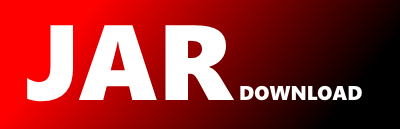
com.pulumi.aws.apigatewayv2.kotlin.AuthorizerArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.apigatewayv2.kotlin
import com.pulumi.aws.apigatewayv2.AuthorizerArgs.builder
import com.pulumi.aws.apigatewayv2.kotlin.inputs.AuthorizerJwtConfigurationArgs
import com.pulumi.aws.apigatewayv2.kotlin.inputs.AuthorizerJwtConfigurationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages an Amazon API Gateway Version 2 authorizer.
* More information can be found in the [Amazon API Gateway Developer Guide](https://docs.aws.amazon.com/apigateway/latest/developerguide/apigateway-websocket-api.html).
* ## Example Usage
* ### Basic WebSocket API
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.apigatewayv2.Authorizer("example", {
* apiId: exampleAwsApigatewayv2Api.id,
* authorizerType: "REQUEST",
* authorizerUri: exampleAwsLambdaFunction.invokeArn,
* identitySources: ["route.request.header.Auth"],
* name: "example-authorizer",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.apigatewayv2.Authorizer("example",
* api_id=example_aws_apigatewayv2_api["id"],
* authorizer_type="REQUEST",
* authorizer_uri=example_aws_lambda_function["invokeArn"],
* identity_sources=["route.request.header.Auth"],
* name="example-authorizer")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.ApiGatewayV2.Authorizer("example", new()
* {
* ApiId = exampleAwsApigatewayv2Api.Id,
* AuthorizerType = "REQUEST",
* AuthorizerUri = exampleAwsLambdaFunction.InvokeArn,
* IdentitySources = new[]
* {
* "route.request.header.Auth",
* },
* Name = "example-authorizer",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/apigatewayv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := apigatewayv2.NewAuthorizer(ctx, "example", &apigatewayv2.AuthorizerArgs{
* ApiId: pulumi.Any(exampleAwsApigatewayv2Api.Id),
* AuthorizerType: pulumi.String("REQUEST"),
* AuthorizerUri: pulumi.Any(exampleAwsLambdaFunction.InvokeArn),
* IdentitySources: pulumi.StringArray{
* pulumi.String("route.request.header.Auth"),
* },
* Name: pulumi.String("example-authorizer"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigatewayv2.Authorizer;
* import com.pulumi.aws.apigatewayv2.AuthorizerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Authorizer("example", AuthorizerArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .authorizerType("REQUEST")
* .authorizerUri(exampleAwsLambdaFunction.invokeArn())
* .identitySources("route.request.header.Auth")
* .name("example-authorizer")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:apigatewayv2:Authorizer
* properties:
* apiId: ${exampleAwsApigatewayv2Api.id}
* authorizerType: REQUEST
* authorizerUri: ${exampleAwsLambdaFunction.invokeArn}
* identitySources:
* - route.request.header.Auth
* name: example-authorizer
* ```
*
* ### Basic HTTP API
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.apigatewayv2.Authorizer("example", {
* apiId: exampleAwsApigatewayv2Api.id,
* authorizerType: "REQUEST",
* authorizerUri: exampleAwsLambdaFunction.invokeArn,
* identitySources: ["$request.header.Authorization"],
* name: "example-authorizer",
* authorizerPayloadFormatVersion: "2.0",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.apigatewayv2.Authorizer("example",
* api_id=example_aws_apigatewayv2_api["id"],
* authorizer_type="REQUEST",
* authorizer_uri=example_aws_lambda_function["invokeArn"],
* identity_sources=["$request.header.Authorization"],
* name="example-authorizer",
* authorizer_payload_format_version="2.0")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.ApiGatewayV2.Authorizer("example", new()
* {
* ApiId = exampleAwsApigatewayv2Api.Id,
* AuthorizerType = "REQUEST",
* AuthorizerUri = exampleAwsLambdaFunction.InvokeArn,
* IdentitySources = new[]
* {
* "$request.header.Authorization",
* },
* Name = "example-authorizer",
* AuthorizerPayloadFormatVersion = "2.0",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/apigatewayv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := apigatewayv2.NewAuthorizer(ctx, "example", &apigatewayv2.AuthorizerArgs{
* ApiId: pulumi.Any(exampleAwsApigatewayv2Api.Id),
* AuthorizerType: pulumi.String("REQUEST"),
* AuthorizerUri: pulumi.Any(exampleAwsLambdaFunction.InvokeArn),
* IdentitySources: pulumi.StringArray{
* pulumi.String("$request.header.Authorization"),
* },
* Name: pulumi.String("example-authorizer"),
* AuthorizerPayloadFormatVersion: pulumi.String("2.0"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigatewayv2.Authorizer;
* import com.pulumi.aws.apigatewayv2.AuthorizerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Authorizer("example", AuthorizerArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .authorizerType("REQUEST")
* .authorizerUri(exampleAwsLambdaFunction.invokeArn())
* .identitySources("$request.header.Authorization")
* .name("example-authorizer")
* .authorizerPayloadFormatVersion("2.0")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:apigatewayv2:Authorizer
* properties:
* apiId: ${exampleAwsApigatewayv2Api.id}
* authorizerType: REQUEST
* authorizerUri: ${exampleAwsLambdaFunction.invokeArn}
* identitySources:
* - $request.header.Authorization
* name: example-authorizer
* authorizerPayloadFormatVersion: '2.0'
* ```
*
* ## Import
* Using `pulumi import`, import `aws_apigatewayv2_authorizer` using the API identifier and authorizer identifier. For example:
* ```sh
* $ pulumi import aws:apigatewayv2/authorizer:Authorizer example aabbccddee/1122334
* ```
* @property apiId API identifier.
* @property authorizerCredentialsArn Required credentials as an IAM role for API Gateway to invoke the authorizer.
* Supported only for `REQUEST` authorizers.
* @property authorizerPayloadFormatVersion Format of the payload sent to an HTTP API Lambda authorizer. Required for HTTP API Lambda authorizers.
* Valid values: `1.0`, `2.0`.
* @property authorizerResultTtlInSeconds Time to live (TTL) for cached authorizer results, in seconds. If it equals 0, authorization caching is disabled.
* If it is greater than 0, API Gateway caches authorizer responses. The maximum value is 3600, or 1 hour. Defaults to `300`.
* Supported only for HTTP API Lambda authorizers.
* @property authorizerType Authorizer type. Valid values: `JWT`, `REQUEST`.
* Specify `REQUEST` for a Lambda function using incoming request parameters.
* For HTTP APIs, specify `JWT` to use JSON Web Tokens.
* @property authorizerUri Authorizer's Uniform Resource Identifier (URI).
* For `REQUEST` authorizers this must be a well-formed Lambda function URI, such as the `invoke_arn` attribute of the `aws.lambda.Function` resource.
* Supported only for `REQUEST` authorizers. Must be between 1 and 2048 characters in length.
* @property enableSimpleResponses Whether a Lambda authorizer returns a response in a simple format. If enabled, the Lambda authorizer can return a boolean value instead of an IAM policy.
* Supported only for HTTP APIs.
* @property identitySources Identity sources for which authorization is requested.
* For `REQUEST` authorizers the value is a list of one or more mapping expressions of the specified request parameters.
* For `JWT` authorizers the single entry specifies where to extract the JSON Web Token (JWT) from inbound requests.
* @property jwtConfiguration Configuration of a JWT authorizer. Required for the `JWT` authorizer type.
* Supported only for HTTP APIs.
* @property name Name of the authorizer. Must be between 1 and 128 characters in length.
*/
public data class AuthorizerArgs(
public val apiId: Output? = null,
public val authorizerCredentialsArn: Output? = null,
public val authorizerPayloadFormatVersion: Output? = null,
public val authorizerResultTtlInSeconds: Output? = null,
public val authorizerType: Output? = null,
public val authorizerUri: Output? = null,
public val enableSimpleResponses: Output? = null,
public val identitySources: Output>? = null,
public val jwtConfiguration: Output? = null,
public val name: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.apigatewayv2.AuthorizerArgs =
com.pulumi.aws.apigatewayv2.AuthorizerArgs.builder()
.apiId(apiId?.applyValue({ args0 -> args0 }))
.authorizerCredentialsArn(authorizerCredentialsArn?.applyValue({ args0 -> args0 }))
.authorizerPayloadFormatVersion(authorizerPayloadFormatVersion?.applyValue({ args0 -> args0 }))
.authorizerResultTtlInSeconds(authorizerResultTtlInSeconds?.applyValue({ args0 -> args0 }))
.authorizerType(authorizerType?.applyValue({ args0 -> args0 }))
.authorizerUri(authorizerUri?.applyValue({ args0 -> args0 }))
.enableSimpleResponses(enableSimpleResponses?.applyValue({ args0 -> args0 }))
.identitySources(identitySources?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.jwtConfiguration(jwtConfiguration?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AuthorizerArgs].
*/
@PulumiTagMarker
public class AuthorizerArgsBuilder internal constructor() {
private var apiId: Output? = null
private var authorizerCredentialsArn: Output? = null
private var authorizerPayloadFormatVersion: Output? = null
private var authorizerResultTtlInSeconds: Output? = null
private var authorizerType: Output? = null
private var authorizerUri: Output? = null
private var enableSimpleResponses: Output? = null
private var identitySources: Output>? = null
private var jwtConfiguration: Output? = null
private var name: Output? = null
/**
* @param value API identifier.
*/
@JvmName("ydoqvhygxvnliwhw")
public suspend fun apiId(`value`: Output) {
this.apiId = value
}
/**
* @param value Required credentials as an IAM role for API Gateway to invoke the authorizer.
* Supported only for `REQUEST` authorizers.
*/
@JvmName("rlcmvpqgpfqkycjw")
public suspend fun authorizerCredentialsArn(`value`: Output) {
this.authorizerCredentialsArn = value
}
/**
* @param value Format of the payload sent to an HTTP API Lambda authorizer. Required for HTTP API Lambda authorizers.
* Valid values: `1.0`, `2.0`.
*/
@JvmName("dmtrrwhpulbshybm")
public suspend fun authorizerPayloadFormatVersion(`value`: Output) {
this.authorizerPayloadFormatVersion = value
}
/**
* @param value Time to live (TTL) for cached authorizer results, in seconds. If it equals 0, authorization caching is disabled.
* If it is greater than 0, API Gateway caches authorizer responses. The maximum value is 3600, or 1 hour. Defaults to `300`.
* Supported only for HTTP API Lambda authorizers.
*/
@JvmName("uifijikipxaqqvyw")
public suspend fun authorizerResultTtlInSeconds(`value`: Output) {
this.authorizerResultTtlInSeconds = value
}
/**
* @param value Authorizer type. Valid values: `JWT`, `REQUEST`.
* Specify `REQUEST` for a Lambda function using incoming request parameters.
* For HTTP APIs, specify `JWT` to use JSON Web Tokens.
*/
@JvmName("xkreuqrgbecqqdlr")
public suspend fun authorizerType(`value`: Output) {
this.authorizerType = value
}
/**
* @param value Authorizer's Uniform Resource Identifier (URI).
* For `REQUEST` authorizers this must be a well-formed Lambda function URI, such as the `invoke_arn` attribute of the `aws.lambda.Function` resource.
* Supported only for `REQUEST` authorizers. Must be between 1 and 2048 characters in length.
*/
@JvmName("lkdtojiivwswmkes")
public suspend fun authorizerUri(`value`: Output) {
this.authorizerUri = value
}
/**
* @param value Whether a Lambda authorizer returns a response in a simple format. If enabled, the Lambda authorizer can return a boolean value instead of an IAM policy.
* Supported only for HTTP APIs.
*/
@JvmName("veobyervcvgavnbv")
public suspend fun enableSimpleResponses(`value`: Output) {
this.enableSimpleResponses = value
}
/**
* @param value Identity sources for which authorization is requested.
* For `REQUEST` authorizers the value is a list of one or more mapping expressions of the specified request parameters.
* For `JWT` authorizers the single entry specifies where to extract the JSON Web Token (JWT) from inbound requests.
*/
@JvmName("grbifcqkguklggvu")
public suspend fun identitySources(`value`: Output>) {
this.identitySources = value
}
@JvmName("namsagwemrpphjkh")
public suspend fun identitySources(vararg values: Output) {
this.identitySources = Output.all(values.asList())
}
/**
* @param values Identity sources for which authorization is requested.
* For `REQUEST` authorizers the value is a list of one or more mapping expressions of the specified request parameters.
* For `JWT` authorizers the single entry specifies where to extract the JSON Web Token (JWT) from inbound requests.
*/
@JvmName("oamitoapdcluyrbl")
public suspend fun identitySources(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy