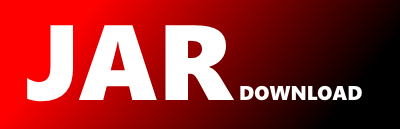
src.jobs.v4.JobArgs.scala Maven / Gradle / Ivy
package besom.api.googlenative.jobs.v4
final case class JobArgs private(
addresses: besom.types.Output[scala.Option[scala.collection.immutable.List[String]]],
applicationInfo: besom.types.Output[scala.Option[besom.api.googlenative.jobs.v4.inputs.ApplicationInfoArgs]],
company: besom.types.Output[String],
compensationInfo: besom.types.Output[scala.Option[besom.api.googlenative.jobs.v4.inputs.CompensationInfoArgs]],
customAttributes: besom.types.Output[scala.Option[scala.Predef.Map[String, String]]],
degreeTypes: besom.types.Output[scala.Option[scala.collection.immutable.List[besom.api.googlenative.jobs.v4.enums.JobDegreeTypesItem]]],
department: besom.types.Output[scala.Option[String]],
description: besom.types.Output[String],
employmentTypes: besom.types.Output[scala.Option[scala.collection.immutable.List[besom.api.googlenative.jobs.v4.enums.JobEmploymentTypesItem]]],
incentives: besom.types.Output[scala.Option[String]],
jobBenefits: besom.types.Output[scala.Option[scala.collection.immutable.List[besom.api.googlenative.jobs.v4.enums.JobJobBenefitsItem]]],
jobEndTime: besom.types.Output[scala.Option[String]],
jobLevel: besom.types.Output[scala.Option[besom.api.googlenative.jobs.v4.enums.JobJobLevel]],
jobStartTime: besom.types.Output[scala.Option[String]],
languageCode: besom.types.Output[scala.Option[String]],
name: besom.types.Output[scala.Option[String]],
postingExpireTime: besom.types.Output[scala.Option[String]],
postingPublishTime: besom.types.Output[scala.Option[String]],
postingRegion: besom.types.Output[scala.Option[besom.api.googlenative.jobs.v4.enums.JobPostingRegion]],
processingOptions: besom.types.Output[scala.Option[besom.api.googlenative.jobs.v4.inputs.ProcessingOptionsArgs]],
project: besom.types.Output[scala.Option[String]],
promotionValue: besom.types.Output[scala.Option[Int]],
qualifications: besom.types.Output[scala.Option[String]],
requisitionId: besom.types.Output[String],
responsibilities: besom.types.Output[scala.Option[String]],
tenantId: besom.types.Output[String],
title: besom.types.Output[String],
visibility: besom.types.Output[scala.Option[besom.api.googlenative.jobs.v4.enums.JobVisibility]]
)
object JobArgs:
def apply(
addresses: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[String]]] = scala.None,
applicationInfo: besom.types.Input.Optional[besom.api.googlenative.jobs.v4.inputs.ApplicationInfoArgs] = scala.None,
company: besom.types.Input[String],
compensationInfo: besom.types.Input.Optional[besom.api.googlenative.jobs.v4.inputs.CompensationInfoArgs] = scala.None,
customAttributes: besom.types.Input.Optional[scala.Predef.Map[String, besom.types.Input[String]]] = scala.None,
degreeTypes: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[besom.api.googlenative.jobs.v4.enums.JobDegreeTypesItem]]] = scala.None,
department: besom.types.Input.Optional[String] = scala.None,
description: besom.types.Input[String],
employmentTypes: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[besom.api.googlenative.jobs.v4.enums.JobEmploymentTypesItem]]] = scala.None,
incentives: besom.types.Input.Optional[String] = scala.None,
jobBenefits: besom.types.Input.Optional[scala.collection.immutable.List[besom.types.Input[besom.api.googlenative.jobs.v4.enums.JobJobBenefitsItem]]] = scala.None,
jobEndTime: besom.types.Input.Optional[String] = scala.None,
jobLevel: besom.types.Input.Optional[besom.api.googlenative.jobs.v4.enums.JobJobLevel] = scala.None,
jobStartTime: besom.types.Input.Optional[String] = scala.None,
languageCode: besom.types.Input.Optional[String] = scala.None,
name: besom.types.Input.Optional[String] = scala.None,
postingExpireTime: besom.types.Input.Optional[String] = scala.None,
postingPublishTime: besom.types.Input.Optional[String] = scala.None,
postingRegion: besom.types.Input.Optional[besom.api.googlenative.jobs.v4.enums.JobPostingRegion] = scala.None,
processingOptions: besom.types.Input.Optional[besom.api.googlenative.jobs.v4.inputs.ProcessingOptionsArgs] = scala.None,
project: besom.types.Input.Optional[String] = scala.None,
promotionValue: besom.types.Input.Optional[Int] = scala.None,
qualifications: besom.types.Input.Optional[String] = scala.None,
requisitionId: besom.types.Input[String],
responsibilities: besom.types.Input.Optional[String] = scala.None,
tenantId: besom.types.Input[String],
title: besom.types.Input[String],
visibility: besom.types.Input.Optional[besom.api.googlenative.jobs.v4.enums.JobVisibility] = scala.None
)(using besom.types.Context): JobArgs =
new JobArgs(
addresses = addresses.asOptionOutput(isSecret = false),
applicationInfo = applicationInfo.asOptionOutput(isSecret = false),
company = company.asOutput(isSecret = false),
compensationInfo = compensationInfo.asOptionOutput(isSecret = false),
customAttributes = customAttributes.asOptionOutput(isSecret = false),
degreeTypes = degreeTypes.asOptionOutput(isSecret = false),
department = department.asOptionOutput(isSecret = false),
description = description.asOutput(isSecret = false),
employmentTypes = employmentTypes.asOptionOutput(isSecret = false),
incentives = incentives.asOptionOutput(isSecret = false),
jobBenefits = jobBenefits.asOptionOutput(isSecret = false),
jobEndTime = jobEndTime.asOptionOutput(isSecret = false),
jobLevel = jobLevel.asOptionOutput(isSecret = false),
jobStartTime = jobStartTime.asOptionOutput(isSecret = false),
languageCode = languageCode.asOptionOutput(isSecret = false),
name = name.asOptionOutput(isSecret = false),
postingExpireTime = postingExpireTime.asOptionOutput(isSecret = false),
postingPublishTime = postingPublishTime.asOptionOutput(isSecret = false),
postingRegion = postingRegion.asOptionOutput(isSecret = false),
processingOptions = processingOptions.asOptionOutput(isSecret = false),
project = project.asOptionOutput(isSecret = false),
promotionValue = promotionValue.asOptionOutput(isSecret = false),
qualifications = qualifications.asOptionOutput(isSecret = false),
requisitionId = requisitionId.asOutput(isSecret = false),
responsibilities = responsibilities.asOptionOutput(isSecret = false),
tenantId = tenantId.asOutput(isSecret = false),
title = title.asOutput(isSecret = false),
visibility = visibility.asOptionOutput(isSecret = false)
)
given encoder(using besom.types.Context): besom.types.Encoder[JobArgs] =
besom.internal.Encoder.derived[JobArgs]
given argsEncoder(using besom.types.Context): besom.types.ArgsEncoder[JobArgs] =
besom.internal.ArgsEncoder.derived[JobArgs]
© 2015 - 2025 Weber Informatics LLC | Privacy Policy