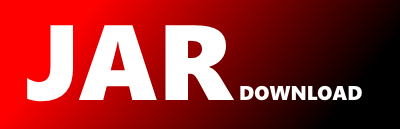
org.vertexium.util.PropertyCollection Maven / Gradle / Ivy
package org.vertexium.util;
import org.vertexium.Property;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentSkipListMap;
import java.util.concurrent.ConcurrentSkipListSet;
public class PropertyCollection {
private final ConcurrentSkipListSet propertiesList = new ConcurrentSkipListSet<>();
private final Map>> propertiesByNameAndKey = new HashMap<>();
public Iterable getProperties() {
return propertiesList;
}
public synchronized Iterable getProperties(String key, String name) {
if (key == null) {
return getProperties(name);
}
Map> propertiesByKey = propertiesByNameAndKey.get(name);
if (propertiesByKey == null) {
return new ArrayList<>();
}
ConcurrentSkipListSet properties = propertiesByKey.get(key);
if (properties == null) {
return new ArrayList<>();
}
return properties;
}
public synchronized Iterable getProperties(String name) {
Map> propertiesByKey = propertiesByNameAndKey.get(name);
if (propertiesByKey == null) {
return new ArrayList<>();
}
List results = new ArrayList<>();
for (ConcurrentSkipListSet properties : propertiesByKey.values()) {
results.addAll(properties);
}
return results;
}
public synchronized Property getProperty(String name, int index) {
Map> propertiesByKey = propertiesByNameAndKey.get(name);
if (propertiesByKey == null) {
return null;
}
for (ConcurrentSkipListSet properties : propertiesByKey.values()) {
for (Property property : properties) {
if (index == 0) {
return property;
}
index--;
}
}
return null;
}
public synchronized Property getProperty(String key, String name, int index) {
if (key == null) {
return getProperty(name, index);
}
Map> propertiesByKey = propertiesByNameAndKey.get(name);
if (propertiesByKey == null) {
return null;
}
ConcurrentSkipListSet properties = propertiesByKey.get(key);
if (properties == null) {
return null;
}
for (Property property : properties) {
if (index == 0) {
return property;
}
index--;
}
return null;
}
public synchronized void addProperty(Property property) {
ConcurrentSkipListMap> propertiesByKey = propertiesByNameAndKey.get(property.getName());
if (propertiesByKey == null) {
propertiesByKey = new ConcurrentSkipListMap<>();
this.propertiesByNameAndKey.put(property.getName(), propertiesByKey);
}
ConcurrentSkipListSet properties = propertiesByKey.get(property.getKey());
if (properties == null) {
properties = new ConcurrentSkipListSet<>();
propertiesByKey.put(property.getKey(), properties);
}
properties.add(property);
this.propertiesList.add(property);
}
public synchronized void removeProperty(Property property) {
Map> propertiesByKey = propertiesByNameAndKey.get(property.getName());
if (propertiesByKey == null) {
return;
}
ConcurrentSkipListSet properties = propertiesByKey.get(property.getKey());
if (properties == null) {
return;
}
properties.remove(property);
this.propertiesList.remove(property);
}
public synchronized Iterable removeProperties(String name) {
List removedProperties = new ArrayList<>();
Map> propertiesByKey = propertiesByNameAndKey.get(name);
if (propertiesByKey != null) {
for (ConcurrentSkipListSet properties : propertiesByKey.values()) {
for (Property property : properties) {
removedProperties.add(property);
}
}
}
for (Property property : removedProperties) {
removeProperty(property);
}
return removedProperties;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy