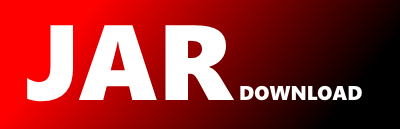
com.pulumi.aws.cloudwatch.kotlin.LogAccountPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cloudwatch.kotlin
import com.pulumi.aws.cloudwatch.LogAccountPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides a CloudWatch Log Account Policy resource.
* ## Example Usage
* ### Account Data Protection Policy
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const dataProtection = new aws.cloudwatch.LogAccountPolicy("data_protection", {
* policyName: "data-protection",
* policyType: "DATA_PROTECTION_POLICY",
* policyDocument: JSON.stringify({
* Name: "DataProtection",
* Version: "2021-06-01",
* Statement: [
* {
* Sid: "Audit",
* DataIdentifier: ["arn:aws:dataprotection::aws:data-identifier/EmailAddress"],
* Operation: {
* Audit: {
* FindingsDestination: {},
* },
* },
* },
* {
* Sid: "Redact",
* DataIdentifier: ["arn:aws:dataprotection::aws:data-identifier/EmailAddress"],
* Operation: {
* Deidentify: {
* MaskConfig: {},
* },
* },
* },
* ],
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* data_protection = aws.cloudwatch.LogAccountPolicy("data_protection",
* policy_name="data-protection",
* policy_type="DATA_PROTECTION_POLICY",
* policy_document=json.dumps({
* "Name": "DataProtection",
* "Version": "2021-06-01",
* "Statement": [
* {
* "Sid": "Audit",
* "DataIdentifier": ["arn:aws:dataprotection::aws:data-identifier/EmailAddress"],
* "Operation": {
* "Audit": {
* "FindingsDestination": {},
* },
* },
* },
* {
* "Sid": "Redact",
* "DataIdentifier": ["arn:aws:dataprotection::aws:data-identifier/EmailAddress"],
* "Operation": {
* "Deidentify": {
* "MaskConfig": {},
* },
* },
* },
* ],
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var dataProtection = new Aws.CloudWatch.LogAccountPolicy("data_protection", new()
* {
* PolicyName = "data-protection",
* PolicyType = "DATA_PROTECTION_POLICY",
* PolicyDocument = JsonSerializer.Serialize(new Dictionary
* {
* ["Name"] = "DataProtection",
* ["Version"] = "2021-06-01",
* ["Statement"] = new[]
* {
* new Dictionary
* {
* ["Sid"] = "Audit",
* ["DataIdentifier"] = new[]
* {
* "arn:aws:dataprotection::aws:data-identifier/EmailAddress",
* },
* ["Operation"] = new Dictionary
* {
* ["Audit"] = new Dictionary
* {
* ["FindingsDestination"] = new Dictionary
* {
* },
* },
* },
* },
* new Dictionary
* {
* ["Sid"] = "Redact",
* ["DataIdentifier"] = new[]
* {
* "arn:aws:dataprotection::aws:data-identifier/EmailAddress",
* },
* ["Operation"] = new Dictionary
* {
* ["Deidentify"] = new Dictionary
* {
* ["MaskConfig"] = new Dictionary
* {
* },
* },
* },
* },
* },
* }),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cloudwatch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "Name": "DataProtection",
* "Version": "2021-06-01",
* "Statement": []interface{}{
* map[string]interface{}{
* "Sid": "Audit",
* "DataIdentifier": []string{
* "arn:aws:dataprotection::aws:data-identifier/EmailAddress",
* },
* "Operation": map[string]interface{}{
* "Audit": map[string]interface{}{
* "FindingsDestination": nil,
* },
* },
* },
* map[string]interface{}{
* "Sid": "Redact",
* "DataIdentifier": []string{
* "arn:aws:dataprotection::aws:data-identifier/EmailAddress",
* },
* "Operation": map[string]interface{}{
* "Deidentify": map[string]interface{}{
* "MaskConfig": nil,
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = cloudwatch.NewLogAccountPolicy(ctx, "data_protection", &cloudwatch.LogAccountPolicyArgs{
* PolicyName: pulumi.String("data-protection"),
* PolicyType: pulumi.String("DATA_PROTECTION_POLICY"),
* PolicyDocument: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.LogAccountPolicy;
* import com.pulumi.aws.cloudwatch.LogAccountPolicyArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var dataProtection = new LogAccountPolicy("dataProtection", LogAccountPolicyArgs.builder()
* .policyName("data-protection")
* .policyType("DATA_PROTECTION_POLICY")
* .policyDocument(serializeJson(
* jsonObject(
* jsonProperty("Name", "DataProtection"),
* jsonProperty("Version", "2021-06-01"),
* jsonProperty("Statement", jsonArray(
* jsonObject(
* jsonProperty("Sid", "Audit"),
* jsonProperty("DataIdentifier", jsonArray("arn:aws:dataprotection::aws:data-identifier/EmailAddress")),
* jsonProperty("Operation", jsonObject(
* jsonProperty("Audit", jsonObject(
* jsonProperty("FindingsDestination", jsonObject(
* ))
* ))
* ))
* ),
* jsonObject(
* jsonProperty("Sid", "Redact"),
* jsonProperty("DataIdentifier", jsonArray("arn:aws:dataprotection::aws:data-identifier/EmailAddress")),
* jsonProperty("Operation", jsonObject(
* jsonProperty("Deidentify", jsonObject(
* jsonProperty("MaskConfig", jsonObject(
* ))
* ))
* ))
* )
* ))
* )))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* dataProtection:
* type: aws:cloudwatch:LogAccountPolicy
* name: data_protection
* properties:
* policyName: data-protection
* policyType: DATA_PROTECTION_POLICY
* policyDocument:
* fn::toJSON:
* Name: DataProtection
* Version: 2021-06-01
* Statement:
* - Sid: Audit
* DataIdentifier:
* - arn:aws:dataprotection::aws:data-identifier/EmailAddress
* Operation:
* Audit:
* FindingsDestination: {}
* - Sid: Redact
* DataIdentifier:
* - arn:aws:dataprotection::aws:data-identifier/EmailAddress
* Operation:
* Deidentify:
* MaskConfig: {}
* ```
*
* ### Subscription Filter Policy
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const subscriptionFilter = new aws.cloudwatch.LogAccountPolicy("subscription_filter", {
* policyName: "subscription-filter",
* policyType: "SUBSCRIPTION_FILTER_POLICY",
* policyDocument: JSON.stringify({
* DestinationArn: test.arn,
* FilterPattern: "test",
* }),
* selectionCriteria: "LogGroupName NOT IN [\"excluded_log_group_name\"]",
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* subscription_filter = aws.cloudwatch.LogAccountPolicy("subscription_filter",
* policy_name="subscription-filter",
* policy_type="SUBSCRIPTION_FILTER_POLICY",
* policy_document=json.dumps({
* "DestinationArn": test["arn"],
* "FilterPattern": "test",
* }),
* selection_criteria="LogGroupName NOT IN [\"excluded_log_group_name\"]")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var subscriptionFilter = new Aws.CloudWatch.LogAccountPolicy("subscription_filter", new()
* {
* PolicyName = "subscription-filter",
* PolicyType = "SUBSCRIPTION_FILTER_POLICY",
* PolicyDocument = JsonSerializer.Serialize(new Dictionary
* {
* ["DestinationArn"] = test.Arn,
* ["FilterPattern"] = "test",
* }),
* SelectionCriteria = "LogGroupName NOT IN [\"excluded_log_group_name\"]",
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cloudwatch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "DestinationArn": test.Arn,
* "FilterPattern": "test",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = cloudwatch.NewLogAccountPolicy(ctx, "subscription_filter", &cloudwatch.LogAccountPolicyArgs{
* PolicyName: pulumi.String("subscription-filter"),
* PolicyType: pulumi.String("SUBSCRIPTION_FILTER_POLICY"),
* PolicyDocument: pulumi.String(json0),
* SelectionCriteria: pulumi.String("LogGroupName NOT IN [\"excluded_log_group_name\"]"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.LogAccountPolicy;
* import com.pulumi.aws.cloudwatch.LogAccountPolicyArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var subscriptionFilter = new LogAccountPolicy("subscriptionFilter", LogAccountPolicyArgs.builder()
* .policyName("subscription-filter")
* .policyType("SUBSCRIPTION_FILTER_POLICY")
* .policyDocument(serializeJson(
* jsonObject(
* jsonProperty("DestinationArn", test.arn()),
* jsonProperty("FilterPattern", "test")
* )))
* .selectionCriteria("LogGroupName NOT IN [\"excluded_log_group_name\"]")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* subscriptionFilter:
* type: aws:cloudwatch:LogAccountPolicy
* name: subscription_filter
* properties:
* policyName: subscription-filter
* policyType: SUBSCRIPTION_FILTER_POLICY
* policyDocument:
* fn::toJSON:
* DestinationArn: ${test.arn}
* FilterPattern: test
* selectionCriteria: LogGroupName NOT IN ["excluded_log_group_name"]
* ```
*
* ## Import
* Using `pulumi import`, import this resource using the `policy_name` and `policy_type` separated by `:`. For example:
* ```sh
* $ pulumi import aws:cloudwatch/logAccountPolicy:LogAccountPolicy example "my-account-policy:SUBSCRIPTION_FILTER_POLICY"
* ```
* @property policyDocument Text of the account policy. Refer to the [AWS docs](https://docs.aws.amazon.com/cli/latest/reference/logs/put-account-policy.html) for more information.
* @property policyName Name of the account policy.
* @property policyType Type of account policy. Either `DATA_PROTECTION_POLICY` or `SUBSCRIPTION_FILTER_POLICY`. You can have one account policy per type in an account.
* @property scope Currently defaults to and only accepts the value: `ALL`.
* @property selectionCriteria Criteria for applying a subscription filter policy to a selection of log groups. The only allowable criteria selector is `LogGroupName NOT IN []`.
*/
public data class LogAccountPolicyArgs(
public val policyDocument: Output? = null,
public val policyName: Output? = null,
public val policyType: Output? = null,
public val scope: Output? = null,
public val selectionCriteria: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cloudwatch.LogAccountPolicyArgs =
com.pulumi.aws.cloudwatch.LogAccountPolicyArgs.builder()
.policyDocument(policyDocument?.applyValue({ args0 -> args0 }))
.policyName(policyName?.applyValue({ args0 -> args0 }))
.policyType(policyType?.applyValue({ args0 -> args0 }))
.scope(scope?.applyValue({ args0 -> args0 }))
.selectionCriteria(selectionCriteria?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LogAccountPolicyArgs].
*/
@PulumiTagMarker
public class LogAccountPolicyArgsBuilder internal constructor() {
private var policyDocument: Output? = null
private var policyName: Output? = null
private var policyType: Output? = null
private var scope: Output? = null
private var selectionCriteria: Output? = null
/**
* @param value Text of the account policy. Refer to the [AWS docs](https://docs.aws.amazon.com/cli/latest/reference/logs/put-account-policy.html) for more information.
*/
@JvmName("hqnaipbhkqqscelp")
public suspend fun policyDocument(`value`: Output) {
this.policyDocument = value
}
/**
* @param value Name of the account policy.
*/
@JvmName("cdoncosfxgkemkee")
public suspend fun policyName(`value`: Output) {
this.policyName = value
}
/**
* @param value Type of account policy. Either `DATA_PROTECTION_POLICY` or `SUBSCRIPTION_FILTER_POLICY`. You can have one account policy per type in an account.
*/
@JvmName("secytqjjhuapvved")
public suspend fun policyType(`value`: Output) {
this.policyType = value
}
/**
* @param value Currently defaults to and only accepts the value: `ALL`.
*/
@JvmName("kvfsyiybtkhbmgka")
public suspend fun scope(`value`: Output) {
this.scope = value
}
/**
* @param value Criteria for applying a subscription filter policy to a selection of log groups. The only allowable criteria selector is `LogGroupName NOT IN []`.
*/
@JvmName("yfnsaoxrefammlur")
public suspend fun selectionCriteria(`value`: Output) {
this.selectionCriteria = value
}
/**
* @param value Text of the account policy. Refer to the [AWS docs](https://docs.aws.amazon.com/cli/latest/reference/logs/put-account-policy.html) for more information.
*/
@JvmName("aqpyoirbtgrhrkwd")
public suspend fun policyDocument(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policyDocument = mapped
}
/**
* @param value Name of the account policy.
*/
@JvmName("engwjtydxgonamht")
public suspend fun policyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policyName = mapped
}
/**
* @param value Type of account policy. Either `DATA_PROTECTION_POLICY` or `SUBSCRIPTION_FILTER_POLICY`. You can have one account policy per type in an account.
*/
@JvmName("hyitnxwrsgjyegim")
public suspend fun policyType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policyType = mapped
}
/**
* @param value Currently defaults to and only accepts the value: `ALL`.
*/
@JvmName("qeghmcfhcgihvpys")
public suspend fun scope(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scope = mapped
}
/**
* @param value Criteria for applying a subscription filter policy to a selection of log groups. The only allowable criteria selector is `LogGroupName NOT IN []`.
*/
@JvmName("qebudqqsuvfmuyna")
public suspend fun selectionCriteria(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.selectionCriteria = mapped
}
internal fun build(): LogAccountPolicyArgs = LogAccountPolicyArgs(
policyDocument = policyDocument,
policyName = policyName,
policyType = policyType,
scope = scope,
selectionCriteria = selectionCriteria,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy