenerator idGenerator;
private final FetchHints defaultFetchHints;
private SearchIndex searchIndex;
private boolean foundIdGeneratorClassnameInMetadata;
protected GraphBaseWithSearchIndex(GraphConfiguration configuration) {
super(configuration.isStrictTyping(), configuration.createMetricsRegistry());
this.configuration = configuration;
this.searchIndex = configuration.createSearchIndex(this);
this.idGenerator = configuration.createIdGenerator(this);
this.defaultFetchHints = FetchHints.ALL;
}
protected GraphBaseWithSearchIndex(GraphConfiguration configuration, IdGenerator idGenerator, SearchIndex searchIndex) {
super(configuration.isStrictTyping(), configuration.createMetricsRegistry());
this.configuration = configuration;
this.searchIndex = searchIndex;
this.idGenerator = idGenerator;
this.defaultFetchHints = FetchHints.ALL;
}
protected void setup() {
setupGraphMetadata();
}
protected void setupGraphMetadata() {
foundIdGeneratorClassnameInMetadata = false;
for (GraphMetadataEntry graphMetadataEntry : getMetadata()) {
setupGraphMetadata(graphMetadataEntry);
}
if (!foundIdGeneratorClassnameInMetadata) {
setMetadata(METADATA_ID_GENERATOR_CLASSNAME, this.idGenerator.getClass().getName());
}
}
protected void setupGraphMetadata(GraphMetadataEntry graphMetadataEntry) {
if (graphMetadataEntry.getKey().startsWith(METADATA_DEFINE_PROPERTY_PREFIX)) {
if (graphMetadataEntry.getValue() instanceof PropertyDefinition) {
addToPropertyDefinitionCache((PropertyDefinition) graphMetadataEntry.getValue());
} else {
throw new VertexiumException("Invalid property definition metadata: " + graphMetadataEntry.getKey() + " expected " + PropertyDefinition.class.getName() + " found " + graphMetadataEntry.getValue().getClass().getName());
}
} else if (graphMetadataEntry.getKey().equals(METADATA_ID_GENERATOR_CLASSNAME)) {
if (graphMetadataEntry.getValue() instanceof String) {
String idGeneratorClassname = (String) graphMetadataEntry.getValue();
if (idGeneratorClassname.equals(idGenerator.getClass().getName())) {
foundIdGeneratorClassnameInMetadata = true;
}
} else {
throw new VertexiumException("Invalid " + METADATA_ID_GENERATOR_CLASSNAME + " expected String found " + graphMetadataEntry.getValue().getClass().getName());
}
}
}
@Override
public GraphQuery query(Authorizations authorizations) {
return getSearchIndex().queryGraph(this, null, authorizations);
}
@Override
public GraphQuery query(String queryString, Authorizations authorizations) {
return getSearchIndex().queryGraph(this, queryString, authorizations);
}
@Override
public MultiVertexQuery query(String[] vertexIds, String queryString, Authorizations authorizations) {
return getSearchIndex().queryGraph(this, vertexIds, queryString, authorizations);
}
@Override
public MultiVertexQuery query(String[] vertexIds, Authorizations authorizations) {
return getSearchIndex().queryGraph(this, vertexIds, null, authorizations);
}
@Override
public boolean isQuerySimilarToTextSupported() {
return getSearchIndex().isQuerySimilarToTextSupported();
}
@Override
public SimilarToGraphQuery querySimilarTo(String[] fields, String text, Authorizations authorizations) {
return getSearchIndex().querySimilarTo(this, fields, text, authorizations);
}
public IdGenerator getIdGenerator() {
return idGenerator;
}
public GraphConfiguration getConfiguration() {
return configuration;
}
@Override
public SearchIndex getSearchIndex() {
return searchIndex;
}
@Override
public void reindex(Authorizations authorizations) {
reindexVertices(authorizations);
reindexEdges(authorizations);
}
protected void reindexVertices(Authorizations authorizations) {
this.searchIndex.addElements(this, getVertices(authorizations), authorizations);
}
private void reindexEdges(Authorizations authorizations) {
this.searchIndex.addElements(this, getEdges(authorizations), authorizations);
}
@Override
public void flush() {
flushStackTraceTracker.addStackTrace();
if (getSearchIndex() != null) {
this.searchIndex.flush(this);
}
}
@Override
public void shutdown() {
flush();
if (getSearchIndex() != null) {
this.searchIndex.shutdown();
this.searchIndex = null;
}
getMetricsRegistry().shutdown();
}
@Override
public abstract void drop();
@Override
public boolean isFieldBoostSupported() {
return getSearchIndex().isFieldBoostSupported();
}
@Override
public SearchIndexSecurityGranularity getSearchIndexSecurityGranularity() {
return getSearchIndex().getSearchIndexSecurityGranularity();
}
@Override
@Deprecated
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy