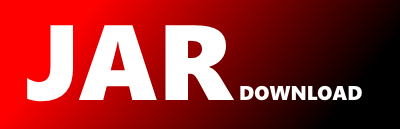
com.pulumi.aws.account.kotlin.PrimaryContactArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.account.kotlin
import com.pulumi.aws.account.PrimaryContactArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages the specified primary contact information associated with an AWS Account.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.account.PrimaryContact("test", {
* addressLine1: "123 Any Street",
* city: "Seattle",
* companyName: "Example Corp, Inc.",
* countryCode: "US",
* districtOrCounty: "King",
* fullName: "My Name",
* phoneNumber: "+64211111111",
* postalCode: "98101",
* stateOrRegion: "WA",
* websiteUrl: "https://www.examplecorp.com",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test = aws.account.PrimaryContact("test",
* address_line1="123 Any Street",
* city="Seattle",
* company_name="Example Corp, Inc.",
* country_code="US",
* district_or_county="King",
* full_name="My Name",
* phone_number="+64211111111",
* postal_code="98101",
* state_or_region="WA",
* website_url="https://www.examplecorp.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.Account.PrimaryContact("test", new()
* {
* AddressLine1 = "123 Any Street",
* City = "Seattle",
* CompanyName = "Example Corp, Inc.",
* CountryCode = "US",
* DistrictOrCounty = "King",
* FullName = "My Name",
* PhoneNumber = "+64211111111",
* PostalCode = "98101",
* StateOrRegion = "WA",
* WebsiteUrl = "https://www.examplecorp.com",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/account"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := account.NewPrimaryContact(ctx, "test", &account.PrimaryContactArgs{
* AddressLine1: pulumi.String("123 Any Street"),
* City: pulumi.String("Seattle"),
* CompanyName: pulumi.String("Example Corp, Inc."),
* CountryCode: pulumi.String("US"),
* DistrictOrCounty: pulumi.String("King"),
* FullName: pulumi.String("My Name"),
* PhoneNumber: pulumi.String("+64211111111"),
* PostalCode: pulumi.String("98101"),
* StateOrRegion: pulumi.String("WA"),
* WebsiteUrl: pulumi.String("https://www.examplecorp.com"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.account.PrimaryContact;
* import com.pulumi.aws.account.PrimaryContactArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new PrimaryContact("test", PrimaryContactArgs.builder()
* .addressLine1("123 Any Street")
* .city("Seattle")
* .companyName("Example Corp, Inc.")
* .countryCode("US")
* .districtOrCounty("King")
* .fullName("My Name")
* .phoneNumber("+64211111111")
* .postalCode("98101")
* .stateOrRegion("WA")
* .websiteUrl("https://www.examplecorp.com")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:account:PrimaryContact
* properties:
* addressLine1: 123 Any Street
* city: Seattle
* companyName: Example Corp, Inc.
* countryCode: US
* districtOrCounty: King
* fullName: My Name
* phoneNumber: '+64211111111'
* postalCode: '98101'
* stateOrRegion: WA
* websiteUrl: https://www.examplecorp.com
* ```
*
* ## Import
* Using `pulumi import`, import the Primary Contact using the `account_id`. For example:
* ```sh
* $ pulumi import aws:account/primaryContact:PrimaryContact test 1234567890
* ```
* @property accountId The ID of the target account when managing member accounts. Will manage current user's account by default if omitted.
* @property addressLine1 The first line of the primary contact address.
* @property addressLine2 The second line of the primary contact address, if any.
* @property addressLine3 The third line of the primary contact address, if any.
* @property city The city of the primary contact address.
* @property companyName The name of the company associated with the primary contact information, if any.
* @property countryCode The ISO-3166 two-letter country code for the primary contact address.
* @property districtOrCounty The district or county of the primary contact address, if any.
* @property fullName The full name of the primary contact address.
* @property phoneNumber The phone number of the primary contact information. The number will be validated and, in some countries, checked for activation.
* @property postalCode The postal code of the primary contact address.
* @property stateOrRegion The state or region of the primary contact address. This field is required in selected countries.
* @property websiteUrl The URL of the website associated with the primary contact information, if any.
*/
public data class PrimaryContactArgs(
public val accountId: Output? = null,
public val addressLine1: Output? = null,
public val addressLine2: Output? = null,
public val addressLine3: Output? = null,
public val city: Output? = null,
public val companyName: Output? = null,
public val countryCode: Output? = null,
public val districtOrCounty: Output? = null,
public val fullName: Output? = null,
public val phoneNumber: Output? = null,
public val postalCode: Output? = null,
public val stateOrRegion: Output? = null,
public val websiteUrl: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.account.PrimaryContactArgs =
com.pulumi.aws.account.PrimaryContactArgs.builder()
.accountId(accountId?.applyValue({ args0 -> args0 }))
.addressLine1(addressLine1?.applyValue({ args0 -> args0 }))
.addressLine2(addressLine2?.applyValue({ args0 -> args0 }))
.addressLine3(addressLine3?.applyValue({ args0 -> args0 }))
.city(city?.applyValue({ args0 -> args0 }))
.companyName(companyName?.applyValue({ args0 -> args0 }))
.countryCode(countryCode?.applyValue({ args0 -> args0 }))
.districtOrCounty(districtOrCounty?.applyValue({ args0 -> args0 }))
.fullName(fullName?.applyValue({ args0 -> args0 }))
.phoneNumber(phoneNumber?.applyValue({ args0 -> args0 }))
.postalCode(postalCode?.applyValue({ args0 -> args0 }))
.stateOrRegion(stateOrRegion?.applyValue({ args0 -> args0 }))
.websiteUrl(websiteUrl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PrimaryContactArgs].
*/
@PulumiTagMarker
public class PrimaryContactArgsBuilder internal constructor() {
private var accountId: Output? = null
private var addressLine1: Output? = null
private var addressLine2: Output? = null
private var addressLine3: Output? = null
private var city: Output? = null
private var companyName: Output? = null
private var countryCode: Output? = null
private var districtOrCounty: Output? = null
private var fullName: Output? = null
private var phoneNumber: Output? = null
private var postalCode: Output? = null
private var stateOrRegion: Output? = null
private var websiteUrl: Output? = null
/**
* @param value The ID of the target account when managing member accounts. Will manage current user's account by default if omitted.
*/
@JvmName("xmortjasioauiphd")
public suspend fun accountId(`value`: Output) {
this.accountId = value
}
/**
* @param value The first line of the primary contact address.
*/
@JvmName("ribrwcplctfgefdw")
public suspend fun addressLine1(`value`: Output) {
this.addressLine1 = value
}
/**
* @param value The second line of the primary contact address, if any.
*/
@JvmName("pnrolwcdygxhvccr")
public suspend fun addressLine2(`value`: Output) {
this.addressLine2 = value
}
/**
* @param value The third line of the primary contact address, if any.
*/
@JvmName("xxvyimyatieugyyj")
public suspend fun addressLine3(`value`: Output) {
this.addressLine3 = value
}
/**
* @param value The city of the primary contact address.
*/
@JvmName("fgatxjdcftvgfmsq")
public suspend fun city(`value`: Output) {
this.city = value
}
/**
* @param value The name of the company associated with the primary contact information, if any.
*/
@JvmName("ogdlwuqnmkudjreb")
public suspend fun companyName(`value`: Output) {
this.companyName = value
}
/**
* @param value The ISO-3166 two-letter country code for the primary contact address.
*/
@JvmName("crcexvuekjqenrws")
public suspend fun countryCode(`value`: Output) {
this.countryCode = value
}
/**
* @param value The district or county of the primary contact address, if any.
*/
@JvmName("bwqrcvukwedcrjxc")
public suspend fun districtOrCounty(`value`: Output) {
this.districtOrCounty = value
}
/**
* @param value The full name of the primary contact address.
*/
@JvmName("nwemennmvocxfsix")
public suspend fun fullName(`value`: Output) {
this.fullName = value
}
/**
* @param value The phone number of the primary contact information. The number will be validated and, in some countries, checked for activation.
*/
@JvmName("ukrlrggcgnuawlxo")
public suspend fun phoneNumber(`value`: Output) {
this.phoneNumber = value
}
/**
* @param value The postal code of the primary contact address.
*/
@JvmName("dmxfdkdhtussgvvk")
public suspend fun postalCode(`value`: Output) {
this.postalCode = value
}
/**
* @param value The state or region of the primary contact address. This field is required in selected countries.
*/
@JvmName("yugnmunfcbgfpblo")
public suspend fun stateOrRegion(`value`: Output) {
this.stateOrRegion = value
}
/**
* @param value The URL of the website associated with the primary contact information, if any.
*/
@JvmName("vboigfsjtxbondqp")
public suspend fun websiteUrl(`value`: Output) {
this.websiteUrl = value
}
/**
* @param value The ID of the target account when managing member accounts. Will manage current user's account by default if omitted.
*/
@JvmName("ibrnjjqyhraxcxne")
public suspend fun accountId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accountId = mapped
}
/**
* @param value The first line of the primary contact address.
*/
@JvmName("kwttvixvxixmqlia")
public suspend fun addressLine1(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.addressLine1 = mapped
}
/**
* @param value The second line of the primary contact address, if any.
*/
@JvmName("vcuswnskfrclcdfi")
public suspend fun addressLine2(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.addressLine2 = mapped
}
/**
* @param value The third line of the primary contact address, if any.
*/
@JvmName("enkaxhjokrwbsosu")
public suspend fun addressLine3(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.addressLine3 = mapped
}
/**
* @param value The city of the primary contact address.
*/
@JvmName("giceuojfoxohmtqi")
public suspend fun city(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.city = mapped
}
/**
* @param value The name of the company associated with the primary contact information, if any.
*/
@JvmName("debixjubejmhhxmv")
public suspend fun companyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.companyName = mapped
}
/**
* @param value The ISO-3166 two-letter country code for the primary contact address.
*/
@JvmName("wuritesivrtrdlec")
public suspend fun countryCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.countryCode = mapped
}
/**
* @param value The district or county of the primary contact address, if any.
*/
@JvmName("hixsiwhakbkhpurq")
public suspend fun districtOrCounty(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.districtOrCounty = mapped
}
/**
* @param value The full name of the primary contact address.
*/
@JvmName("qachmxlksuwqurak")
public suspend fun fullName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fullName = mapped
}
/**
* @param value The phone number of the primary contact information. The number will be validated and, in some countries, checked for activation.
*/
@JvmName("vialtsiqmnjsokoa")
public suspend fun phoneNumber(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.phoneNumber = mapped
}
/**
* @param value The postal code of the primary contact address.
*/
@JvmName("lswlujdiflnhhugw")
public suspend fun postalCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postalCode = mapped
}
/**
* @param value The state or region of the primary contact address. This field is required in selected countries.
*/
@JvmName("oiqxnucovlfbgmrw")
public suspend fun stateOrRegion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stateOrRegion = mapped
}
/**
* @param value The URL of the website associated with the primary contact information, if any.
*/
@JvmName("wudscqdqfwurodpf")
public suspend fun websiteUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.websiteUrl = mapped
}
internal fun build(): PrimaryContactArgs = PrimaryContactArgs(
accountId = accountId,
addressLine1 = addressLine1,
addressLine2 = addressLine2,
addressLine3 = addressLine3,
city = city,
companyName = companyName,
countryCode = countryCode,
districtOrCounty = districtOrCounty,
fullName = fullName,
phoneNumber = phoneNumber,
postalCode = postalCode,
stateOrRegion = stateOrRegion,
websiteUrl = websiteUrl,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy