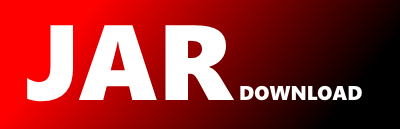
com.pulumi.aws.batch.kotlin.JobDefinition.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.batch.kotlin
import com.pulumi.aws.batch.kotlin.outputs.JobDefinitionEksProperties
import com.pulumi.aws.batch.kotlin.outputs.JobDefinitionRetryStrategy
import com.pulumi.aws.batch.kotlin.outputs.JobDefinitionTimeout
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.batch.kotlin.outputs.JobDefinitionEksProperties.Companion.toKotlin as jobDefinitionEksPropertiesToKotlin
import com.pulumi.aws.batch.kotlin.outputs.JobDefinitionRetryStrategy.Companion.toKotlin as jobDefinitionRetryStrategyToKotlin
import com.pulumi.aws.batch.kotlin.outputs.JobDefinitionTimeout.Companion.toKotlin as jobDefinitionTimeoutToKotlin
/**
* Builder for [JobDefinition].
*/
@PulumiTagMarker
public class JobDefinitionResourceBuilder internal constructor() {
public var name: String? = null
public var args: JobDefinitionArgs = JobDefinitionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend JobDefinitionArgsBuilder.() -> Unit) {
val builder = JobDefinitionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): JobDefinition {
val builtJavaResource = com.pulumi.aws.batch.JobDefinition(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return JobDefinition(builtJavaResource)
}
}
/**
* Provides a Batch Job Definition resource.
* ## Example Usage
* ### Job definition of type container
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.batch.JobDefinition("test", {
* name: "my_test_batch_job_definition",
* type: "container",
* containerProperties: JSON.stringify({
* command: [
* "ls",
* "-la",
* ],
* image: "busybox",
* resourceRequirements: [
* {
* type: "VCPU",
* value: "0.25",
* },
* {
* type: "MEMORY",
* value: "512",
* },
* ],
* volumes: [{
* host: {
* sourcePath: "/tmp",
* },
* name: "tmp",
* }],
* environment: [{
* name: "VARNAME",
* value: "VARVAL",
* }],
* mountPoints: [{
* sourceVolume: "tmp",
* containerPath: "/tmp",
* readOnly: false,
* }],
* ulimits: [{
* hardLimit: 1024,
* name: "nofile",
* softLimit: 1024,
* }],
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* test = aws.batch.JobDefinition("test",
* name="my_test_batch_job_definition",
* type="container",
* container_properties=json.dumps({
* "command": [
* "ls",
* "-la",
* ],
* "image": "busybox",
* "resourceRequirements": [
* {
* "type": "VCPU",
* "value": "0.25",
* },
* {
* "type": "MEMORY",
* "value": "512",
* },
* ],
* "volumes": [{
* "host": {
* "sourcePath": "/tmp",
* },
* "name": "tmp",
* }],
* "environment": [{
* "name": "VARNAME",
* "value": "VARVAL",
* }],
* "mountPoints": [{
* "sourceVolume": "tmp",
* "containerPath": "/tmp",
* "readOnly": False,
* }],
* "ulimits": [{
* "hardLimit": 1024,
* "name": "nofile",
* "softLimit": 1024,
* }],
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.Batch.JobDefinition("test", new()
* {
* Name = "my_test_batch_job_definition",
* Type = "container",
* ContainerProperties = JsonSerializer.Serialize(new Dictionary
* {
* ["command"] = new[]
* {
* "ls",
* "-la",
* },
* ["image"] = "busybox",
* ["resourceRequirements"] = new[]
* {
* new Dictionary
* {
* ["type"] = "VCPU",
* ["value"] = "0.25",
* },
* new Dictionary
* {
* ["type"] = "MEMORY",
* ["value"] = "512",
* },
* },
* ["volumes"] = new[]
* {
* new Dictionary
* {
* ["host"] = new Dictionary
* {
* ["sourcePath"] = "/tmp",
* },
* ["name"] = "tmp",
* },
* },
* ["environment"] = new[]
* {
* new Dictionary
* {
* ["name"] = "VARNAME",
* ["value"] = "VARVAL",
* },
* },
* ["mountPoints"] = new[]
* {
* new Dictionary
* {
* ["sourceVolume"] = "tmp",
* ["containerPath"] = "/tmp",
* ["readOnly"] = false,
* },
* },
* ["ulimits"] = new[]
* {
* new Dictionary
* {
* ["hardLimit"] = 1024,
* ["name"] = "nofile",
* ["softLimit"] = 1024,
* },
* },
* }),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/batch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "command": []string{
* "ls",
* "-la",
* },
* "image": "busybox",
* "resourceRequirements": []map[string]interface{}{
* map[string]interface{}{
* "type": "VCPU",
* "value": "0.25",
* },
* map[string]interface{}{
* "type": "MEMORY",
* "value": "512",
* },
* },
* "volumes": []map[string]interface{}{
* map[string]interface{}{
* "host": map[string]interface{}{
* "sourcePath": "/tmp",
* },
* "name": "tmp",
* },
* },
* "environment": []map[string]interface{}{
* map[string]interface{}{
* "name": "VARNAME",
* "value": "VARVAL",
* },
* },
* "mountPoints": []map[string]interface{}{
* map[string]interface{}{
* "sourceVolume": "tmp",
* "containerPath": "/tmp",
* "readOnly": false,
* },
* },
* "ulimits": []map[string]interface{}{
* map[string]interface{}{
* "hardLimit": 1024,
* "name": "nofile",
* "softLimit": 1024,
* },
* },
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = batch.NewJobDefinition(ctx, "test", &batch.JobDefinitionArgs{
* Name: pulumi.String("my_test_batch_job_definition"),
* Type: pulumi.String("container"),
* ContainerProperties: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.batch.JobDefinition;
* import com.pulumi.aws.batch.JobDefinitionArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new JobDefinition("test", JobDefinitionArgs.builder()
* .name("my_test_batch_job_definition")
* .type("container")
* .containerProperties(serializeJson(
* jsonObject(
* jsonProperty("command", jsonArray(
* "ls",
* "-la"
* )),
* jsonProperty("image", "busybox"),
* jsonProperty("resourceRequirements", jsonArray(
* jsonObject(
* jsonProperty("type", "VCPU"),
* jsonProperty("value", "0.25")
* ),
* jsonObject(
* jsonProperty("type", "MEMORY"),
* jsonProperty("value", "512")
* )
* )),
* jsonProperty("volumes", jsonArray(jsonObject(
* jsonProperty("host", jsonObject(
* jsonProperty("sourcePath", "/tmp")
* )),
* jsonProperty("name", "tmp")
* ))),
* jsonProperty("environment", jsonArray(jsonObject(
* jsonProperty("name", "VARNAME"),
* jsonProperty("value", "VARVAL")
* ))),
* jsonProperty("mountPoints", jsonArray(jsonObject(
* jsonProperty("sourceVolume", "tmp"),
* jsonProperty("containerPath", "/tmp"),
* jsonProperty("readOnly", false)
* ))),
* jsonProperty("ulimits", jsonArray(jsonObject(
* jsonProperty("hardLimit", 1024),
* jsonProperty("name", "nofile"),
* jsonProperty("softLimit", 1024)
* )))
* )))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:batch:JobDefinition
* properties:
* name: my_test_batch_job_definition
* type: container
* containerProperties:
* fn::toJSON:
* command:
* - ls
* - -la
* image: busybox
* resourceRequirements:
* - type: VCPU
* value: '0.25'
* - type: MEMORY
* value: '512'
* volumes:
* - host:
* sourcePath: /tmp
* name: tmp
* environment:
* - name: VARNAME
* value: VARVAL
* mountPoints:
* - sourceVolume: tmp
* containerPath: /tmp
* readOnly: false
* ulimits:
* - hardLimit: 1024
* name: nofile
* softLimit: 1024
* ```
*
* ### Job definition of type multinode
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.batch.JobDefinition("test", {
* name: "tf_test_batch_job_definition_multinode",
* type: "multinode",
* nodeProperties: JSON.stringify({
* mainNode: 0,
* nodeRangeProperties: [
* {
* container: {
* command: [
* "ls",
* "-la",
* ],
* image: "busybox",
* memory: 128,
* vcpus: 1,
* },
* targetNodes: "0:",
* },
* {
* container: {
* command: [
* "echo",
* "test",
* ],
* image: "busybox",
* memory: 128,
* vcpus: 1,
* },
* targetNodes: "1:",
* },
* ],
* numNodes: 2,
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* test = aws.batch.JobDefinition("test",
* name="tf_test_batch_job_definition_multinode",
* type="multinode",
* node_properties=json.dumps({
* "mainNode": 0,
* "nodeRangeProperties": [
* {
* "container": {
* "command": [
* "ls",
* "-la",
* ],
* "image": "busybox",
* "memory": 128,
* "vcpus": 1,
* },
* "targetNodes": "0:",
* },
* {
* "container": {
* "command": [
* "echo",
* "test",
* ],
* "image": "busybox",
* "memory": 128,
* "vcpus": 1,
* },
* "targetNodes": "1:",
* },
* ],
* "numNodes": 2,
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.Batch.JobDefinition("test", new()
* {
* Name = "tf_test_batch_job_definition_multinode",
* Type = "multinode",
* NodeProperties = JsonSerializer.Serialize(new Dictionary
* {
* ["mainNode"] = 0,
* ["nodeRangeProperties"] = new[]
* {
* new Dictionary
* {
* ["container"] = new Dictionary
* {
* ["command"] = new[]
* {
* "ls",
* "-la",
* },
* ["image"] = "busybox",
* ["memory"] = 128,
* ["vcpus"] = 1,
* },
* ["targetNodes"] = "0:",
* },
* new Dictionary
* {
* ["container"] = new Dictionary
* {
* ["command"] = new[]
* {
* "echo",
* "test",
* },
* ["image"] = "busybox",
* ["memory"] = 128,
* ["vcpus"] = 1,
* },
* ["targetNodes"] = "1:",
* },
* },
* ["numNodes"] = 2,
* }),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/batch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "mainNode": 0,
* "nodeRangeProperties": []map[string]interface{}{
* map[string]interface{}{
* "container": map[string]interface{}{
* "command": []string{
* "ls",
* "-la",
* },
* "image": "busybox",
* "memory": 128,
* "vcpus": 1,
* },
* "targetNodes": "0:",
* },
* map[string]interface{}{
* "container": map[string]interface{}{
* "command": []string{
* "echo",
* "test",
* },
* "image": "busybox",
* "memory": 128,
* "vcpus": 1,
* },
* "targetNodes": "1:",
* },
* },
* "numNodes": 2,
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = batch.NewJobDefinition(ctx, "test", &batch.JobDefinitionArgs{
* Name: pulumi.String("tf_test_batch_job_definition_multinode"),
* Type: pulumi.String("multinode"),
* NodeProperties: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.batch.JobDefinition;
* import com.pulumi.aws.batch.JobDefinitionArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new JobDefinition("test", JobDefinitionArgs.builder()
* .name("tf_test_batch_job_definition_multinode")
* .type("multinode")
* .nodeProperties(serializeJson(
* jsonObject(
* jsonProperty("mainNode", 0),
* jsonProperty("nodeRangeProperties", jsonArray(
* jsonObject(
* jsonProperty("container", jsonObject(
* jsonProperty("command", jsonArray(
* "ls",
* "-la"
* )),
* jsonProperty("image", "busybox"),
* jsonProperty("memory", 128),
* jsonProperty("vcpus", 1)
* )),
* jsonProperty("targetNodes", "0:")
* ),
* jsonObject(
* jsonProperty("container", jsonObject(
* jsonProperty("command", jsonArray(
* "echo",
* "test"
* )),
* jsonProperty("image", "busybox"),
* jsonProperty("memory", 128),
* jsonProperty("vcpus", 1)
* )),
* jsonProperty("targetNodes", "1:")
* )
* )),
* jsonProperty("numNodes", 2)
* )))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:batch:JobDefinition
* properties:
* name: tf_test_batch_job_definition_multinode
* type: multinode
* nodeProperties:
* fn::toJSON:
* mainNode: 0
* nodeRangeProperties:
* - container:
* command:
* - ls
* - -la
* image: busybox
* memory: 128
* vcpus: 1
* targetNodes: '0:'
* - container:
* command:
* - echo
* - test
* image: busybox
* memory: 128
* vcpus: 1
* targetNodes: '1:'
* numNodes: 2
* ```
*
* ### Job Definition of type EKS
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.batch.JobDefinition("test", {
* name: " tf_test_batch_job_definition_eks",
* type: "container",
* eksProperties: {
* podProperties: {
* hostNetwork: true,
* containers: {
* image: "public.ecr.aws/amazonlinux/amazonlinux:1",
* commands: [
* "sleep",
* "60",
* ],
* resources: {
* limits: {
* cpu: "1",
* memory: "1024Mi",
* },
* },
* },
* metadata: {
* labels: {
* environment: "test",
* },
* },
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test = aws.batch.JobDefinition("test",
* name=" tf_test_batch_job_definition_eks",
* type="container",
* eks_properties={
* "pod_properties": {
* "host_network": True,
* "containers": {
* "image": "public.ecr.aws/amazonlinux/amazonlinux:1",
* "commands": [
* "sleep",
* "60",
* ],
* "resources": {
* "limits": {
* "cpu": "1",
* "memory": "1024Mi",
* },
* },
* },
* "metadata": {
* "labels": {
* "environment": "test",
* },
* },
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.Batch.JobDefinition("test", new()
* {
* Name = " tf_test_batch_job_definition_eks",
* Type = "container",
* EksProperties = new Aws.Batch.Inputs.JobDefinitionEksPropertiesArgs
* {
* PodProperties = new Aws.Batch.Inputs.JobDefinitionEksPropertiesPodPropertiesArgs
* {
* HostNetwork = true,
* Containers = new Aws.Batch.Inputs.JobDefinitionEksPropertiesPodPropertiesContainersArgs
* {
* Image = "public.ecr.aws/amazonlinux/amazonlinux:1",
* Commands = new[]
* {
* "sleep",
* "60",
* },
* Resources = new Aws.Batch.Inputs.JobDefinitionEksPropertiesPodPropertiesContainersResourcesArgs
* {
* Limits =
* {
* { "cpu", "1" },
* { "memory", "1024Mi" },
* },
* },
* },
* Metadata = new Aws.Batch.Inputs.JobDefinitionEksPropertiesPodPropertiesMetadataArgs
* {
* Labels =
* {
* { "environment", "test" },
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/batch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewJobDefinition(ctx, "test", &batch.JobDefinitionArgs{
* Name: pulumi.String(" tf_test_batch_job_definition_eks"),
* Type: pulumi.String("container"),
* EksProperties: &batch.JobDefinitionEksPropertiesArgs{
* PodProperties: &batch.JobDefinitionEksPropertiesPodPropertiesArgs{
* HostNetwork: pulumi.Bool(true),
* Containers: &batch.JobDefinitionEksPropertiesPodPropertiesContainersArgs{
* Image: pulumi.String("public.ecr.aws/amazonlinux/amazonlinux:1"),
* Commands: pulumi.StringArray{
* pulumi.String("sleep"),
* pulumi.String("60"),
* },
* Resources: &batch.JobDefinitionEksPropertiesPodPropertiesContainersResourcesArgs{
* Limits: pulumi.StringMap{
* "cpu": pulumi.String("1"),
* "memory": pulumi.String("1024Mi"),
* },
* },
* },
* Metadata: &batch.JobDefinitionEksPropertiesPodPropertiesMetadataArgs{
* Labels: pulumi.StringMap{
* "environment": pulumi.String("test"),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.batch.JobDefinition;
* import com.pulumi.aws.batch.JobDefinitionArgs;
* import com.pulumi.aws.batch.inputs.JobDefinitionEksPropertiesArgs;
* import com.pulumi.aws.batch.inputs.JobDefinitionEksPropertiesPodPropertiesArgs;
* import com.pulumi.aws.batch.inputs.JobDefinitionEksPropertiesPodPropertiesContainersArgs;
* import com.pulumi.aws.batch.inputs.JobDefinitionEksPropertiesPodPropertiesContainersResourcesArgs;
* import com.pulumi.aws.batch.inputs.JobDefinitionEksPropertiesPodPropertiesMetadataArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new JobDefinition("test", JobDefinitionArgs.builder()
* .name(" tf_test_batch_job_definition_eks")
* .type("container")
* .eksProperties(JobDefinitionEksPropertiesArgs.builder()
* .podProperties(JobDefinitionEksPropertiesPodPropertiesArgs.builder()
* .hostNetwork(true)
* .containers(JobDefinitionEksPropertiesPodPropertiesContainersArgs.builder()
* .image("public.ecr.aws/amazonlinux/amazonlinux:1")
* .commands(
* "sleep",
* "60")
* .resources(JobDefinitionEksPropertiesPodPropertiesContainersResourcesArgs.builder()
* .limits(Map.ofEntries(
* Map.entry("cpu", "1"),
* Map.entry("memory", "1024Mi")
* ))
* .build())
* .build())
* .metadata(JobDefinitionEksPropertiesPodPropertiesMetadataArgs.builder()
* .labels(Map.of("environment", "test"))
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:batch:JobDefinition
* properties:
* name: ' tf_test_batch_job_definition_eks'
* type: container
* eksProperties:
* podProperties:
* hostNetwork: true
* containers:
* image: public.ecr.aws/amazonlinux/amazonlinux:1
* commands:
* - sleep
* - '60'
* resources:
* limits:
* cpu: '1'
* memory: 1024Mi
* metadata:
* labels:
* environment: test
* ```
*
* ### Fargate Platform Capability
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const assumeRolePolicy = aws.iam.getPolicyDocument({
* statements: [{
* actions: ["sts:AssumeRole"],
* principals: [{
* type: "Service",
* identifiers: ["ecs-tasks.amazonaws.com"],
* }],
* }],
* });
* const ecsTaskExecutionRole = new aws.iam.Role("ecs_task_execution_role", {
* name: "my_test_batch_exec_role",
* assumeRolePolicy: assumeRolePolicy.then(assumeRolePolicy => assumeRolePolicy.json),
* });
* const ecsTaskExecutionRolePolicy = new aws.iam.RolePolicyAttachment("ecs_task_execution_role_policy", {
* role: ecsTaskExecutionRole.name,
* policyArn: "arn:aws:iam::aws:policy/service-role/AmazonECSTaskExecutionRolePolicy",
* });
* const test = new aws.batch.JobDefinition("test", {
* name: "my_test_batch_job_definition",
* type: "container",
* platformCapabilities: ["FARGATE"],
* containerProperties: pulumi.jsonStringify({
* command: [
* "echo",
* "test",
* ],
* image: "busybox",
* jobRoleArn: "arn:aws:iam::123456789012:role/AWSBatchS3ReadOnly",
* fargatePlatformConfiguration: {
* platformVersion: "LATEST",
* },
* resourceRequirements: [
* {
* type: "VCPU",
* value: "0.25",
* },
* {
* type: "MEMORY",
* value: "512",
* },
* ],
* executionRoleArn: ecsTaskExecutionRole.arn,
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* assume_role_policy = aws.iam.get_policy_document(statements=[{
* "actions": ["sts:AssumeRole"],
* "principals": [{
* "type": "Service",
* "identifiers": ["ecs-tasks.amazonaws.com"],
* }],
* }])
* ecs_task_execution_role = aws.iam.Role("ecs_task_execution_role",
* name="my_test_batch_exec_role",
* assume_role_policy=assume_role_policy.json)
* ecs_task_execution_role_policy = aws.iam.RolePolicyAttachment("ecs_task_execution_role_policy",
* role=ecs_task_execution_role.name,
* policy_arn="arn:aws:iam::aws:policy/service-role/AmazonECSTaskExecutionRolePolicy")
* test = aws.batch.JobDefinition("test",
* name="my_test_batch_job_definition",
* type="container",
* platform_capabilities=["FARGATE"],
* container_properties=pulumi.Output.json_dumps({
* "command": [
* "echo",
* "test",
* ],
* "image": "busybox",
* "jobRoleArn": "arn:aws:iam::123456789012:role/AWSBatchS3ReadOnly",
* "fargatePlatformConfiguration": {
* "platformVersion": "LATEST",
* },
* "resourceRequirements": [
* {
* "type": "VCPU",
* "value": "0.25",
* },
* {
* "type": "MEMORY",
* "value": "512",
* },
* ],
* "executionRoleArn": ecs_task_execution_role.arn,
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var assumeRolePolicy = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Actions = new[]
* {
* "sts:AssumeRole",
* },
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "ecs-tasks.amazonaws.com",
* },
* },
* },
* },
* },
* });
* var ecsTaskExecutionRole = new Aws.Iam.Role("ecs_task_execution_role", new()
* {
* Name = "my_test_batch_exec_role",
* AssumeRolePolicy = assumeRolePolicy.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var ecsTaskExecutionRolePolicy = new Aws.Iam.RolePolicyAttachment("ecs_task_execution_role_policy", new()
* {
* Role = ecsTaskExecutionRole.Name,
* PolicyArn = "arn:aws:iam::aws:policy/service-role/AmazonECSTaskExecutionRolePolicy",
* });
* var test = new Aws.Batch.JobDefinition("test", new()
* {
* Name = "my_test_batch_job_definition",
* Type = "container",
* PlatformCapabilities = new[]
* {
* "FARGATE",
* },
* ContainerProperties = Output.JsonSerialize(Output.Create(new Dictionary
* {
* ["command"] = new[]
* {
* "echo",
* "test",
* },
* ["image"] = "busybox",
* ["jobRoleArn"] = "arn:aws:iam::123456789012:role/AWSBatchS3ReadOnly",
* ["fargatePlatformConfiguration"] = new Dictionary
* {
* ["platformVersion"] = "LATEST",
* },
* ["resourceRequirements"] = new[]
* {
* new Dictionary
* {
* ["type"] = "VCPU",
* ["value"] = "0.25",
* },
* new Dictionary
* {
* ["type"] = "MEMORY",
* ["value"] = "512",
* },
* },
* ["executionRoleArn"] = ecsTaskExecutionRole.Arn,
* })),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/batch"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* assumeRolePolicy, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Actions: []string{
* "sts:AssumeRole",
* },
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* "ecs-tasks.amazonaws.com",
* },
* },
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* ecsTaskExecutionRole, err := iam.NewRole(ctx, "ecs_task_execution_role", &iam.RoleArgs{
* Name: pulumi.String("my_test_batch_exec_role"),
* AssumeRolePolicy: pulumi.String(assumeRolePolicy.Json),
* })
* if err != nil {
* return err
* }
* _, err = iam.NewRolePolicyAttachment(ctx, "ecs_task_execution_role_policy", &iam.RolePolicyAttachmentArgs{
* Role: ecsTaskExecutionRole.Name,
* PolicyArn: pulumi.String("arn:aws:iam::aws:policy/service-role/AmazonECSTaskExecutionRolePolicy"),
* })
* if err != nil {
* return err
* }
* _, err = batch.NewJobDefinition(ctx, "test", &batch.JobDefinitionArgs{
* Name: pulumi.String("my_test_batch_job_definition"),
* Type: pulumi.String("container"),
* PlatformCapabilities: pulumi.StringArray{
* pulumi.String("FARGATE"),
* },
* ContainerProperties: ecsTaskExecutionRole.Arn.ApplyT(func(arn string) (pulumi.String, error) {
* var _zero pulumi.String
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "command": []string{
* "echo",
* "test",
* },
* "image": "busybox",
* "jobRoleArn": "arn:aws:iam::123456789012:role/AWSBatchS3ReadOnly",
* "fargatePlatformConfiguration": map[string]interface{}{
* "platformVersion": "LATEST",
* },
* "resourceRequirements": []map[string]interface{}{
* map[string]interface{}{
* "type": "VCPU",
* "value": "0.25",
* },
* map[string]interface{}{
* "type": "MEMORY",
* "value": "512",
* },
* },
* "executionRoleArn": arn,
* })
* if err != nil {
* return _zero, err
* }
* json0 := string(tmpJSON0)
* return pulumi.String(json0), nil
* }).(pulumi.StringOutput),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import com.pulumi.aws.batch.JobDefinition;
* import com.pulumi.aws.batch.JobDefinitionArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var assumeRolePolicy = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .actions("sts:AssumeRole")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("ecs-tasks.amazonaws.com")
* .build())
* .build())
* .build());
* var ecsTaskExecutionRole = new Role("ecsTaskExecutionRole", RoleArgs.builder()
* .name("my_test_batch_exec_role")
* .assumeRolePolicy(assumeRolePolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var ecsTaskExecutionRolePolicy = new RolePolicyAttachment("ecsTaskExecutionRolePolicy", RolePolicyAttachmentArgs.builder()
* .role(ecsTaskExecutionRole.name())
* .policyArn("arn:aws:iam::aws:policy/service-role/AmazonECSTaskExecutionRolePolicy")
* .build());
* var test = new JobDefinition("test", JobDefinitionArgs.builder()
* .name("my_test_batch_job_definition")
* .type("container")
* .platformCapabilities("FARGATE")
* .containerProperties(ecsTaskExecutionRole.arn().applyValue(arn -> serializeJson(
* jsonObject(
* jsonProperty("command", jsonArray(
* "echo",
* "test"
* )),
* jsonProperty("image", "busybox"),
* jsonProperty("jobRoleArn", "arn:aws:iam::123456789012:role/AWSBatchS3ReadOnly"),
* jsonProperty("fargatePlatformConfiguration", jsonObject(
* jsonProperty("platformVersion", "LATEST")
* )),
* jsonProperty("resourceRequirements", jsonArray(
* jsonObject(
* jsonProperty("type", "VCPU"),
* jsonProperty("value", "0.25")
* ),
* jsonObject(
* jsonProperty("type", "MEMORY"),
* jsonProperty("value", "512")
* )
* )),
* jsonProperty("executionRoleArn", arn)
* ))))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* ecsTaskExecutionRole:
* type: aws:iam:Role
* name: ecs_task_execution_role
* properties:
* name: my_test_batch_exec_role
* assumeRolePolicy: ${assumeRolePolicy.json}
* ecsTaskExecutionRolePolicy:
* type: aws:iam:RolePolicyAttachment
* name: ecs_task_execution_role_policy
* properties:
* role: ${ecsTaskExecutionRole.name}
* policyArn: arn:aws:iam::aws:policy/service-role/AmazonECSTaskExecutionRolePolicy
* test:
* type: aws:batch:JobDefinition
* properties:
* name: my_test_batch_job_definition
* type: container
* platformCapabilities:
* - FARGATE
* containerProperties:
* fn::toJSON:
* command:
* - echo
* - test
* image: busybox
* jobRoleArn: arn:aws:iam::123456789012:role/AWSBatchS3ReadOnly
* fargatePlatformConfiguration:
* platformVersion: LATEST
* resourceRequirements:
* - type: VCPU
* value: '0.25'
* - type: MEMORY
* value: '512'
* executionRoleArn: ${ecsTaskExecutionRole.arn}
* variables:
* assumeRolePolicy:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - actions:
* - sts:AssumeRole
* principals:
* - type: Service
* identifiers:
* - ecs-tasks.amazonaws.com
* ```
*
* ### Job definition of type container using `ecs_properties`
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.batch.JobDefinition("test", {
* name: "my_test_batch_job_definition",
* type: "container",
* platformCapabilities: ["FARGATE"],
* ecsProperties: JSON.stringify({
* taskProperties: [{
* executionRoleArn: ecsTaskExecutionRole.arn,
* containers: [
* {
* image: "public.ecr.aws/amazonlinux/amazonlinux:1",
* command: [
* "sleep",
* "60",
* ],
* dependsOn: [{
* containerName: "container_b",
* condition: "COMPLETE",
* }],
* secrets: [{
* name: "TEST",
* valueFrom: "DUMMY",
* }],
* environment: [{
* name: "test",
* value: "Environment Variable",
* }],
* essential: true,
* logConfiguration: {
* logDriver: "awslogs",
* options: {
* "awslogs-group": "tf_test_batch_job",
* "awslogs-region": "us-west-2",
* "awslogs-stream-prefix": "ecs",
* },
* },
* name: "container_a",
* privileged: false,
* readonlyRootFilesystem: false,
* resourceRequirements: [
* {
* value: "1.0",
* type: "VCPU",
* },
* {
* value: "2048",
* type: "MEMORY",
* },
* ],
* },
* {
* image: "public.ecr.aws/amazonlinux/amazonlinux:1",
* command: [
* "sleep",
* "360",
* ],
* name: "container_b",
* essential: false,
* resourceRequirements: [
* {
* value: "1.0",
* type: "VCPU",
* },
* {
* value: "2048",
* type: "MEMORY",
* },
* ],
* },
* ],
* }],
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* test = aws.batch.JobDefinition("test",
* name="my_test_batch_job_definition",
* type="container",
* platform_capabilities=["FARGATE"],
* ecs_properties=json.dumps({
* "taskProperties": [{
* "executionRoleArn": ecs_task_execution_role["arn"],
* "containers": [
* {
* "image": "public.ecr.aws/amazonlinux/amazonlinux:1",
* "command": [
* "sleep",
* "60",
* ],
* "dependsOn": [{
* "containerName": "container_b",
* "condition": "COMPLETE",
* }],
* "secrets": [{
* "name": "TEST",
* "valueFrom": "DUMMY",
* }],
* "environment": [{
* "name": "test",
* "value": "Environment Variable",
* }],
* "essential": True,
* "logConfiguration": {
* "logDriver": "awslogs",
* "options": {
* "awslogs-group": "tf_test_batch_job",
* "awslogs-region": "us-west-2",
* "awslogs-stream-prefix": "ecs",
* },
* },
* "name": "container_a",
* "privileged": False,
* "readonlyRootFilesystem": False,
* "resourceRequirements": [
* {
* "value": "1.0",
* "type": "VCPU",
* },
* {
* "value": "2048",
* "type": "MEMORY",
* },
* ],
* },
* {
* "image": "public.ecr.aws/amazonlinux/amazonlinux:1",
* "command": [
* "sleep",
* "360",
* ],
* "name": "container_b",
* "essential": False,
* "resourceRequirements": [
* {
* "value": "1.0",
* "type": "VCPU",
* },
* {
* "value": "2048",
* "type": "MEMORY",
* },
* ],
* },
* ],
* }],
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.Batch.JobDefinition("test", new()
* {
* Name = "my_test_batch_job_definition",
* Type = "container",
* PlatformCapabilities = new[]
* {
* "FARGATE",
* },
* EcsProperties = JsonSerializer.Serialize(new Dictionary
* {
* ["taskProperties"] = new[]
* {
* new Dictionary
* {
* ["executionRoleArn"] = ecsTaskExecutionRole.Arn,
* ["containers"] = new[]
* {
* new Dictionary
* {
* ["image"] = "public.ecr.aws/amazonlinux/amazonlinux:1",
* ["command"] = new[]
* {
* "sleep",
* "60",
* },
* ["dependsOn"] = new[]
* {
* new Dictionary
* {
* ["containerName"] = "container_b",
* ["condition"] = "COMPLETE",
* },
* },
* ["secrets"] = new[]
* {
* new Dictionary
* {
* ["name"] = "TEST",
* ["valueFrom"] = "DUMMY",
* },
* },
* ["environment"] = new[]
* {
* new Dictionary
* {
* ["name"] = "test",
* ["value"] = "Environment Variable",
* },
* },
* ["essential"] = true,
* ["logConfiguration"] = new Dictionary
* {
* ["logDriver"] = "awslogs",
* ["options"] = new Dictionary
* {
* ["awslogs-group"] = "tf_test_batch_job",
* ["awslogs-region"] = "us-west-2",
* ["awslogs-stream-prefix"] = "ecs",
* },
* },
* ["name"] = "container_a",
* ["privileged"] = false,
* ["readonlyRootFilesystem"] = false,
* ["resourceRequirements"] = new[]
* {
* new Dictionary
* {
* ["value"] = "1.0",
* ["type"] = "VCPU",
* },
* new Dictionary
* {
* ["value"] = "2048",
* ["type"] = "MEMORY",
* },
* },
* },
* new Dictionary
* {
* ["image"] = "public.ecr.aws/amazonlinux/amazonlinux:1",
* ["command"] = new[]
* {
* "sleep",
* "360",
* },
* ["name"] = "container_b",
* ["essential"] = false,
* ["resourceRequirements"] = new[]
* {
* new Dictionary
* {
* ["value"] = "1.0",
* ["type"] = "VCPU",
* },
* new Dictionary
* {
* ["value"] = "2048",
* ["type"] = "MEMORY",
* },
* },
* },
* },
* },
* },
* }),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/batch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "taskProperties": []map[string]interface{}{
* map[string]interface{}{
* "executionRoleArn": ecsTaskExecutionRole.Arn,
* "containers": []interface{}{
* map[string]interface{}{
* "image": "public.ecr.aws/amazonlinux/amazonlinux:1",
* "command": []string{
* "sleep",
* "60",
* },
* "dependsOn": []map[string]interface{}{
* map[string]interface{}{
* "containerName": "container_b",
* "condition": "COMPLETE",
* },
* },
* "secrets": []map[string]interface{}{
* map[string]interface{}{
* "name": "TEST",
* "valueFrom": "DUMMY",
* },
* },
* "environment": []map[string]interface{}{
* map[string]interface{}{
* "name": "test",
* "value": "Environment Variable",
* },
* },
* "essential": true,
* "logConfiguration": map[string]interface{}{
* "logDriver": "awslogs",
* "options": map[string]interface{}{
* "awslogs-group": "tf_test_batch_job",
* "awslogs-region": "us-west-2",
* "awslogs-stream-prefix": "ecs",
* },
* },
* "name": "container_a",
* "privileged": false,
* "readonlyRootFilesystem": false,
* "resourceRequirements": []map[string]interface{}{
* map[string]interface{}{
* "value": "1.0",
* "type": "VCPU",
* },
* map[string]interface{}{
* "value": "2048",
* "type": "MEMORY",
* },
* },
* },
* map[string]interface{}{
* "image": "public.ecr.aws/amazonlinux/amazonlinux:1",
* "command": []string{
* "sleep",
* "360",
* },
* "name": "container_b",
* "essential": false,
* "resourceRequirements": []map[string]interface{}{
* map[string]interface{}{
* "value": "1.0",
* "type": "VCPU",
* },
* map[string]interface{}{
* "value": "2048",
* "type": "MEMORY",
* },
* },
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = batch.NewJobDefinition(ctx, "test", &batch.JobDefinitionArgs{
* Name: pulumi.String("my_test_batch_job_definition"),
* Type: pulumi.String("container"),
* PlatformCapabilities: pulumi.StringArray{
* pulumi.String("FARGATE"),
* },
* EcsProperties: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.batch.JobDefinition;
* import com.pulumi.aws.batch.JobDefinitionArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new JobDefinition("test", JobDefinitionArgs.builder()
* .name("my_test_batch_job_definition")
* .type("container")
* .platformCapabilities("FARGATE")
* .ecsProperties(serializeJson(
* jsonObject(
* jsonProperty("taskProperties", jsonArray(jsonObject(
* jsonProperty("executionRoleArn", ecsTaskExecutionRole.arn()),
* jsonProperty("containers", jsonArray(
* jsonObject(
* jsonProperty("image", "public.ecr.aws/amazonlinux/amazonlinux:1"),
* jsonProperty("command", jsonArray(
* "sleep",
* "60"
* )),
* jsonProperty("dependsOn", jsonArray(jsonObject(
* jsonProperty("containerName", "container_b"),
* jsonProperty("condition", "COMPLETE")
* ))),
* jsonProperty("secrets", jsonArray(jsonObject(
* jsonProperty("name", "TEST"),
* jsonProperty("valueFrom", "DUMMY")
* ))),
* jsonProperty("environment", jsonArray(jsonObject(
* jsonProperty("name", "test"),
* jsonProperty("value", "Environment Variable")
* ))),
* jsonProperty("essential", true),
* jsonProperty("logConfiguration", jsonObject(
* jsonProperty("logDriver", "awslogs"),
* jsonProperty("options", jsonObject(
* jsonProperty("awslogs-group", "tf_test_batch_job"),
* jsonProperty("awslogs-region", "us-west-2"),
* jsonProperty("awslogs-stream-prefix", "ecs")
* ))
* )),
* jsonProperty("name", "container_a"),
* jsonProperty("privileged", false),
* jsonProperty("readonlyRootFilesystem", false),
* jsonProperty("resourceRequirements", jsonArray(
* jsonObject(
* jsonProperty("value", "1.0"),
* jsonProperty("type", "VCPU")
* ),
* jsonObject(
* jsonProperty("value", "2048"),
* jsonProperty("type", "MEMORY")
* )
* ))
* ),
* jsonObject(
* jsonProperty("image", "public.ecr.aws/amazonlinux/amazonlinux:1"),
* jsonProperty("command", jsonArray(
* "sleep",
* "360"
* )),
* jsonProperty("name", "container_b"),
* jsonProperty("essential", false),
* jsonProperty("resourceRequirements", jsonArray(
* jsonObject(
* jsonProperty("value", "1.0"),
* jsonProperty("type", "VCPU")
* ),
* jsonObject(
* jsonProperty("value", "2048"),
* jsonProperty("type", "MEMORY")
* )
* ))
* )
* ))
* )))
* )))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:batch:JobDefinition
* properties:
* name: my_test_batch_job_definition
* type: container
* platformCapabilities:
* - FARGATE
* ecsProperties:
* fn::toJSON:
* taskProperties:
* - executionRoleArn: ${ecsTaskExecutionRole.arn}
* containers:
* - image: public.ecr.aws/amazonlinux/amazonlinux:1
* command:
* - sleep
* - '60'
* dependsOn:
* - containerName: container_b
* condition: COMPLETE
* secrets:
* - name: TEST
* valueFrom: DUMMY
* environment:
* - name: test
* value: Environment Variable
* essential: true
* logConfiguration:
* logDriver: awslogs
* options:
* awslogs-group: tf_test_batch_job
* awslogs-region: us-west-2
* awslogs-stream-prefix: ecs
* name: container_a
* privileged: false
* readonlyRootFilesystem: false
* resourceRequirements:
* - value: '1.0'
* type: VCPU
* - value: '2048'
* type: MEMORY
* - image: public.ecr.aws/amazonlinux/amazonlinux:1
* command:
* - sleep
* - '360'
* name: container_b
* essential: false
* resourceRequirements:
* - value: '1.0'
* type: VCPU
* - value: '2048'
* type: MEMORY
* ```
*
* ## Import
* Using `pulumi import`, import Batch Job Definition using the `arn`. For example:
* ```sh
* $ pulumi import aws:batch/jobDefinition:JobDefinition test arn:aws:batch:us-east-1:123456789012:job-definition/sample
* ```
*/
public class JobDefinition internal constructor(
override val javaResource: com.pulumi.aws.batch.JobDefinition,
) : KotlinCustomResource(javaResource, JobDefinitionMapper) {
/**
* ARN of the job definition, includes revision (`:#`).
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* ARN without the revision number.
*/
public val arnPrefix: Output
get() = javaResource.arnPrefix().applyValue({ args0 -> args0 })
/**
* Valid [container properties](http://docs.aws.amazon.com/batch/latest/APIReference/API_RegisterJobDefinition.html) provided as a single valid JSON document. This parameter is only valid if the `type` parameter is `container`.
*/
public val containerProperties: Output?
get() = javaResource.containerProperties().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* When updating a job definition a new revision is created. This parameter determines if the previous version is `deregistered` (`INACTIVE`) or left `ACTIVE`. Defaults to `true`.
*/
public val deregisterOnNewRevision: Output?
get() = javaResource.deregisterOnNewRevision().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Valid [ECS properties](http://docs.aws.amazon.com/batch/latest/APIReference/API_RegisterJobDefinition.html) provided as a single valid JSON document. This parameter is only valid if the `type` parameter is `container`.
*/
public val ecsProperties: Output?
get() = javaResource.ecsProperties().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Valid eks properties. This parameter is only valid if the `type` parameter is `container`.
*/
public val eksProperties: Output?
get() = javaResource.eksProperties().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> jobDefinitionEksPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* Name of the job definition.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Valid [node properties](http://docs.aws.amazon.com/batch/latest/APIReference/API_RegisterJobDefinition.html) provided as a single valid JSON document. This parameter is required if the `type` parameter is `multinode`.
*/
public val nodeProperties: Output?
get() = javaResource.nodeProperties().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Parameter substitution placeholders to set in the job definition.
*/
public val parameters: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy