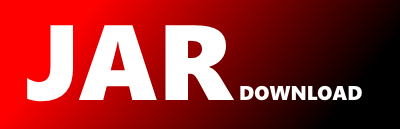
src.cloudbuild.v1.TriggerArgs.scala Maven / Gradle / Ivy
package besom.api.googlenative.cloudbuild.v1
final case class TriggerArgs private(
approvalConfig: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.ApprovalConfigArgs]],
autodetect: besom.types.Output[scala.Option[Boolean]],
bitbucketServerTriggerConfig: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.BitbucketServerTriggerConfigArgs]],
build: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.BuildArgs]],
description: besom.types.Output[scala.Option[String]],
disabled: besom.types.Output[scala.Option[Boolean]],
eventType: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.enums.TriggerEventType]],
filename: besom.types.Output[scala.Option[String]],
filter: besom.types.Output[scala.Option[String]],
gitFileSource: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.GitFileSourceArgs]],
github: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.GitHubEventsConfigArgs]],
gitlabEnterpriseEventsConfig: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.GitLabEventsConfigArgs]],
ignoredFiles: besom.types.Output[scala.Option[scala.collection.immutable.List[String]]],
includeBuildLogs: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.enums.TriggerIncludeBuildLogs]],
includedFiles: besom.types.Output[scala.Option[scala.collection.immutable.List[String]]],
location: besom.types.Output[scala.Option[String]],
name: besom.types.Output[scala.Option[String]],
project: besom.types.Output[scala.Option[String]],
projectId: besom.types.Output[String],
pubsubConfig: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.PubsubConfigArgs]],
repositoryEventConfig: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.RepositoryEventConfigArgs]],
resourceName: besom.types.Output[scala.Option[String]],
serviceAccount: besom.types.Output[scala.Option[String]],
sourceToBuild: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.GitRepoSourceArgs]],
substitutions: besom.types.Output[scala.Option[scala.Predef.Map[String, String]]],
tags: besom.types.Output[scala.Option[scala.collection.immutable.List[String]]],
triggerTemplate: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.RepoSourceArgs]],
webhookConfig: besom.types.Output[scala.Option[besom.api.googlenative.cloudbuild.v1.inputs.WebhookConfigArgs]]
)
object TriggerArgs:
def apply(
approvalConfig: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.ApprovalConfigArgs] = scala.None,
autodetect: besom.types.Input.Optional[Boolean] = scala.None,
bitbucketServerTriggerConfig: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.BitbucketServerTriggerConfigArgs] = scala.None,
build: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.BuildArgs] = scala.None,
description: besom.types.Input.Optional[String] = scala.None,
disabled: besom.types.Input.Optional[Boolean] = scala.None,
eventType: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.enums.TriggerEventType] = scala.None,
filename: besom.types.Input.Optional[String] = scala.None,
filter: besom.types.Input.Optional[String] = scala.None,
gitFileSource: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.GitFileSourceArgs] = scala.None,
github: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.GitHubEventsConfigArgs] = scala.None,
gitlabEnterpriseEventsConfig: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.GitLabEventsConfigArgs] = scala.None,
ignoredFiles: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[String]]] = scala.None,
includeBuildLogs: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.enums.TriggerIncludeBuildLogs] = scala.None,
includedFiles: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[String]]] = scala.None,
location: besom.types.Input.Optional[String] = scala.None,
name: besom.types.Input.Optional[String] = scala.None,
project: besom.types.Input.Optional[String] = scala.None,
projectId: besom.types.Input[String],
pubsubConfig: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.PubsubConfigArgs] = scala.None,
repositoryEventConfig: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.RepositoryEventConfigArgs] = scala.None,
resourceName: besom.types.Input.Optional[String] = scala.None,
serviceAccount: besom.types.Input.Optional[String] = scala.None,
sourceToBuild: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.GitRepoSourceArgs] = scala.None,
substitutions: besom.types.Input.Optional[scala.Predef.Map[String, besom.types.Input[String]]] = scala.None,
tags: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[String]]] = scala.None,
triggerTemplate: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.RepoSourceArgs] = scala.None,
webhookConfig: besom.types.Input.Optional[besom.api.googlenative.cloudbuild.v1.inputs.WebhookConfigArgs] = scala.None
)(using besom.types.Context): TriggerArgs =
new TriggerArgs(
approvalConfig = approvalConfig.asOptionOutput(isSecret = false),
autodetect = autodetect.asOptionOutput(isSecret = false),
bitbucketServerTriggerConfig = bitbucketServerTriggerConfig.asOptionOutput(isSecret = false),
build = build.asOptionOutput(isSecret = false),
description = description.asOptionOutput(isSecret = false),
disabled = disabled.asOptionOutput(isSecret = false),
eventType = eventType.asOptionOutput(isSecret = false),
filename = filename.asOptionOutput(isSecret = false),
filter = filter.asOptionOutput(isSecret = false),
gitFileSource = gitFileSource.asOptionOutput(isSecret = false),
github = github.asOptionOutput(isSecret = false),
gitlabEnterpriseEventsConfig = gitlabEnterpriseEventsConfig.asOptionOutput(isSecret = false),
ignoredFiles = ignoredFiles.asOptionOutput(isSecret = false),
includeBuildLogs = includeBuildLogs.asOptionOutput(isSecret = false),
includedFiles = includedFiles.asOptionOutput(isSecret = false),
location = location.asOptionOutput(isSecret = false),
name = name.asOptionOutput(isSecret = false),
project = project.asOptionOutput(isSecret = false),
projectId = projectId.asOutput(isSecret = false),
pubsubConfig = pubsubConfig.asOptionOutput(isSecret = false),
repositoryEventConfig = repositoryEventConfig.asOptionOutput(isSecret = false),
resourceName = resourceName.asOptionOutput(isSecret = false),
serviceAccount = serviceAccount.asOptionOutput(isSecret = false),
sourceToBuild = sourceToBuild.asOptionOutput(isSecret = false),
substitutions = substitutions.asOptionOutput(isSecret = false),
tags = tags.asOptionOutput(isSecret = false),
triggerTemplate = triggerTemplate.asOptionOutput(isSecret = false),
webhookConfig = webhookConfig.asOptionOutput(isSecret = false)
)
given encoder(using besom.types.Context): besom.types.Encoder[TriggerArgs] =
besom.internal.Encoder.derived[TriggerArgs]
given argsEncoder(using besom.types.Context): besom.types.ArgsEncoder[TriggerArgs] =
besom.internal.ArgsEncoder.derived[TriggerArgs]
© 2015 - 2025 Weber Informatics LLC | Privacy Policy