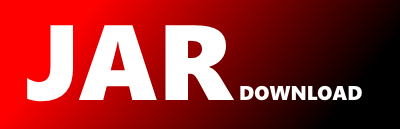
com.pulumi.aws.ecs.kotlin.inputs.ServiceServiceConnectConfigurationServiceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ecs.kotlin.inputs
import com.pulumi.aws.ecs.inputs.ServiceServiceConnectConfigurationServiceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property clientAlias List of client aliases for this Service Connect service. You use these to assign names that can be used by client applications. The maximum number of client aliases that you can have in this list is 1. See below.
* @property discoveryName Name of the new AWS Cloud Map service that Amazon ECS creates for this Amazon ECS service.
* @property ingressPortOverride Port number for the Service Connect proxy to listen on.
* @property portName Name of one of the `portMappings` from all the containers in the task definition of this Amazon ECS service.
* @property timeout Configuration timeouts for Service Connect
* @property tls Configuration for enabling Transport Layer Security (TLS)
*/
public data class ServiceServiceConnectConfigurationServiceArgs(
public val clientAlias: Output>? =
null,
public val discoveryName: Output? = null,
public val ingressPortOverride: Output? = null,
public val portName: Output,
public val timeout: Output? = null,
public val tls: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.ecs.inputs.ServiceServiceConnectConfigurationServiceArgs =
com.pulumi.aws.ecs.inputs.ServiceServiceConnectConfigurationServiceArgs.builder()
.clientAlias(
clientAlias?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.discoveryName(discoveryName?.applyValue({ args0 -> args0 }))
.ingressPortOverride(ingressPortOverride?.applyValue({ args0 -> args0 }))
.portName(portName.applyValue({ args0 -> args0 }))
.timeout(timeout?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tls(tls?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ServiceServiceConnectConfigurationServiceArgs].
*/
@PulumiTagMarker
public class ServiceServiceConnectConfigurationServiceArgsBuilder internal constructor() {
private var clientAlias: Output>? =
null
private var discoveryName: Output? = null
private var ingressPortOverride: Output? = null
private var portName: Output? = null
private var timeout: Output? = null
private var tls: Output? = null
/**
* @param value List of client aliases for this Service Connect service. You use these to assign names that can be used by client applications. The maximum number of client aliases that you can have in this list is 1. See below.
*/
@JvmName("dlvwogbthvrapoal")
public suspend fun clientAlias(`value`: Output>) {
this.clientAlias = value
}
@JvmName("wlwgealohilkgqef")
public suspend fun clientAlias(vararg values: Output) {
this.clientAlias = Output.all(values.asList())
}
/**
* @param values List of client aliases for this Service Connect service. You use these to assign names that can be used by client applications. The maximum number of client aliases that you can have in this list is 1. See below.
*/
@JvmName("ylpbiqqydtndxdwf")
public suspend fun clientAlias(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy