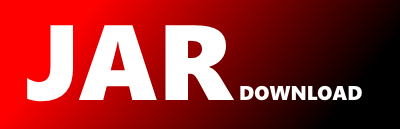
src.bigquery.v2.Table.scala Maven / Gradle / Ivy
package besom.api.googlenative.bigquery.v2
final case class Table private(
urn: besom.types.Output[besom.types.URN],
id: besom.types.Output[besom.types.ResourceId],
biglakeConfiguration: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.BigLakeConfigurationResponse],
cloneDefinition: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.CloneDefinitionResponse],
clustering: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.ClusteringResponse],
creationTime: besom.types.Output[String],
datasetId: besom.types.Output[String],
defaultCollation: besom.types.Output[String],
defaultRoundingMode: besom.types.Output[String],
description: besom.types.Output[String],
encryptionConfiguration: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.EncryptionConfigurationResponse],
etag: besom.types.Output[String],
expirationTime: besom.types.Output[String],
externalDataConfiguration: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.ExternalDataConfigurationResponse],
friendlyName: besom.types.Output[String],
kind: besom.types.Output[String],
labels: besom.types.Output[scala.Predef.Map[String, String]],
lastModifiedTime: besom.types.Output[String],
location: besom.types.Output[String],
materializedView: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.MaterializedViewDefinitionResponse],
maxStaleness: besom.types.Output[String],
model: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.ModelDefinitionResponse],
numActiveLogicalBytes: besom.types.Output[String],
numActivePhysicalBytes: besom.types.Output[String],
numBytes: besom.types.Output[String],
numLongTermBytes: besom.types.Output[String],
numLongTermLogicalBytes: besom.types.Output[String],
numLongTermPhysicalBytes: besom.types.Output[String],
numPartitions: besom.types.Output[String],
numPhysicalBytes: besom.types.Output[String],
numRows: besom.types.Output[String],
numTimeTravelPhysicalBytes: besom.types.Output[String],
numTotalLogicalBytes: besom.types.Output[String],
numTotalPhysicalBytes: besom.types.Output[String],
project: besom.types.Output[String],
rangePartitioning: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.RangePartitioningResponse],
requirePartitionFilter: besom.types.Output[Boolean],
resourceTags: besom.types.Output[scala.Predef.Map[String, String]],
schema: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.TableSchemaResponse],
selfLink: besom.types.Output[String],
snapshotDefinition: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.SnapshotDefinitionResponse],
streamingBuffer: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.StreamingbufferResponse],
tableConstraints: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.TableConstraintsResponse],
tableReference: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.TableReferenceResponse],
timePartitioning: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.TimePartitioningResponse],
`type`: besom.types.Output[String],
view: besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.ViewDefinitionResponse]
) extends besom.CustomResource
object Table extends besom.ResourceCompanion[Table]:
/** Resource constructor for Table.
*
* @param name [[besom.util.NonEmptyString]] The unique (stack-wise) name of the resource in Pulumi state (not on provider's side).
* NonEmptyString is inferred automatically from non-empty string literals, even when interpolated. If you encounter any
* issues with this, please try using `: NonEmptyString` type annotation. If you need to convert a dynamically generated
* string to NonEmptyString, use `NonEmptyString.apply` method - `NonEmptyString(str): Option[NonEmptyString]`.
*
* @param args [[TableArgs]] The configuration to use to create this resource.
*
* @param opts [[besom.CustomResourceOptions]] Resource options to use for this resource.
* Defaults to empty options. If you need to set some options, use [[besom.opts]] function to create them, for example:
*
* {{{
* val res = Table(
* "my-resource",
* TableArgs(...), // your args
* opts(provider = myProvider)
* )
* }}}
*/
def apply(using ctx: besom.types.Context)(
name: besom.util.NonEmptyString,
args: TableArgs,
opts: besom.ResourceOptsVariant.Custom ?=> besom.CustomResourceOptions = besom.CustomResourceOptions()
): besom.types.Output[Table] =
ctx.readOrRegisterResource[Table, TableArgs]("google-native:bigquery/v2:Table", name, args, opts(using besom.ResourceOptsVariant.Custom))
private[besom] def typeToken: besom.types.ResourceType = "google-native:bigquery/v2:Table"
given resourceDecoder(using besom.types.Context): besom.types.ResourceDecoder[Table] =
besom.internal.ResourceDecoder.derived[Table]
given decoder(using besom.types.Context): besom.types.Decoder[Table] =
besom.internal.Decoder.customResourceDecoder[Table]
given outputOps: {} with
extension(output: besom.types.Output[Table])
def urn : besom.types.Output[besom.types.URN] = output.flatMap(_.urn)
def id : besom.types.Output[besom.types.ResourceId] = output.flatMap(_.id)
def biglakeConfiguration : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.BigLakeConfigurationResponse] = output.flatMap(_.biglakeConfiguration)
def cloneDefinition : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.CloneDefinitionResponse] = output.flatMap(_.cloneDefinition)
def clustering : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.ClusteringResponse] = output.flatMap(_.clustering)
def creationTime : besom.types.Output[String] = output.flatMap(_.creationTime)
def datasetId : besom.types.Output[String] = output.flatMap(_.datasetId)
def defaultCollation : besom.types.Output[String] = output.flatMap(_.defaultCollation)
def defaultRoundingMode : besom.types.Output[String] = output.flatMap(_.defaultRoundingMode)
def description : besom.types.Output[String] = output.flatMap(_.description)
def encryptionConfiguration : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.EncryptionConfigurationResponse] = output.flatMap(_.encryptionConfiguration)
def etag : besom.types.Output[String] = output.flatMap(_.etag)
def expirationTime : besom.types.Output[String] = output.flatMap(_.expirationTime)
def externalDataConfiguration : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.ExternalDataConfigurationResponse] = output.flatMap(_.externalDataConfiguration)
def friendlyName : besom.types.Output[String] = output.flatMap(_.friendlyName)
def kind : besom.types.Output[String] = output.flatMap(_.kind)
def labels : besom.types.Output[scala.Predef.Map[String, String]] = output.flatMap(_.labels)
def lastModifiedTime : besom.types.Output[String] = output.flatMap(_.lastModifiedTime)
def location : besom.types.Output[String] = output.flatMap(_.location)
def materializedView : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.MaterializedViewDefinitionResponse] = output.flatMap(_.materializedView)
def maxStaleness : besom.types.Output[String] = output.flatMap(_.maxStaleness)
def model : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.ModelDefinitionResponse] = output.flatMap(_.model)
def numActiveLogicalBytes : besom.types.Output[String] = output.flatMap(_.numActiveLogicalBytes)
def numActivePhysicalBytes : besom.types.Output[String] = output.flatMap(_.numActivePhysicalBytes)
def numBytes : besom.types.Output[String] = output.flatMap(_.numBytes)
def numLongTermBytes : besom.types.Output[String] = output.flatMap(_.numLongTermBytes)
def numLongTermLogicalBytes : besom.types.Output[String] = output.flatMap(_.numLongTermLogicalBytes)
def numLongTermPhysicalBytes : besom.types.Output[String] = output.flatMap(_.numLongTermPhysicalBytes)
def numPartitions : besom.types.Output[String] = output.flatMap(_.numPartitions)
def numPhysicalBytes : besom.types.Output[String] = output.flatMap(_.numPhysicalBytes)
def numRows : besom.types.Output[String] = output.flatMap(_.numRows)
def numTimeTravelPhysicalBytes : besom.types.Output[String] = output.flatMap(_.numTimeTravelPhysicalBytes)
def numTotalLogicalBytes : besom.types.Output[String] = output.flatMap(_.numTotalLogicalBytes)
def numTotalPhysicalBytes : besom.types.Output[String] = output.flatMap(_.numTotalPhysicalBytes)
def project : besom.types.Output[String] = output.flatMap(_.project)
def rangePartitioning : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.RangePartitioningResponse] = output.flatMap(_.rangePartitioning)
def requirePartitionFilter : besom.types.Output[Boolean] = output.flatMap(_.requirePartitionFilter)
def resourceTags : besom.types.Output[scala.Predef.Map[String, String]] = output.flatMap(_.resourceTags)
def schema : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.TableSchemaResponse] = output.flatMap(_.schema)
def selfLink : besom.types.Output[String] = output.flatMap(_.selfLink)
def snapshotDefinition : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.SnapshotDefinitionResponse] = output.flatMap(_.snapshotDefinition)
def streamingBuffer : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.StreamingbufferResponse] = output.flatMap(_.streamingBuffer)
def tableConstraints : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.TableConstraintsResponse] = output.flatMap(_.tableConstraints)
def tableReference : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.TableReferenceResponse] = output.flatMap(_.tableReference)
def timePartitioning : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.TimePartitioningResponse] = output.flatMap(_.timePartitioning)
def `type` : besom.types.Output[String] = output.flatMap(_.`type`)
def view : besom.types.Output[besom.api.googlenative.bigquery.v2.outputs.ViewDefinitionResponse] = output.flatMap(_.view)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy