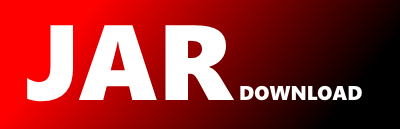
com.pulumi.aws.kinesis.kotlin.VideoStreamArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kinesis.kotlin
import com.pulumi.aws.kinesis.VideoStreamArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a Kinesis Video Stream resource. Amazon Kinesis Video Streams makes it easy to securely stream video from connected devices to AWS for analytics, machine learning (ML), playback, and other processing.
* For more details, see the [Amazon Kinesis Documentation](https://aws.amazon.com/documentation/kinesis/).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const _default = new aws.kinesis.VideoStream("default", {
* name: "kinesis-video-stream",
* dataRetentionInHours: 1,
* deviceName: "kinesis-video-device-name",
* mediaType: "video/h264",
* tags: {
* Name: "kinesis-video-stream",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* default = aws.kinesis.VideoStream("default",
* name="kinesis-video-stream",
* data_retention_in_hours=1,
* device_name="kinesis-video-device-name",
* media_type="video/h264",
* tags={
* "Name": "kinesis-video-stream",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Aws.Kinesis.VideoStream("default", new()
* {
* Name = "kinesis-video-stream",
* DataRetentionInHours = 1,
* DeviceName = "kinesis-video-device-name",
* MediaType = "video/h264",
* Tags =
* {
* { "Name", "kinesis-video-stream" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kinesis"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kinesis.NewVideoStream(ctx, "default", &kinesis.VideoStreamArgs{
* Name: pulumi.String("kinesis-video-stream"),
* DataRetentionInHours: pulumi.Int(1),
* DeviceName: pulumi.String("kinesis-video-device-name"),
* MediaType: pulumi.String("video/h264"),
* Tags: pulumi.StringMap{
* "Name": pulumi.String("kinesis-video-stream"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kinesis.VideoStream;
* import com.pulumi.aws.kinesis.VideoStreamArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new VideoStream("default", VideoStreamArgs.builder()
* .name("kinesis-video-stream")
* .dataRetentionInHours(1)
* .deviceName("kinesis-video-device-name")
* .mediaType("video/h264")
* .tags(Map.of("Name", "kinesis-video-stream"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: aws:kinesis:VideoStream
* properties:
* name: kinesis-video-stream
* dataRetentionInHours: 1
* deviceName: kinesis-video-device-name
* mediaType: video/h264
* tags:
* Name: kinesis-video-stream
* ```
*
* ## Import
* Using `pulumi import`, import Kinesis Streams using the `arn`. For example:
* ```sh
* $ pulumi import aws:kinesis/videoStream:VideoStream test_stream arn:aws:kinesisvideo:us-west-2:123456789012:stream/pulumi-kinesis-test/1554978910975
* ```
* @property dataRetentionInHours The number of hours that you want to retain the data in the stream. Kinesis Video Streams retains the data in a data store that is associated with the stream. The default value is `0`, indicating that the stream does not persist data.
* @property deviceName The name of the device that is writing to the stream. **In the current implementation, Kinesis Video Streams does not use this name.**
* @property kmsKeyId The ID of the AWS Key Management Service (AWS KMS) key that you want Kinesis Video Streams to use to encrypt stream data. If no key ID is specified, the default, Kinesis Video-managed key (`aws/kinesisvideo`) is used.
* @property mediaType The media type of the stream. Consumers of the stream can use this information when processing the stream. For more information about media types, see [Media Types](http://www.iana.org/assignments/media-types/media-types.xhtml). If you choose to specify the MediaType, see [Naming Requirements](https://tools.ietf.org/html/rfc6838#section-4.2) for guidelines.
* @property name A name to identify the stream. This is unique to the
* AWS account and region the Stream is created in.
* @property tags A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public data class VideoStreamArgs(
public val dataRetentionInHours: Output? = null,
public val deviceName: Output? = null,
public val kmsKeyId: Output? = null,
public val mediaType: Output? = null,
public val name: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy