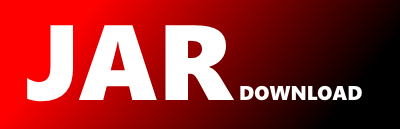
src.tpu.v2alpha1.Node.scala Maven / Gradle / Ivy
package besom.api.googlenative.tpu.v2alpha1
final case class Node private(
urn: besom.types.Output[besom.types.URN],
id: besom.types.Output[besom.types.ResourceId],
acceleratorConfig: besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.AcceleratorConfigResponse],
acceleratorType: besom.types.Output[String],
apiVersion: besom.types.Output[String],
autocheckpointEnabled: besom.types.Output[Boolean],
bootDiskConfig: besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.BootDiskConfigResponse],
cidrBlock: besom.types.Output[String],
createTime: besom.types.Output[String],
dataDisks: besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.tpu.v2alpha1.outputs.AttachedDiskResponse]],
description: besom.types.Output[String],
health: besom.types.Output[String],
healthDescription: besom.types.Output[String],
labels: besom.types.Output[scala.Predef.Map[String, String]],
location: besom.types.Output[String],
metadata: besom.types.Output[scala.Predef.Map[String, String]],
multisliceNode: besom.types.Output[Boolean],
name: besom.types.Output[String],
networkConfig: besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.NetworkConfigResponse],
networkEndpoints: besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.tpu.v2alpha1.outputs.NetworkEndpointResponse]],
nodeId: besom.types.Output[scala.Option[String]],
project: besom.types.Output[String],
queuedResource: besom.types.Output[String],
requestId: besom.types.Output[scala.Option[String]],
runtimeVersion: besom.types.Output[String],
schedulingConfig: besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.SchedulingConfigResponse],
serviceAccount: besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.ServiceAccountResponse],
shieldedInstanceConfig: besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.ShieldedInstanceConfigResponse],
state: besom.types.Output[String],
symptoms: besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.tpu.v2alpha1.outputs.SymptomResponse]],
tags: besom.types.Output[scala.collection.immutable.List[String]]
) extends besom.CustomResource
object Node extends besom.ResourceCompanion[Node]:
/** Resource constructor for Node.
*
* @param name [[besom.util.NonEmptyString]] The unique (stack-wise) name of the resource in Pulumi state (not on provider's side).
* NonEmptyString is inferred automatically from non-empty string literals, even when interpolated. If you encounter any
* issues with this, please try using `: NonEmptyString` type annotation. If you need to convert a dynamically generated
* string to NonEmptyString, use `NonEmptyString.apply` method - `NonEmptyString(str): Option[NonEmptyString]`.
*
* @param args [[NodeArgs]] The configuration to use to create this resource.
*
* @param opts [[besom.CustomResourceOptions]] Resource options to use for this resource.
* Defaults to empty options. If you need to set some options, use [[besom.opts]] function to create them, for example:
*
* {{{
* val res = Node(
* "my-resource",
* NodeArgs(...), // your args
* opts(provider = myProvider)
* )
* }}}
*/
def apply(using ctx: besom.types.Context)(
name: besom.util.NonEmptyString,
args: NodeArgs,
opts: besom.ResourceOptsVariant.Custom ?=> besom.CustomResourceOptions = besom.CustomResourceOptions()
): besom.types.Output[Node] =
ctx.readOrRegisterResource[Node, NodeArgs]("google-native:tpu/v2alpha1:Node", name, args, opts(using besom.ResourceOptsVariant.Custom))
private[besom] def typeToken: besom.types.ResourceType = "google-native:tpu/v2alpha1:Node"
given resourceDecoder(using besom.types.Context): besom.types.ResourceDecoder[Node] =
besom.internal.ResourceDecoder.derived[Node]
given decoder(using besom.types.Context): besom.types.Decoder[Node] =
besom.internal.Decoder.customResourceDecoder[Node]
given outputOps: {} with
extension(output: besom.types.Output[Node])
def urn : besom.types.Output[besom.types.URN] = output.flatMap(_.urn)
def id : besom.types.Output[besom.types.ResourceId] = output.flatMap(_.id)
def acceleratorConfig : besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.AcceleratorConfigResponse] = output.flatMap(_.acceleratorConfig)
def acceleratorType : besom.types.Output[String] = output.flatMap(_.acceleratorType)
def apiVersion : besom.types.Output[String] = output.flatMap(_.apiVersion)
def autocheckpointEnabled : besom.types.Output[Boolean] = output.flatMap(_.autocheckpointEnabled)
def bootDiskConfig : besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.BootDiskConfigResponse] = output.flatMap(_.bootDiskConfig)
def cidrBlock : besom.types.Output[String] = output.flatMap(_.cidrBlock)
def createTime : besom.types.Output[String] = output.flatMap(_.createTime)
def dataDisks : besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.tpu.v2alpha1.outputs.AttachedDiskResponse]] = output.flatMap(_.dataDisks)
def description : besom.types.Output[String] = output.flatMap(_.description)
def health : besom.types.Output[String] = output.flatMap(_.health)
def healthDescription : besom.types.Output[String] = output.flatMap(_.healthDescription)
def labels : besom.types.Output[scala.Predef.Map[String, String]] = output.flatMap(_.labels)
def location : besom.types.Output[String] = output.flatMap(_.location)
def metadata : besom.types.Output[scala.Predef.Map[String, String]] = output.flatMap(_.metadata)
def multisliceNode : besom.types.Output[Boolean] = output.flatMap(_.multisliceNode)
def name : besom.types.Output[String] = output.flatMap(_.name)
def networkConfig : besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.NetworkConfigResponse] = output.flatMap(_.networkConfig)
def networkEndpoints : besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.tpu.v2alpha1.outputs.NetworkEndpointResponse]] = output.flatMap(_.networkEndpoints)
def nodeId : besom.types.Output[scala.Option[String]] = output.flatMap(_.nodeId)
def project : besom.types.Output[String] = output.flatMap(_.project)
def queuedResource : besom.types.Output[String] = output.flatMap(_.queuedResource)
def requestId : besom.types.Output[scala.Option[String]] = output.flatMap(_.requestId)
def runtimeVersion : besom.types.Output[String] = output.flatMap(_.runtimeVersion)
def schedulingConfig : besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.SchedulingConfigResponse] = output.flatMap(_.schedulingConfig)
def serviceAccount : besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.ServiceAccountResponse] = output.flatMap(_.serviceAccount)
def shieldedInstanceConfig : besom.types.Output[besom.api.googlenative.tpu.v2alpha1.outputs.ShieldedInstanceConfigResponse] = output.flatMap(_.shieldedInstanceConfig)
def state : besom.types.Output[String] = output.flatMap(_.state)
def symptoms : besom.types.Output[scala.collection.immutable.List[besom.api.googlenative.tpu.v2alpha1.outputs.SymptomResponse]] = output.flatMap(_.symptoms)
def tags : besom.types.Output[scala.collection.immutable.List[String]] = output.flatMap(_.tags)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy