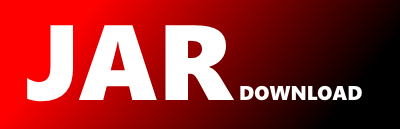
com.pulumi.aws.redshift.kotlin.inputs.ClusterLoggingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.redshift.kotlin.inputs
import com.pulumi.aws.redshift.inputs.ClusterLoggingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property bucketName The name of an existing S3 bucket where the log files are to be stored. Must be in the same region as the cluster and the cluster must have read bucket and put object permissions.
* For more information on the permissions required for the bucket, please read the AWS [documentation](http://docs.aws.amazon.com/redshift/latest/mgmt/db-auditing.html#db-auditing-enable-logging)
* @property enable Enables logging information such as queries and connection attempts, for the specified Amazon Redshift cluster.
* @property logDestinationType The log destination type. An enum with possible values of `s3` and `cloudwatch`.
* @property logExports The collection of exported log types. Log types include the connection log, user log and user activity log. Required when `log_destination_type` is `cloudwatch`. Valid log types are `connectionlog`, `userlog`, and `useractivitylog`.
* @property s3KeyPrefix The prefix applied to the log file names.
*/
public data class ClusterLoggingArgs(
public val bucketName: Output? = null,
public val enable: Output,
public val logDestinationType: Output? = null,
public val logExports: Output>? = null,
public val s3KeyPrefix: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.redshift.inputs.ClusterLoggingArgs =
com.pulumi.aws.redshift.inputs.ClusterLoggingArgs.builder()
.bucketName(bucketName?.applyValue({ args0 -> args0 }))
.enable(enable.applyValue({ args0 -> args0 }))
.logDestinationType(logDestinationType?.applyValue({ args0 -> args0 }))
.logExports(logExports?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.s3KeyPrefix(s3KeyPrefix?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ClusterLoggingArgs].
*/
@PulumiTagMarker
public class ClusterLoggingArgsBuilder internal constructor() {
private var bucketName: Output? = null
private var enable: Output? = null
private var logDestinationType: Output? = null
private var logExports: Output>? = null
private var s3KeyPrefix: Output? = null
/**
* @param value The name of an existing S3 bucket where the log files are to be stored. Must be in the same region as the cluster and the cluster must have read bucket and put object permissions.
* For more information on the permissions required for the bucket, please read the AWS [documentation](http://docs.aws.amazon.com/redshift/latest/mgmt/db-auditing.html#db-auditing-enable-logging)
*/
@JvmName("eexdqahofmcccpxu")
public suspend fun bucketName(`value`: Output) {
this.bucketName = value
}
/**
* @param value Enables logging information such as queries and connection attempts, for the specified Amazon Redshift cluster.
*/
@JvmName("mbhnlgnslsikjxyh")
public suspend fun enable(`value`: Output) {
this.enable = value
}
/**
* @param value The log destination type. An enum with possible values of `s3` and `cloudwatch`.
*/
@JvmName("dqjjpmqqjvikpsaq")
public suspend fun logDestinationType(`value`: Output) {
this.logDestinationType = value
}
/**
* @param value The collection of exported log types. Log types include the connection log, user log and user activity log. Required when `log_destination_type` is `cloudwatch`. Valid log types are `connectionlog`, `userlog`, and `useractivitylog`.
*/
@JvmName("lorwbemtexyllvvr")
public suspend fun logExports(`value`: Output>) {
this.logExports = value
}
@JvmName("aopagwqetcacqacx")
public suspend fun logExports(vararg values: Output) {
this.logExports = Output.all(values.asList())
}
/**
* @param values The collection of exported log types. Log types include the connection log, user log and user activity log. Required when `log_destination_type` is `cloudwatch`. Valid log types are `connectionlog`, `userlog`, and `useractivitylog`.
*/
@JvmName("dbfvcpyttqehqajs")
public suspend fun logExports(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy