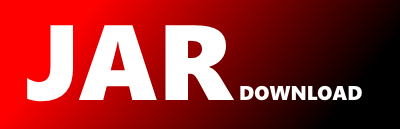
com.pulumi.aws.apigateway.kotlin.inputs.GetSdkPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.apigateway.kotlin.inputs
import com.pulumi.aws.apigateway.inputs.GetSdkPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getSdk.
* @property parameters Key-value map of query string parameters `sdk_type` properties of the SDK. For SDK Type of `objectivec` or `swift`, a parameter named `classPrefix` is required. For SDK Type of `android`, parameters named `groupId`, `artifactId`, `artifactVersion`, and `invokerPackage` are required. For SDK Type of `java`, parameters named `serviceName` and `javaPackageName` are required.
* @property restApiId Identifier of the associated REST API.
* @property sdkType Language for the generated SDK. Currently `java`, `javascript`, `android`, `objectivec` (for iOS), `swift` (for iOS), and `ruby` are supported.
* @property stageName Name of the Stage that will be exported.
*/
public data class GetSdkPlainArgs(
public val parameters: Map? = null,
public val restApiId: String,
public val sdkType: String,
public val stageName: String,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.apigateway.inputs.GetSdkPlainArgs =
com.pulumi.aws.apigateway.inputs.GetSdkPlainArgs.builder()
.parameters(parameters?.let({ args0 -> args0.map({ args0 -> args0.key.to(args0.value) }).toMap() }))
.restApiId(restApiId.let({ args0 -> args0 }))
.sdkType(sdkType.let({ args0 -> args0 }))
.stageName(stageName.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetSdkPlainArgs].
*/
@PulumiTagMarker
public class GetSdkPlainArgsBuilder internal constructor() {
private var parameters: Map? = null
private var restApiId: String? = null
private var sdkType: String? = null
private var stageName: String? = null
/**
* @param value Key-value map of query string parameters `sdk_type` properties of the SDK. For SDK Type of `objectivec` or `swift`, a parameter named `classPrefix` is required. For SDK Type of `android`, parameters named `groupId`, `artifactId`, `artifactVersion`, and `invokerPackage` are required. For SDK Type of `java`, parameters named `serviceName` and `javaPackageName` are required.
*/
@JvmName("ffgrhsdyujamigsl")
public suspend fun parameters(`value`: Map?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.parameters = mapped
}
/**
* @param values Key-value map of query string parameters `sdk_type` properties of the SDK. For SDK Type of `objectivec` or `swift`, a parameter named `classPrefix` is required. For SDK Type of `android`, parameters named `groupId`, `artifactId`, `artifactVersion`, and `invokerPackage` are required. For SDK Type of `java`, parameters named `serviceName` and `javaPackageName` are required.
*/
@JvmName("dbftvsvsgoegtrhi")
public fun parameters(vararg values: Pair) {
val toBeMapped = values.toMap()
val mapped = toBeMapped.let({ args0 -> args0 })
this.parameters = mapped
}
/**
* @param value Identifier of the associated REST API.
*/
@JvmName("toenfftfafdhrcst")
public suspend fun restApiId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.restApiId = mapped
}
/**
* @param value Language for the generated SDK. Currently `java`, `javascript`, `android`, `objectivec` (for iOS), `swift` (for iOS), and `ruby` are supported.
*/
@JvmName("lpawvxciiyidfkuy")
public suspend fun sdkType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.sdkType = mapped
}
/**
* @param value Name of the Stage that will be exported.
*/
@JvmName("ryegtpesqcrvnvba")
public suspend fun stageName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.stageName = mapped
}
internal fun build(): GetSdkPlainArgs = GetSdkPlainArgs(
parameters = parameters,
restApiId = restApiId ?: throw PulumiNullFieldException("restApiId"),
sdkType = sdkType ?: throw PulumiNullFieldException("sdkType"),
stageName = stageName ?: throw PulumiNullFieldException("stageName"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy