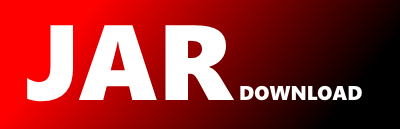
src.compute.alpha.RegionBackendService.scala Maven / Gradle / Ivy
The newest version!
package besom.api.googlenative.compute.alpha
final case class RegionBackendService private(
urn: besom.types.Output[besom.types.URN],
id: besom.types.Output[besom.types.ResourceId],
affinityCookieTtlSec: besom.types.Output[Int],
backends: besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.compute.alpha.outputs.BackendResponse]],
cdnPolicy: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceCdnPolicyResponse],
circuitBreakers: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.CircuitBreakersResponse],
compressionMode: besom.types.Output[String],
connectionDraining: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.ConnectionDrainingResponse],
connectionTrackingPolicy: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceConnectionTrackingPolicyResponse],
consistentHash: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.ConsistentHashLoadBalancerSettingsResponse],
creationTimestamp: besom.types.Output[String],
customRequestHeaders: besom.types.Output[scala.collection.immutable.List[String]],
customResponseHeaders: besom.types.Output[scala.collection.immutable.List[String]],
description: besom.types.Output[String],
edgeSecurityPolicy: besom.types.Output[String],
enableCDN: besom.types.Output[Boolean],
failoverPolicy: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceFailoverPolicyResponse],
fingerprint: besom.types.Output[String],
healthChecks: besom.types.Output[scala.collection.immutable.List[String]],
iap: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceIapResponse],
ipAddressSelectionPolicy: besom.types.Output[String],
kind: besom.types.Output[String],
loadBalancingScheme: besom.types.Output[String],
localityLbPolicies: besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.compute.alpha.outputs.BackendServiceLocalityLoadBalancingPolicyConfigResponse]],
localityLbPolicy: besom.types.Output[String],
logConfig: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceLogConfigResponse],
maxStreamDuration: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.DurationResponse],
metadatas: besom.types.Output[scala.Predef.Map[String, String]],
name: besom.types.Output[String],
network: besom.types.Output[String],
outlierDetection: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.OutlierDetectionResponse],
port: besom.types.Output[Int],
portName: besom.types.Output[String],
project: besom.types.Output[String],
protocol: besom.types.Output[String],
region: besom.types.Output[String],
requestId: besom.types.Output[scala.Option[String]],
securityPolicy: besom.types.Output[String],
securitySettings: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.SecuritySettingsResponse],
selfLink: besom.types.Output[String],
selfLinkWithId: besom.types.Output[String],
serviceBindings: besom.types.Output[scala.collection.immutable.List[String]],
serviceLbPolicy: besom.types.Output[String],
sessionAffinity: besom.types.Output[String],
subsetting: besom.types.Output[besom.api.googlenative.compute.alpha.outputs.SubsettingResponse],
timeoutSec: besom.types.Output[Int],
usedBy: besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.compute.alpha.outputs.BackendServiceUsedByResponse]],
vpcNetworkScope: besom.types.Output[String]
) extends besom.CustomResource
object RegionBackendService extends besom.ResourceCompanion[RegionBackendService]:
/** Resource constructor for RegionBackendService.
*
* @param name [[besom.util.NonEmptyString]] The unique (stack-wise) name of the resource in Pulumi state (not on provider's side).
* NonEmptyString is inferred automatically from non-empty string literals, even when interpolated. If you encounter any
* issues with this, please try using `: NonEmptyString` type annotation. If you need to convert a dynamically generated
* string to NonEmptyString, use `NonEmptyString.apply` method - `NonEmptyString(str): Option[NonEmptyString]`.
*
* @param args [[RegionBackendServiceArgs]] The configuration to use to create this resource.
*
* @param opts [[besom.CustomResourceOptions]] Resource options to use for this resource.
* Defaults to empty options. If you need to set some options, use [[besom.opts]] function to create them, for example:
*
* {{{
* val res = RegionBackendService(
* "my-resource",
* RegionBackendServiceArgs(...), // your args
* opts(provider = myProvider)
* )
* }}}
*/
def apply(using ctx: besom.types.Context)(
name: besom.util.NonEmptyString,
args: RegionBackendServiceArgs,
opts: besom.ResourceOptsVariant.Custom ?=> besom.CustomResourceOptions = besom.CustomResourceOptions()
): besom.types.Output[RegionBackendService] =
ctx.readOrRegisterResource[RegionBackendService, RegionBackendServiceArgs]("google-native:compute/alpha:RegionBackendService", name, args, opts(using besom.ResourceOptsVariant.Custom))
private[besom] def typeToken: besom.types.ResourceType = "google-native:compute/alpha:RegionBackendService"
given resourceDecoder(using besom.types.Context): besom.types.ResourceDecoder[RegionBackendService] =
besom.internal.ResourceDecoder.derived[RegionBackendService]
given decoder(using besom.types.Context): besom.types.Decoder[RegionBackendService] =
besom.internal.Decoder.customResourceDecoder[RegionBackendService]
given outputOps: {} with
extension(output: besom.types.Output[RegionBackendService])
def urn : besom.types.Output[besom.types.URN] = output.flatMap(_.urn)
def id : besom.types.Output[besom.types.ResourceId] = output.flatMap(_.id)
def affinityCookieTtlSec : besom.types.Output[Int] = output.flatMap(_.affinityCookieTtlSec)
def backends : besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.compute.alpha.outputs.BackendResponse]] = output.flatMap(_.backends)
def cdnPolicy : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceCdnPolicyResponse] = output.flatMap(_.cdnPolicy)
def circuitBreakers : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.CircuitBreakersResponse] = output.flatMap(_.circuitBreakers)
def compressionMode : besom.types.Output[String] = output.flatMap(_.compressionMode)
def connectionDraining : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.ConnectionDrainingResponse] = output.flatMap(_.connectionDraining)
def connectionTrackingPolicy : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceConnectionTrackingPolicyResponse] = output.flatMap(_.connectionTrackingPolicy)
def consistentHash : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.ConsistentHashLoadBalancerSettingsResponse] = output.flatMap(_.consistentHash)
def creationTimestamp : besom.types.Output[String] = output.flatMap(_.creationTimestamp)
def customRequestHeaders : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.customRequestHeaders)
def customResponseHeaders : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.customResponseHeaders)
def description : besom.types.Output[String] = output.flatMap(_.description)
def edgeSecurityPolicy : besom.types.Output[String] = output.flatMap(_.edgeSecurityPolicy)
def enableCDN : besom.types.Output[Boolean] = output.flatMap(_.enableCDN)
def failoverPolicy : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceFailoverPolicyResponse] = output.flatMap(_.failoverPolicy)
def fingerprint : besom.types.Output[String] = output.flatMap(_.fingerprint)
def healthChecks : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.healthChecks)
def iap : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceIapResponse] = output.flatMap(_.iap)
def ipAddressSelectionPolicy : besom.types.Output[String] = output.flatMap(_.ipAddressSelectionPolicy)
def kind : besom.types.Output[String] = output.flatMap(_.kind)
def loadBalancingScheme : besom.types.Output[String] = output.flatMap(_.loadBalancingScheme)
def localityLbPolicies : besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.compute.alpha.outputs.BackendServiceLocalityLoadBalancingPolicyConfigResponse]] = output.flatMap(_.localityLbPolicies)
def localityLbPolicy : besom.types.Output[String] = output.flatMap(_.localityLbPolicy)
def logConfig : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.BackendServiceLogConfigResponse] = output.flatMap(_.logConfig)
def maxStreamDuration : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.DurationResponse] = output.flatMap(_.maxStreamDuration)
def metadatas : besom.types.Output[scala.Predef.Map[String, String]] = output.flatMap(_.metadatas)
def name : besom.types.Output[String] = output.flatMap(_.name)
def network : besom.types.Output[String] = output.flatMap(_.network)
def outlierDetection : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.OutlierDetectionResponse] = output.flatMap(_.outlierDetection)
def port : besom.types.Output[Int] = output.flatMap(_.port)
def portName : besom.types.Output[String] = output.flatMap(_.portName)
def project : besom.types.Output[String] = output.flatMap(_.project)
def protocol : besom.types.Output[String] = output.flatMap(_.protocol)
def region : besom.types.Output[String] = output.flatMap(_.region)
def requestId : besom.types.Output[scala.Option[String]] = output.flatMap(_.requestId)
def securityPolicy : besom.types.Output[String] = output.flatMap(_.securityPolicy)
def securitySettings : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.SecuritySettingsResponse] = output.flatMap(_.securitySettings)
def selfLink : besom.types.Output[String] = output.flatMap(_.selfLink)
def selfLinkWithId : besom.types.Output[String] = output.flatMap(_.selfLinkWithId)
def serviceBindings : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.serviceBindings)
def serviceLbPolicy : besom.types.Output[String] = output.flatMap(_.serviceLbPolicy)
def sessionAffinity : besom.types.Output[String] = output.flatMap(_.sessionAffinity)
def subsetting : besom.types.Output[besom.api.googlenative.compute.alpha.outputs.SubsettingResponse] = output.flatMap(_.subsetting)
def timeoutSec : besom.types.Output[Int] = output.flatMap(_.timeoutSec)
def usedBy : besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.compute.alpha.outputs.BackendServiceUsedByResponse]] = output.flatMap(_.usedBy)
def vpcNetworkScope : besom.types.Output[String] = output.flatMap(_.vpcNetworkScope)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy