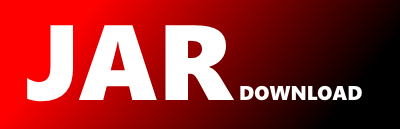
org.vesalainen.parsers.nmea.GPSClock Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of NMEAParser Show documentation
Show all versions of NMEAParser Show documentation
NMEA Parser parses NMEA (including AIS) messages.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy