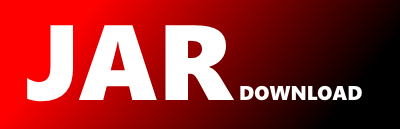
src.compute.beta.Disk.scala Maven / Gradle / Ivy
The newest version!
package besom.api.googlenative.compute.beta
final case class Disk private(
urn: besom.types.Output[besom.types.URN],
id: besom.types.Output[besom.types.ResourceId],
architecture: besom.types.Output[String],
asyncPrimaryDisk: besom.types.Output[besom.api.googlenative.compute.beta.outputs.DiskAsyncReplicationResponse],
asyncSecondaryDisks: besom.types.Output[scala.Predef.Map[String, String]],
creationTimestamp: besom.types.Output[String],
description: besom.types.Output[String],
diskEncryptionKey: besom.types.Output[besom.api.googlenative.compute.beta.outputs.CustomerEncryptionKeyResponse],
enableConfidentialCompute: besom.types.Output[Boolean],
eraseWindowsVssSignature: besom.types.Output[Boolean],
guestOsFeatures: besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.compute.beta.outputs.GuestOsFeatureResponse]],
interface: besom.types.Output[String],
kind: besom.types.Output[String],
labelFingerprint: besom.types.Output[String],
labels: besom.types.Output[scala.Predef.Map[String, String]],
lastAttachTimestamp: besom.types.Output[String],
lastDetachTimestamp: besom.types.Output[String],
licenseCodes: besom.types.Output[scala.collection.immutable.List[String]],
licenses: besom.types.Output[scala.collection.immutable.List[String]],
locationHint: besom.types.Output[String],
locked: besom.types.Output[Boolean],
multiWriter: besom.types.Output[Boolean],
name: besom.types.Output[String],
options: besom.types.Output[String],
params: besom.types.Output[besom.api.googlenative.compute.beta.outputs.DiskParamsResponse],
physicalBlockSizeBytes: besom.types.Output[String],
project: besom.types.Output[String],
provisionedIops: besom.types.Output[String],
provisionedThroughput: besom.types.Output[String],
region: besom.types.Output[String],
replicaZones: besom.types.Output[scala.collection.immutable.List[String]],
requestId: besom.types.Output[scala.Option[String]],
resourcePolicies: besom.types.Output[scala.collection.immutable.List[String]],
resourceStatus: besom.types.Output[besom.api.googlenative.compute.beta.outputs.DiskResourceStatusResponse],
satisfiesPzs: besom.types.Output[Boolean],
selfLink: besom.types.Output[String],
sizeGb: besom.types.Output[String],
sourceConsistencyGroupPolicy: besom.types.Output[String],
sourceConsistencyGroupPolicyId: besom.types.Output[String],
sourceDisk: besom.types.Output[String],
sourceDiskId: besom.types.Output[String],
sourceImage: besom.types.Output[String],
sourceImageEncryptionKey: besom.types.Output[besom.api.googlenative.compute.beta.outputs.CustomerEncryptionKeyResponse],
sourceImageId: besom.types.Output[String],
sourceInstantSnapshot: besom.types.Output[String],
sourceInstantSnapshotId: besom.types.Output[String],
sourceSnapshot: besom.types.Output[String],
sourceSnapshotEncryptionKey: besom.types.Output[besom.api.googlenative.compute.beta.outputs.CustomerEncryptionKeyResponse],
sourceSnapshotId: besom.types.Output[String],
sourceStorageObject: besom.types.Output[String],
status: besom.types.Output[String],
storageType: besom.types.Output[String],
`type`: besom.types.Output[String],
userLicenses: besom.types.Output[scala.collection.immutable.List[String]],
users: besom.types.Output[scala.collection.immutable.List[String]],
zone: besom.types.Output[String]
) extends besom.CustomResource
object Disk extends besom.ResourceCompanion[Disk]:
/** Resource constructor for Disk.
*
* @param name [[besom.util.NonEmptyString]] The unique (stack-wise) name of the resource in Pulumi state (not on provider's side).
* NonEmptyString is inferred automatically from non-empty string literals, even when interpolated. If you encounter any
* issues with this, please try using `: NonEmptyString` type annotation. If you need to convert a dynamically generated
* string to NonEmptyString, use `NonEmptyString.apply` method - `NonEmptyString(str): Option[NonEmptyString]`.
*
* @param args [[DiskArgs]] The configuration to use to create this resource. This resource has a default configuration.
*
* @param opts [[besom.CustomResourceOptions]] Resource options to use for this resource.
* Defaults to empty options. If you need to set some options, use [[besom.opts]] function to create them, for example:
*
* {{{
* val res = Disk(
* "my-resource",
* DiskArgs(...), // your args
* opts(provider = myProvider)
* )
* }}}
*/
def apply(using ctx: besom.types.Context)(
name: besom.util.NonEmptyString,
args: DiskArgs = DiskArgs(),
opts: besom.ResourceOptsVariant.Custom ?=> besom.CustomResourceOptions = besom.CustomResourceOptions()
): besom.types.Output[Disk] =
ctx.readOrRegisterResource[Disk, DiskArgs]("google-native:compute/beta:Disk", name, args, opts(using besom.ResourceOptsVariant.Custom))
private[besom] def typeToken: besom.types.ResourceType = "google-native:compute/beta:Disk"
given resourceDecoder(using besom.types.Context): besom.types.ResourceDecoder[Disk] =
besom.internal.ResourceDecoder.derived[Disk]
given decoder(using besom.types.Context): besom.types.Decoder[Disk] =
besom.internal.Decoder.customResourceDecoder[Disk]
given outputOps: {} with
extension(output: besom.types.Output[Disk])
def urn : besom.types.Output[besom.types.URN] = output.flatMap(_.urn)
def id : besom.types.Output[besom.types.ResourceId] = output.flatMap(_.id)
def architecture : besom.types.Output[String] = output.flatMap(_.architecture)
def asyncPrimaryDisk : besom.types.Output[besom.api.googlenative.compute.beta.outputs.DiskAsyncReplicationResponse] = output.flatMap(_.asyncPrimaryDisk)
def asyncSecondaryDisks : besom.types.Output[scala.Predef.Map[String, String]] = output.flatMap(_.asyncSecondaryDisks)
def creationTimestamp : besom.types.Output[String] = output.flatMap(_.creationTimestamp)
def description : besom.types.Output[String] = output.flatMap(_.description)
def diskEncryptionKey : besom.types.Output[besom.api.googlenative.compute.beta.outputs.CustomerEncryptionKeyResponse] = output.flatMap(_.diskEncryptionKey)
def enableConfidentialCompute : besom.types.Output[Boolean] = output.flatMap(_.enableConfidentialCompute)
def eraseWindowsVssSignature : besom.types.Output[Boolean] = output.flatMap(_.eraseWindowsVssSignature)
def guestOsFeatures : besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.compute.beta.outputs.GuestOsFeatureResponse]] = output.flatMap(_.guestOsFeatures)
def interface : besom.types.Output[String] = output.flatMap(_.interface)
def kind : besom.types.Output[String] = output.flatMap(_.kind)
def labelFingerprint : besom.types.Output[String] = output.flatMap(_.labelFingerprint)
def labels : besom.types.Output[scala.Predef.Map[String, String]] = output.flatMap(_.labels)
def lastAttachTimestamp : besom.types.Output[String] = output.flatMap(_.lastAttachTimestamp)
def lastDetachTimestamp : besom.types.Output[String] = output.flatMap(_.lastDetachTimestamp)
def licenseCodes : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.licenseCodes)
def licenses : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.licenses)
def locationHint : besom.types.Output[String] = output.flatMap(_.locationHint)
def locked : besom.types.Output[Boolean] = output.flatMap(_.locked)
def multiWriter : besom.types.Output[Boolean] = output.flatMap(_.multiWriter)
def name : besom.types.Output[String] = output.flatMap(_.name)
def options : besom.types.Output[String] = output.flatMap(_.options)
def params : besom.types.Output[besom.api.googlenative.compute.beta.outputs.DiskParamsResponse] = output.flatMap(_.params)
def physicalBlockSizeBytes : besom.types.Output[String] = output.flatMap(_.physicalBlockSizeBytes)
def project : besom.types.Output[String] = output.flatMap(_.project)
def provisionedIops : besom.types.Output[String] = output.flatMap(_.provisionedIops)
def provisionedThroughput : besom.types.Output[String] = output.flatMap(_.provisionedThroughput)
def region : besom.types.Output[String] = output.flatMap(_.region)
def replicaZones : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.replicaZones)
def requestId : besom.types.Output[scala.Option[String]] = output.flatMap(_.requestId)
def resourcePolicies : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.resourcePolicies)
def resourceStatus : besom.types.Output[besom.api.googlenative.compute.beta.outputs.DiskResourceStatusResponse] = output.flatMap(_.resourceStatus)
def satisfiesPzs : besom.types.Output[Boolean] = output.flatMap(_.satisfiesPzs)
def selfLink : besom.types.Output[String] = output.flatMap(_.selfLink)
def sizeGb : besom.types.Output[String] = output.flatMap(_.sizeGb)
def sourceConsistencyGroupPolicy : besom.types.Output[String] = output.flatMap(_.sourceConsistencyGroupPolicy)
def sourceConsistencyGroupPolicyId : besom.types.Output[String] = output.flatMap(_.sourceConsistencyGroupPolicyId)
def sourceDisk : besom.types.Output[String] = output.flatMap(_.sourceDisk)
def sourceDiskId : besom.types.Output[String] = output.flatMap(_.sourceDiskId)
def sourceImage : besom.types.Output[String] = output.flatMap(_.sourceImage)
def sourceImageEncryptionKey : besom.types.Output[besom.api.googlenative.compute.beta.outputs.CustomerEncryptionKeyResponse] = output.flatMap(_.sourceImageEncryptionKey)
def sourceImageId : besom.types.Output[String] = output.flatMap(_.sourceImageId)
def sourceInstantSnapshot : besom.types.Output[String] = output.flatMap(_.sourceInstantSnapshot)
def sourceInstantSnapshotId : besom.types.Output[String] = output.flatMap(_.sourceInstantSnapshotId)
def sourceSnapshot : besom.types.Output[String] = output.flatMap(_.sourceSnapshot)
def sourceSnapshotEncryptionKey : besom.types.Output[besom.api.googlenative.compute.beta.outputs.CustomerEncryptionKeyResponse] = output.flatMap(_.sourceSnapshotEncryptionKey)
def sourceSnapshotId : besom.types.Output[String] = output.flatMap(_.sourceSnapshotId)
def sourceStorageObject : besom.types.Output[String] = output.flatMap(_.sourceStorageObject)
def status : besom.types.Output[String] = output.flatMap(_.status)
def storageType : besom.types.Output[String] = output.flatMap(_.storageType)
def `type` : besom.types.Output[String] = output.flatMap(_.`type`)
def userLicenses : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.userLicenses)
def users : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.users)
def zone : besom.types.Output[String] = output.flatMap(_.zone)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy