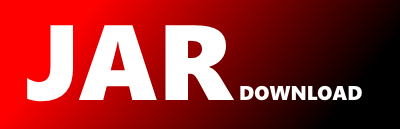
src.dataflow.v1b3.JobArgs.scala Maven / Gradle / Ivy
The newest version!
package besom.api.googlenative.dataflow.v1b3
final case class JobArgs private(
clientRequestId: besom.types.Output[scala.Option[String]],
createTime: besom.types.Output[scala.Option[String]],
createdFromSnapshotId: besom.types.Output[scala.Option[String]],
currentState: besom.types.Output[scala.Option[besom.api.googlenative.dataflow.v1b3.enums.JobCurrentState]],
currentStateTime: besom.types.Output[scala.Option[String]],
environment: besom.types.Output[scala.Option[besom.api.googlenative.dataflow.v1b3.inputs.EnvironmentArgs]],
executionInfo: besom.types.Output[scala.Option[besom.api.googlenative.dataflow.v1b3.inputs.JobExecutionInfoArgs]],
id: besom.types.Output[scala.Option[String]],
jobMetadata: besom.types.Output[scala.Option[besom.api.googlenative.dataflow.v1b3.inputs.JobMetadataArgs]],
labels: besom.types.Output[scala.Option[scala.Predef.Map[String, String]]],
location: besom.types.Output[scala.Option[String]],
name: besom.types.Output[scala.Option[String]],
pipelineDescription: besom.types.Output[scala.Option[besom.api.googlenative.dataflow.v1b3.inputs.PipelineDescriptionArgs]],
project: besom.types.Output[scala.Option[String]],
replaceJobId: besom.types.Output[scala.Option[String]],
replacedByJobId: besom.types.Output[scala.Option[String]],
requestedState: besom.types.Output[scala.Option[besom.api.googlenative.dataflow.v1b3.enums.JobRequestedState]],
runtimeUpdatableParams: besom.types.Output[scala.Option[besom.api.googlenative.dataflow.v1b3.inputs.RuntimeUpdatableParamsArgs]],
satisfiesPzs: besom.types.Output[scala.Option[Boolean]],
stageStates: besom.types.Output[scala.Option[scala.collection.immutable.List[besom.api.googlenative.dataflow.v1b3.inputs.ExecutionStageStateArgs]]],
startTime: besom.types.Output[scala.Option[String]],
steps: besom.types.Output[scala.Option[scala.collection.immutable.List[besom.api.googlenative.dataflow.v1b3.inputs.StepArgs]]],
stepsLocation: besom.types.Output[scala.Option[String]],
tempFiles: besom.types.Output[scala.Option[scala.collection.immutable.List[String]]],
transformNameMapping: besom.types.Output[scala.Option[scala.Predef.Map[String, String]]],
`type`: besom.types.Output[scala.Option[besom.api.googlenative.dataflow.v1b3.enums.JobType]],
view: besom.types.Output[scala.Option[String]]
)
object JobArgs:
def apply(
clientRequestId: besom.types.Input.Optional[String] = scala.None,
createTime: besom.types.Input.Optional[String] = scala.None,
createdFromSnapshotId: besom.types.Input.Optional[String] = scala.None,
currentState: besom.types.Input.Optional[besom.api.googlenative.dataflow.v1b3.enums.JobCurrentState] = scala.None,
currentStateTime: besom.types.Input.Optional[String] = scala.None,
environment: besom.types.Input.Optional[besom.api.googlenative.dataflow.v1b3.inputs.EnvironmentArgs] = scala.None,
executionInfo: besom.types.Input.Optional[besom.api.googlenative.dataflow.v1b3.inputs.JobExecutionInfoArgs] = scala.None,
id: besom.types.Input.Optional[String] = scala.None,
jobMetadata: besom.types.Input.Optional[besom.api.googlenative.dataflow.v1b3.inputs.JobMetadataArgs] = scala.None,
labels: besom.types.Input.Optional[scala.Predef.Map[String, besom.types.Input[String]]] = scala.None,
location: besom.types.Input.Optional[String] = scala.None,
name: besom.types.Input.Optional[String] = scala.None,
pipelineDescription: besom.types.Input.Optional[besom.api.googlenative.dataflow.v1b3.inputs.PipelineDescriptionArgs] = scala.None,
project: besom.types.Input.Optional[String] = scala.None,
replaceJobId: besom.types.Input.Optional[String] = scala.None,
replacedByJobId: besom.types.Input.Optional[String] = scala.None,
requestedState: besom.types.Input.Optional[besom.api.googlenative.dataflow.v1b3.enums.JobRequestedState] = scala.None,
runtimeUpdatableParams: besom.types.Input.Optional[besom.api.googlenative.dataflow.v1b3.inputs.RuntimeUpdatableParamsArgs] = scala.None,
satisfiesPzs: besom.types.Input.Optional[Boolean] = scala.None,
stageStates: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[besom.api.googlenative.dataflow.v1b3.inputs.ExecutionStageStateArgs]]] = scala.None,
startTime: besom.types.Input.Optional[String] = scala.None,
steps: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[besom.api.googlenative.dataflow.v1b3.inputs.StepArgs]]] = scala.None,
stepsLocation: besom.types.Input.Optional[String] = scala.None,
tempFiles: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[String]]] = scala.None,
transformNameMapping: besom.types.Input.Optional[scala.Predef.Map[String, besom.types.Input[String]]] = scala.None,
`type`: besom.types.Input.Optional[besom.api.googlenative.dataflow.v1b3.enums.JobType] = scala.None,
view: besom.types.Input.Optional[String] = scala.None
)(using besom.types.Context): JobArgs =
new JobArgs(
clientRequestId = clientRequestId.asOptionOutput(isSecret = false),
createTime = createTime.asOptionOutput(isSecret = false),
createdFromSnapshotId = createdFromSnapshotId.asOptionOutput(isSecret = false),
currentState = currentState.asOptionOutput(isSecret = false),
currentStateTime = currentStateTime.asOptionOutput(isSecret = false),
environment = environment.asOptionOutput(isSecret = false),
executionInfo = executionInfo.asOptionOutput(isSecret = false),
id = id.asOptionOutput(isSecret = false),
jobMetadata = jobMetadata.asOptionOutput(isSecret = false),
labels = labels.asOptionOutput(isSecret = false),
location = location.asOptionOutput(isSecret = false),
name = name.asOptionOutput(isSecret = false),
pipelineDescription = pipelineDescription.asOptionOutput(isSecret = false),
project = project.asOptionOutput(isSecret = false),
replaceJobId = replaceJobId.asOptionOutput(isSecret = false),
replacedByJobId = replacedByJobId.asOptionOutput(isSecret = false),
requestedState = requestedState.asOptionOutput(isSecret = false),
runtimeUpdatableParams = runtimeUpdatableParams.asOptionOutput(isSecret = false),
satisfiesPzs = satisfiesPzs.asOptionOutput(isSecret = false),
stageStates = stageStates.asOptionOutput(isSecret = false),
startTime = startTime.asOptionOutput(isSecret = false),
steps = steps.asOptionOutput(isSecret = false),
stepsLocation = stepsLocation.asOptionOutput(isSecret = false),
tempFiles = tempFiles.asOptionOutput(isSecret = false),
transformNameMapping = transformNameMapping.asOptionOutput(isSecret = false),
`type` = `type`.asOptionOutput(isSecret = false),
view = view.asOptionOutput(isSecret = false)
)
given encoder(using besom.types.Context): besom.types.Encoder[JobArgs] =
besom.internal.Encoder.derived[JobArgs]
given argsEncoder(using besom.types.Context): besom.types.ArgsEncoder[JobArgs] =
besom.internal.ArgsEncoder.derived[JobArgs]
© 2015 - 2025 Weber Informatics LLC | Privacy Policy