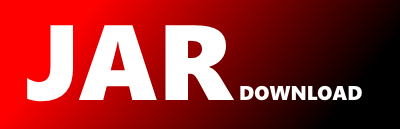
VAqua.src.org.violetlib.aqua.AquaAppearance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vaqua Show documentation
Show all versions of vaqua Show documentation
An improved native Swing look and feel for macOS
The newest version!
/*
* Copyright (c) 2018-2021 Alan Snyder.
* All rights reserved.
*
* You may not use, copy or modify this file, except in compliance with the license agreement. For details see
* accompanying license terms.
*/
package org.violetlib.aqua;
import java.awt.*;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.violetlib.vappearances.VAppearance;
/**
* An object representing a specific appearance, including the current accent and highlight colors.
*/
public class AquaAppearance extends BasicAquaAppearance {
public AquaAppearance(@NotNull VAppearance appearance,
@NotNull Colors colors,
@NotNull Logger log) {
super(appearance, colors, log);
}
/**
* Return the color with the specified name.
* @param colorName The color name.
* @return the color, as a ColorUIResource, or null if the color name not defined in this appearance.
*/
public @Nullable Color getColor(@NotNull String colorName) {
Color color = super.getColor(colorName);
if (AquaColors.isDebugging()) {
Utils.logDebug(" Color " + colorName + ": " + AquaColors.toString(color));
}
return color;
}
/**
* Return a color modified for a specified effect. If no color is defined for that effect, the basic color is
* returned.
* @param colorName The color name.
* @param effectName The effect name.
* @return the color as defined by the color name and effect, or the basic color if there is no variable color
* defined for the specified effect, or null if the color name is note defined in this appearance.
*/
public @Nullable Color getColorForEffect(@NotNull String colorName, @NotNull EffectName effectName) {
if (effectName == EffectName.EFFECT_NONE) {
return getColor(colorName);
}
String extendedName = colorName + "_" + effectName;
Color c = getColor(extendedName);
if (c != null) {
return c;
}
return getColor(colorName);
}
/**
* Return a color modified for a specified effect. If no color is defined for that effect, the basic color is
* returned.
* @param colorName The color name.
* @param effectName The effect name.
* @return the color as defined by the color name and effect, or the basic color if there is no variable color
* defined for the specified effect, or null if the color name is note defined in this appearance.
*/
public @Nullable Color getColorForOptionalEffect(@NotNull String colorName, @NotNull EffectName effectName) {
if (effectName == EffectName.EFFECT_NONE) {
return getColor(colorName);
}
String extendedName = colorName + "_" + effectName;
Color c = getColor(extendedName);
return c != null ? c : getColor(colorName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy