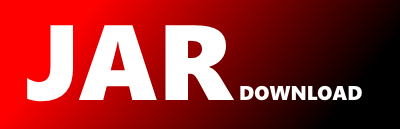
com.pulumi.aws.ec2.kotlin.VpcIpamPool.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [VpcIpamPool].
*/
@PulumiTagMarker
public class VpcIpamPoolResourceBuilder internal constructor() {
public var name: String? = null
public var args: VpcIpamPoolArgs = VpcIpamPoolArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend VpcIpamPoolArgsBuilder.() -> Unit) {
val builder = VpcIpamPoolArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): VpcIpamPool {
val builtJavaResource = com.pulumi.aws.ec2.VpcIpamPool(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return VpcIpamPool(builtJavaResource)
}
}
/**
* Provides an IP address pool resource for IPAM.
* ## Example Usage
* Basic usage:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const current = aws.getRegion({});
* const example = new aws.ec2.VpcIpam("example", {operatingRegions: [{
* regionName: current.then(current => current.name),
* }]});
* const exampleVpcIpamPool = new aws.ec2.VpcIpamPool("example", {
* addressFamily: "ipv4",
* ipamScopeId: example.privateDefaultScopeId,
* locale: current.then(current => current.name),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* current = aws.get_region()
* example = aws.ec2.VpcIpam("example", operating_regions=[{
* "region_name": current.name,
* }])
* example_vpc_ipam_pool = aws.ec2.VpcIpamPool("example",
* address_family="ipv4",
* ipam_scope_id=example.private_default_scope_id,
* locale=current.name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var current = Aws.GetRegion.Invoke();
* var example = new Aws.Ec2.VpcIpam("example", new()
* {
* OperatingRegions = new[]
* {
* new Aws.Ec2.Inputs.VpcIpamOperatingRegionArgs
* {
* RegionName = current.Apply(getRegionResult => getRegionResult.Name),
* },
* },
* });
* var exampleVpcIpamPool = new Aws.Ec2.VpcIpamPool("example", new()
* {
* AddressFamily = "ipv4",
* IpamScopeId = example.PrivateDefaultScopeId,
* Locale = current.Apply(getRegionResult => getRegionResult.Name),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := aws.GetRegion(ctx, nil, nil)
* if err != nil {
* return err
* }
* example, err := ec2.NewVpcIpam(ctx, "example", &ec2.VpcIpamArgs{
* OperatingRegions: ec2.VpcIpamOperatingRegionArray{
* &ec2.VpcIpamOperatingRegionArgs{
* RegionName: pulumi.String(current.Name),
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = ec2.NewVpcIpamPool(ctx, "example", &ec2.VpcIpamPoolArgs{
* AddressFamily: pulumi.String("ipv4"),
* IpamScopeId: example.PrivateDefaultScopeId,
* Locale: pulumi.String(current.Name),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetRegionArgs;
* import com.pulumi.aws.ec2.VpcIpam;
* import com.pulumi.aws.ec2.VpcIpamArgs;
* import com.pulumi.aws.ec2.inputs.VpcIpamOperatingRegionArgs;
* import com.pulumi.aws.ec2.VpcIpamPool;
* import com.pulumi.aws.ec2.VpcIpamPoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = AwsFunctions.getRegion();
* var example = new VpcIpam("example", VpcIpamArgs.builder()
* .operatingRegions(VpcIpamOperatingRegionArgs.builder()
* .regionName(current.applyValue(getRegionResult -> getRegionResult.name()))
* .build())
* .build());
* var exampleVpcIpamPool = new VpcIpamPool("exampleVpcIpamPool", VpcIpamPoolArgs.builder()
* .addressFamily("ipv4")
* .ipamScopeId(example.privateDefaultScopeId())
* .locale(current.applyValue(getRegionResult -> getRegionResult.name()))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ec2:VpcIpam
* properties:
* operatingRegions:
* - regionName: ${current.name}
* exampleVpcIpamPool:
* type: aws:ec2:VpcIpamPool
* name: example
* properties:
* addressFamily: ipv4
* ipamScopeId: ${example.privateDefaultScopeId}
* locale: ${current.name}
* variables:
* current:
* fn::invoke:
* Function: aws:getRegion
* Arguments: {}
* ```
*
* Nested Pools:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const current = aws.getRegion({});
* const example = new aws.ec2.VpcIpam("example", {operatingRegions: [{
* regionName: current.then(current => current.name),
* }]});
* const parent = new aws.ec2.VpcIpamPool("parent", {
* addressFamily: "ipv4",
* ipamScopeId: example.privateDefaultScopeId,
* });
* const parentTest = new aws.ec2.VpcIpamPoolCidr("parent_test", {
* ipamPoolId: parent.id,
* cidr: "172.20.0.0/16",
* });
* const child = new aws.ec2.VpcIpamPool("child", {
* addressFamily: "ipv4",
* ipamScopeId: example.privateDefaultScopeId,
* locale: current.then(current => current.name),
* sourceIpamPoolId: parent.id,
* });
* const childTest = new aws.ec2.VpcIpamPoolCidr("child_test", {
* ipamPoolId: child.id,
* cidr: "172.20.0.0/24",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* current = aws.get_region()
* example = aws.ec2.VpcIpam("example", operating_regions=[{
* "region_name": current.name,
* }])
* parent = aws.ec2.VpcIpamPool("parent",
* address_family="ipv4",
* ipam_scope_id=example.private_default_scope_id)
* parent_test = aws.ec2.VpcIpamPoolCidr("parent_test",
* ipam_pool_id=parent.id,
* cidr="172.20.0.0/16")
* child = aws.ec2.VpcIpamPool("child",
* address_family="ipv4",
* ipam_scope_id=example.private_default_scope_id,
* locale=current.name,
* source_ipam_pool_id=parent.id)
* child_test = aws.ec2.VpcIpamPoolCidr("child_test",
* ipam_pool_id=child.id,
* cidr="172.20.0.0/24")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var current = Aws.GetRegion.Invoke();
* var example = new Aws.Ec2.VpcIpam("example", new()
* {
* OperatingRegions = new[]
* {
* new Aws.Ec2.Inputs.VpcIpamOperatingRegionArgs
* {
* RegionName = current.Apply(getRegionResult => getRegionResult.Name),
* },
* },
* });
* var parent = new Aws.Ec2.VpcIpamPool("parent", new()
* {
* AddressFamily = "ipv4",
* IpamScopeId = example.PrivateDefaultScopeId,
* });
* var parentTest = new Aws.Ec2.VpcIpamPoolCidr("parent_test", new()
* {
* IpamPoolId = parent.Id,
* Cidr = "172.20.0.0/16",
* });
* var child = new Aws.Ec2.VpcIpamPool("child", new()
* {
* AddressFamily = "ipv4",
* IpamScopeId = example.PrivateDefaultScopeId,
* Locale = current.Apply(getRegionResult => getRegionResult.Name),
* SourceIpamPoolId = parent.Id,
* });
* var childTest = new Aws.Ec2.VpcIpamPoolCidr("child_test", new()
* {
* IpamPoolId = child.Id,
* Cidr = "172.20.0.0/24",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := aws.GetRegion(ctx, nil, nil)
* if err != nil {
* return err
* }
* example, err := ec2.NewVpcIpam(ctx, "example", &ec2.VpcIpamArgs{
* OperatingRegions: ec2.VpcIpamOperatingRegionArray{
* &ec2.VpcIpamOperatingRegionArgs{
* RegionName: pulumi.String(current.Name),
* },
* },
* })
* if err != nil {
* return err
* }
* parent, err := ec2.NewVpcIpamPool(ctx, "parent", &ec2.VpcIpamPoolArgs{
* AddressFamily: pulumi.String("ipv4"),
* IpamScopeId: example.PrivateDefaultScopeId,
* })
* if err != nil {
* return err
* }
* _, err = ec2.NewVpcIpamPoolCidr(ctx, "parent_test", &ec2.VpcIpamPoolCidrArgs{
* IpamPoolId: parent.ID(),
* Cidr: pulumi.String("172.20.0.0/16"),
* })
* if err != nil {
* return err
* }
* child, err := ec2.NewVpcIpamPool(ctx, "child", &ec2.VpcIpamPoolArgs{
* AddressFamily: pulumi.String("ipv4"),
* IpamScopeId: example.PrivateDefaultScopeId,
* Locale: pulumi.String(current.Name),
* SourceIpamPoolId: parent.ID(),
* })
* if err != nil {
* return err
* }
* _, err = ec2.NewVpcIpamPoolCidr(ctx, "child_test", &ec2.VpcIpamPoolCidrArgs{
* IpamPoolId: child.ID(),
* Cidr: pulumi.String("172.20.0.0/24"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetRegionArgs;
* import com.pulumi.aws.ec2.VpcIpam;
* import com.pulumi.aws.ec2.VpcIpamArgs;
* import com.pulumi.aws.ec2.inputs.VpcIpamOperatingRegionArgs;
* import com.pulumi.aws.ec2.VpcIpamPool;
* import com.pulumi.aws.ec2.VpcIpamPoolArgs;
* import com.pulumi.aws.ec2.VpcIpamPoolCidr;
* import com.pulumi.aws.ec2.VpcIpamPoolCidrArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = AwsFunctions.getRegion();
* var example = new VpcIpam("example", VpcIpamArgs.builder()
* .operatingRegions(VpcIpamOperatingRegionArgs.builder()
* .regionName(current.applyValue(getRegionResult -> getRegionResult.name()))
* .build())
* .build());
* var parent = new VpcIpamPool("parent", VpcIpamPoolArgs.builder()
* .addressFamily("ipv4")
* .ipamScopeId(example.privateDefaultScopeId())
* .build());
* var parentTest = new VpcIpamPoolCidr("parentTest", VpcIpamPoolCidrArgs.builder()
* .ipamPoolId(parent.id())
* .cidr("172.20.0.0/16")
* .build());
* var child = new VpcIpamPool("child", VpcIpamPoolArgs.builder()
* .addressFamily("ipv4")
* .ipamScopeId(example.privateDefaultScopeId())
* .locale(current.applyValue(getRegionResult -> getRegionResult.name()))
* .sourceIpamPoolId(parent.id())
* .build());
* var childTest = new VpcIpamPoolCidr("childTest", VpcIpamPoolCidrArgs.builder()
* .ipamPoolId(child.id())
* .cidr("172.20.0.0/24")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ec2:VpcIpam
* properties:
* operatingRegions:
* - regionName: ${current.name}
* parent:
* type: aws:ec2:VpcIpamPool
* properties:
* addressFamily: ipv4
* ipamScopeId: ${example.privateDefaultScopeId}
* parentTest:
* type: aws:ec2:VpcIpamPoolCidr
* name: parent_test
* properties:
* ipamPoolId: ${parent.id}
* cidr: 172.20.0.0/16
* child:
* type: aws:ec2:VpcIpamPool
* properties:
* addressFamily: ipv4
* ipamScopeId: ${example.privateDefaultScopeId}
* locale: ${current.name}
* sourceIpamPoolId: ${parent.id}
* childTest:
* type: aws:ec2:VpcIpamPoolCidr
* name: child_test
* properties:
* ipamPoolId: ${child.id}
* cidr: 172.20.0.0/24
* variables:
* current:
* fn::invoke:
* Function: aws:getRegion
* Arguments: {}
* ```
*
* ## Import
* Using `pulumi import`, import IPAMs using the IPAM pool `id`. For example:
* ```sh
* $ pulumi import aws:ec2/vpcIpamPool:VpcIpamPool example ipam-pool-0958f95207d978e1e
* ```
*/
public class VpcIpamPool internal constructor(
override val javaResource: com.pulumi.aws.ec2.VpcIpamPool,
) : KotlinCustomResource(javaResource, VpcIpamPoolMapper) {
/**
* The IP protocol assigned to this pool. You must choose either IPv4 or IPv6 protocol for a pool.
*/
public val addressFamily: Output
get() = javaResource.addressFamily().applyValue({ args0 -> args0 })
/**
* A default netmask length for allocations added to this pool. If, for example, the CIDR assigned to this pool is 10.0.0.0/8 and you enter 16 here, new allocations will default to 10.0.0.0/16 (unless you provide a different netmask value when you create the new allocation).
*/
public val allocationDefaultNetmaskLength: Output?
get() = javaResource.allocationDefaultNetmaskLength().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum netmask length that will be required for CIDR allocations in this pool.
*/
public val allocationMaxNetmaskLength: Output?
get() = javaResource.allocationMaxNetmaskLength().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The minimum netmask length that will be required for CIDR allocations in this pool.
*/
public val allocationMinNetmaskLength: Output?
get() = javaResource.allocationMinNetmaskLength().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Tags that are required for resources that use CIDRs from this IPAM pool. Resources that do not have these tags will not be allowed to allocate space from the pool. If the resources have their tags changed after they have allocated space or if the allocation tagging requirements are changed on the pool, the resource may be marked as noncompliant.
*/
public val allocationResourceTags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy