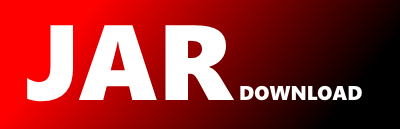
org.vertexium.inmemory.InMemoryTable Maven / Gradle / Ivy
package org.vertexium.inmemory;
import org.vertexium.Authorizations;
import org.vertexium.FetchHint;
import org.vertexium.inmemory.mutations.Mutation;
import org.vertexium.util.LookAheadIterable;
import java.util.EnumSet;
import java.util.Iterator;
import java.util.Map;
import java.util.concurrent.ConcurrentSkipListMap;
public abstract class InMemoryTable {
private Map> rows;
protected InMemoryTable(Map> rows) {
this.rows = rows;
}
protected InMemoryTable() {
this(new ConcurrentSkipListMap>());
}
public TElement get(InMemoryGraph graph, String id, Authorizations authorizations) {
InMemoryTableElement inMemoryTableElement = getTableElement(id);
if (inMemoryTableElement == null) {
return null;
}
return inMemoryTableElement.createElement(graph, authorizations);
}
public InMemoryTableElement getTableElement(String id) {
return rows.get(id);
}
public synchronized void append(String id, Mutation... newMutations) {
InMemoryTableElement inMemoryTableElement = rows.get(id);
if (inMemoryTableElement == null) {
inMemoryTableElement = createInMemoryTableElement(id);
rows.put(id, inMemoryTableElement);
}
inMemoryTableElement.addAll(newMutations);
}
protected abstract InMemoryTableElement createInMemoryTableElement(String id);
public void remove(String id) {
rows.remove(id);
}
public void clear() {
rows.clear();
}
public Iterable getAll(final InMemoryGraph graph, final EnumSet fetchHints, final Long endTime,
final Authorizations authorizations) {
final boolean includeHidden = fetchHints.contains(FetchHint.INCLUDE_HIDDEN);
return new LookAheadIterable, TElement>() {
@Override
protected boolean isIncluded(InMemoryTableElement src, TElement element) {
return graph.isIncludedInTimeSpan(src, fetchHints, endTime, authorizations);
}
@Override
protected TElement convert(InMemoryTableElement element) {
return element.createElement(graph, includeHidden, endTime, authorizations);
}
@Override
protected Iterator> createIterator() {
return rows.values().iterator();
}
};
}
public Iterable> getRowValues() {
return this.rows.values();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy