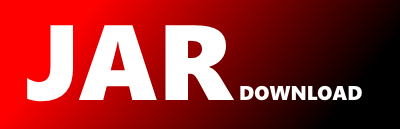
com.pulumi.aws.acmpca.kotlin.PolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.acmpca.kotlin
import com.pulumi.aws.acmpca.PolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Attaches a resource based policy to a private CA.
* ## Example Usage
* ### Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.iam.getPolicyDocument({
* statements: [
* {
* sid: "1",
* effect: "Allow",
* principals: [{
* type: "AWS",
* identifiers: [current.accountId],
* }],
* actions: [
* "acm-pca:DescribeCertificateAuthority",
* "acm-pca:GetCertificate",
* "acm-pca:GetCertificateAuthorityCertificate",
* "acm-pca:ListPermissions",
* "acm-pca:ListTags",
* ],
* resources: [exampleAwsAcmpcaCertificateAuthority.arn],
* },
* {
* sid: "2",
* effect: allow,
* principals: [{
* type: "AWS",
* identifiers: [current.accountId],
* }],
* actions: ["acm-pca:IssueCertificate"],
* resources: [exampleAwsAcmpcaCertificateAuthority.arn],
* conditions: [{
* test: "StringEquals",
* variable: "acm-pca:TemplateArn",
* values: ["arn:aws:acm-pca:::template/EndEntityCertificate/V1"],
* }],
* },
* ],
* });
* const examplePolicy = new aws.acmpca.Policy("example", {
* resourceArn: exampleAwsAcmpcaCertificateAuthority.arn,
* policy: example.then(example => example.json),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.iam.get_policy_document(statements=[
* {
* "sid": "1",
* "effect": "Allow",
* "principals": [{
* "type": "AWS",
* "identifiers": [current["accountId"]],
* }],
* "actions": [
* "acm-pca:DescribeCertificateAuthority",
* "acm-pca:GetCertificate",
* "acm-pca:GetCertificateAuthorityCertificate",
* "acm-pca:ListPermissions",
* "acm-pca:ListTags",
* ],
* "resources": [example_aws_acmpca_certificate_authority["arn"]],
* },
* {
* "sid": "2",
* "effect": allow,
* "principals": [{
* "type": "AWS",
* "identifiers": [current["accountId"]],
* }],
* "actions": ["acm-pca:IssueCertificate"],
* "resources": [example_aws_acmpca_certificate_authority["arn"]],
* "conditions": [{
* "test": "StringEquals",
* "variable": "acm-pca:TemplateArn",
* "values": ["arn:aws:acm-pca:::template/EndEntityCertificate/V1"],
* }],
* },
* ])
* example_policy = aws.acmpca.Policy("example",
* resource_arn=example_aws_acmpca_certificate_authority["arn"],
* policy=example.json)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Sid = "1",
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "AWS",
* Identifiers = new[]
* {
* current.AccountId,
* },
* },
* },
* Actions = new[]
* {
* "acm-pca:DescribeCertificateAuthority",
* "acm-pca:GetCertificate",
* "acm-pca:GetCertificateAuthorityCertificate",
* "acm-pca:ListPermissions",
* "acm-pca:ListTags",
* },
* Resources = new[]
* {
* exampleAwsAcmpcaCertificateAuthority.Arn,
* },
* },
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Sid = "2",
* Effect = allow,
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "AWS",
* Identifiers = new[]
* {
* current.AccountId,
* },
* },
* },
* Actions = new[]
* {
* "acm-pca:IssueCertificate",
* },
* Resources = new[]
* {
* exampleAwsAcmpcaCertificateAuthority.Arn,
* },
* Conditions = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementConditionInputArgs
* {
* Test = "StringEquals",
* Variable = "acm-pca:TemplateArn",
* Values = new[]
* {
* "arn:aws:acm-pca:::template/EndEntityCertificate/V1",
* },
* },
* },
* },
* },
* });
* var examplePolicy = new Aws.Acmpca.Policy("example", new()
* {
* ResourceArn = exampleAwsAcmpcaCertificateAuthority.Arn,
* PolicyDetails = example.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/acmpca"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Sid: pulumi.StringRef("1"),
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "AWS",
* Identifiers: interface{}{
* current.AccountId,
* },
* },
* },
* Actions: []string{
* "acm-pca:DescribeCertificateAuthority",
* "acm-pca:GetCertificate",
* "acm-pca:GetCertificateAuthorityCertificate",
* "acm-pca:ListPermissions",
* "acm-pca:ListTags",
* },
* Resources: interface{}{
* exampleAwsAcmpcaCertificateAuthority.Arn,
* },
* },
* {
* Sid: pulumi.StringRef("2"),
* Effect: pulumi.StringRef(allow),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "AWS",
* Identifiers: interface{}{
* current.AccountId,
* },
* },
* },
* Actions: []string{
* "acm-pca:IssueCertificate",
* },
* Resources: interface{}{
* exampleAwsAcmpcaCertificateAuthority.Arn,
* },
* Conditions: []iam.GetPolicyDocumentStatementCondition{
* {
* Test: "StringEquals",
* Variable: "acm-pca:TemplateArn",
* Values: []string{
* "arn:aws:acm-pca:::template/EndEntityCertificate/V1",
* },
* },
* },
* },
* },
* }, nil);
* if err != nil {
* return err
* }
* _, err = acmpca.NewPolicy(ctx, "example", &acmpca.PolicyArgs{
* ResourceArn: pulumi.Any(exampleAwsAcmpcaCertificateAuthority.Arn),
* Policy: pulumi.String(example.Json),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.acmpca.Policy;
* import com.pulumi.aws.acmpca.PolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(
* GetPolicyDocumentStatementArgs.builder()
* .sid("1")
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("AWS")
* .identifiers(current.accountId())
* .build())
* .actions(
* "acm-pca:DescribeCertificateAuthority",
* "acm-pca:GetCertificate",
* "acm-pca:GetCertificateAuthorityCertificate",
* "acm-pca:ListPermissions",
* "acm-pca:ListTags")
* .resources(exampleAwsAcmpcaCertificateAuthority.arn())
* .build(),
* GetPolicyDocumentStatementArgs.builder()
* .sid("2")
* .effect(allow)
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("AWS")
* .identifiers(current.accountId())
* .build())
* .actions("acm-pca:IssueCertificate")
* .resources(exampleAwsAcmpcaCertificateAuthority.arn())
* .conditions(GetPolicyDocumentStatementConditionArgs.builder()
* .test("StringEquals")
* .variable("acm-pca:TemplateArn")
* .values("arn:aws:acm-pca:::template/EndEntityCertificate/V1")
* .build())
* .build())
* .build());
* var examplePolicy = new Policy("examplePolicy", PolicyArgs.builder()
* .resourceArn(exampleAwsAcmpcaCertificateAuthority.arn())
* .policy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* examplePolicy:
* type: aws:acmpca:Policy
* name: example
* properties:
* resourceArn: ${exampleAwsAcmpcaCertificateAuthority.arn}
* policy: ${example.json}
* variables:
* example:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - sid: '1'
* effect: Allow
* principals:
* - type: AWS
* identifiers:
* - ${current.accountId}
* actions:
* - acm-pca:DescribeCertificateAuthority
* - acm-pca:GetCertificate
* - acm-pca:GetCertificateAuthorityCertificate
* - acm-pca:ListPermissions
* - acm-pca:ListTags
* resources:
* - ${exampleAwsAcmpcaCertificateAuthority.arn}
* - sid: '2'
* effect: ${allow}
* principals:
* - type: AWS
* identifiers:
* - ${current.accountId}
* actions:
* - acm-pca:IssueCertificate
* resources:
* - ${exampleAwsAcmpcaCertificateAuthority.arn}
* conditions:
* - test: StringEquals
* variable: acm-pca:TemplateArn
* values:
* - arn:aws:acm-pca:::template/EndEntityCertificate/V1
* ```
*
* ## Import
* Using `pulumi import`, import `aws_acmpca_policy` using the `resource_arn` value. For example:
* ```sh
* $ pulumi import aws:acmpca/policy:Policy example arn:aws:acm-pca:us-east-1:123456789012:certificate-authority/12345678-1234-1234-1234-123456789012
* ```
* @property policy JSON-formatted IAM policy to attach to the specified private CA resource.
* @property resourceArn ARN of the private CA to associate with the policy.
*/
public data class PolicyArgs(
public val policy: Output? = null,
public val resourceArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.acmpca.PolicyArgs =
com.pulumi.aws.acmpca.PolicyArgs.builder()
.policy(policy?.applyValue({ args0 -> args0 }))
.resourceArn(resourceArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PolicyArgs].
*/
@PulumiTagMarker
public class PolicyArgsBuilder internal constructor() {
private var policy: Output? = null
private var resourceArn: Output? = null
/**
* @param value JSON-formatted IAM policy to attach to the specified private CA resource.
*/
@JvmName("akwnxrponxnriajb")
public suspend fun policy(`value`: Output) {
this.policy = value
}
/**
* @param value ARN of the private CA to associate with the policy.
*/
@JvmName("emogddledirvclcg")
public suspend fun resourceArn(`value`: Output) {
this.resourceArn = value
}
/**
* @param value JSON-formatted IAM policy to attach to the specified private CA resource.
*/
@JvmName("xydpnhuduiueowpp")
public suspend fun policy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policy = mapped
}
/**
* @param value ARN of the private CA to associate with the policy.
*/
@JvmName("bsqlvlfbdjuaxerr")
public suspend fun resourceArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceArn = mapped
}
internal fun build(): PolicyArgs = PolicyArgs(
policy = policy,
resourceArn = resourceArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy