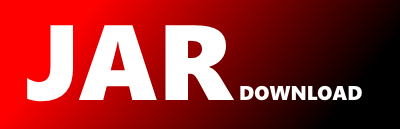
com.pulumi.aws.cloudwatch.kotlin.EventBusArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cloudwatch.kotlin
import com.pulumi.aws.cloudwatch.EventBusArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides an EventBridge event bus resource.
* > **Note:** EventBridge was formerly known as CloudWatch Events. The functionality is identical.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const messenger = new aws.cloudwatch.EventBus("messenger", {name: "chat-messages"});
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* messenger = aws.cloudwatch.EventBus("messenger", name="chat-messages")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var messenger = new Aws.CloudWatch.EventBus("messenger", new()
* {
* Name = "chat-messages",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cloudwatch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudwatch.NewEventBus(ctx, "messenger", &cloudwatch.EventBusArgs{
* Name: pulumi.String("chat-messages"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.EventBus;
* import com.pulumi.aws.cloudwatch.EventBusArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var messenger = new EventBus("messenger", EventBusArgs.builder()
* .name("chat-messages")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* messenger:
* type: aws:cloudwatch:EventBus
* properties:
* name: chat-messages
* ```
*
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const examplepartner = aws.cloudwatch.getEventSource({
* namePrefix: "aws.partner/examplepartner.com",
* });
* const examplepartnerEventBus = new aws.cloudwatch.EventBus("examplepartner", {
* name: examplepartner.then(examplepartner => examplepartner.name),
* eventSourceName: examplepartner.then(examplepartner => examplepartner.name),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* examplepartner = aws.cloudwatch.get_event_source(name_prefix="aws.partner/examplepartner.com")
* examplepartner_event_bus = aws.cloudwatch.EventBus("examplepartner",
* name=examplepartner.name,
* event_source_name=examplepartner.name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var examplepartner = Aws.CloudWatch.GetEventSource.Invoke(new()
* {
* NamePrefix = "aws.partner/examplepartner.com",
* });
* var examplepartnerEventBus = new Aws.CloudWatch.EventBus("examplepartner", new()
* {
* Name = examplepartner.Apply(getEventSourceResult => getEventSourceResult.Name),
* EventSourceName = examplepartner.Apply(getEventSourceResult => getEventSourceResult.Name),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cloudwatch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* examplepartner, err := cloudwatch.GetEventSource(ctx, &cloudwatch.GetEventSourceArgs{
* NamePrefix: pulumi.StringRef("aws.partner/examplepartner.com"),
* }, nil)
* if err != nil {
* return err
* }
* _, err = cloudwatch.NewEventBus(ctx, "examplepartner", &cloudwatch.EventBusArgs{
* Name: pulumi.String(examplepartner.Name),
* EventSourceName: pulumi.String(examplepartner.Name),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.CloudwatchFunctions;
* import com.pulumi.aws.cloudwatch.inputs.GetEventSourceArgs;
* import com.pulumi.aws.cloudwatch.EventBus;
* import com.pulumi.aws.cloudwatch.EventBusArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var examplepartner = CloudwatchFunctions.getEventSource(GetEventSourceArgs.builder()
* .namePrefix("aws.partner/examplepartner.com")
* .build());
* var examplepartnerEventBus = new EventBus("examplepartnerEventBus", EventBusArgs.builder()
* .name(examplepartner.applyValue(getEventSourceResult -> getEventSourceResult.name()))
* .eventSourceName(examplepartner.applyValue(getEventSourceResult -> getEventSourceResult.name()))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* examplepartnerEventBus:
* type: aws:cloudwatch:EventBus
* name: examplepartner
* properties:
* name: ${examplepartner.name}
* eventSourceName: ${examplepartner.name}
* variables:
* examplepartner:
* fn::invoke:
* Function: aws:cloudwatch:getEventSource
* Arguments:
* namePrefix: aws.partner/examplepartner.com
* ```
*
* ## Import
* Using `pulumi import`, import EventBridge event buses using the `name` (which can also be a partner event source name). For example:
* ```sh
* $ pulumi import aws:cloudwatch/eventBus:EventBus messenger chat-messages
* ```
* @property eventSourceName The partner event source that the new event bus will be matched with. Must match `name`.
* @property kmsKeyIdentifier The identifier of the AWS KMS customer managed key for EventBridge to use, if you choose to use a customer managed key to encrypt events on this event bus. The identifier can be the key Amazon Resource Name (ARN), KeyId, key alias, or key alias ARN.
* @property name The name of the new event bus. The names of custom event buses can't contain the / character. To create a partner event bus, ensure the `name` matches the `event_source_name`.
* @property tags A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public data class EventBusArgs(
public val eventSourceName: Output? = null,
public val kmsKeyIdentifier: Output? = null,
public val name: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy