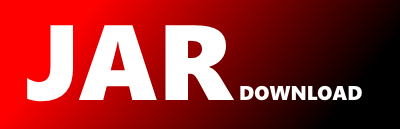
com.pulumi.aws.apigatewayv2.kotlin.Integration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.apigatewayv2.kotlin
import com.pulumi.aws.apigatewayv2.kotlin.outputs.IntegrationResponseParameter
import com.pulumi.aws.apigatewayv2.kotlin.outputs.IntegrationTlsConfig
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.apigatewayv2.kotlin.outputs.IntegrationResponseParameter.Companion.toKotlin as integrationResponseParameterToKotlin
import com.pulumi.aws.apigatewayv2.kotlin.outputs.IntegrationTlsConfig.Companion.toKotlin as integrationTlsConfigToKotlin
/**
* Builder for [Integration].
*/
@PulumiTagMarker
public class IntegrationResourceBuilder internal constructor() {
public var name: String? = null
public var args: IntegrationArgs = IntegrationArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend IntegrationArgsBuilder.() -> Unit) {
val builder = IntegrationArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Integration {
val builtJavaResource = com.pulumi.aws.apigatewayv2.Integration(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Integration(builtJavaResource)
}
}
/**
* Manages an Amazon API Gateway Version 2 integration.
* More information can be found in the [Amazon API Gateway Developer Guide](https://docs.aws.amazon.com/apigateway/latest/developerguide/apigateway-websocket-api.html).
* ## Example Usage
* ### Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.apigatewayv2.Integration("example", {
* apiId: exampleAwsApigatewayv2Api.id,
* integrationType: "MOCK",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.apigatewayv2.Integration("example",
* api_id=example_aws_apigatewayv2_api["id"],
* integration_type="MOCK")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.ApiGatewayV2.Integration("example", new()
* {
* ApiId = exampleAwsApigatewayv2Api.Id,
* IntegrationType = "MOCK",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/apigatewayv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := apigatewayv2.NewIntegration(ctx, "example", &apigatewayv2.IntegrationArgs{
* ApiId: pulumi.Any(exampleAwsApigatewayv2Api.Id),
* IntegrationType: pulumi.String("MOCK"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigatewayv2.Integration;
* import com.pulumi.aws.apigatewayv2.IntegrationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Integration("example", IntegrationArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .integrationType("MOCK")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:apigatewayv2:Integration
* properties:
* apiId: ${exampleAwsApigatewayv2Api.id}
* integrationType: MOCK
* ```
*
* ### Lambda Integration
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.lambda.Function("example", {
* code: new pulumi.asset.FileArchive("example.zip"),
* name: "Example",
* role: exampleAwsIamRole.arn,
* handler: "index.handler",
* runtime: aws.lambda.Runtime.NodeJS20dX,
* });
* const exampleIntegration = new aws.apigatewayv2.Integration("example", {
* apiId: exampleAwsApigatewayv2Api.id,
* integrationType: "AWS_PROXY",
* connectionType: "INTERNET",
* contentHandlingStrategy: "CONVERT_TO_TEXT",
* description: "Lambda example",
* integrationMethod: "POST",
* integrationUri: example.invokeArn,
* passthroughBehavior: "WHEN_NO_MATCH",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.lambda_.Function("example",
* code=pulumi.FileArchive("example.zip"),
* name="Example",
* role=example_aws_iam_role["arn"],
* handler="index.handler",
* runtime=aws.lambda_.Runtime.NODE_JS20D_X)
* example_integration = aws.apigatewayv2.Integration("example",
* api_id=example_aws_apigatewayv2_api["id"],
* integration_type="AWS_PROXY",
* connection_type="INTERNET",
* content_handling_strategy="CONVERT_TO_TEXT",
* description="Lambda example",
* integration_method="POST",
* integration_uri=example.invoke_arn,
* passthrough_behavior="WHEN_NO_MATCH")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Lambda.Function("example", new()
* {
* Code = new FileArchive("example.zip"),
* Name = "Example",
* Role = exampleAwsIamRole.Arn,
* Handler = "index.handler",
* Runtime = Aws.Lambda.Runtime.NodeJS20dX,
* });
* var exampleIntegration = new Aws.ApiGatewayV2.Integration("example", new()
* {
* ApiId = exampleAwsApigatewayv2Api.Id,
* IntegrationType = "AWS_PROXY",
* ConnectionType = "INTERNET",
* ContentHandlingStrategy = "CONVERT_TO_TEXT",
* Description = "Lambda example",
* IntegrationMethod = "POST",
* IntegrationUri = example.InvokeArn,
* PassthroughBehavior = "WHEN_NO_MATCH",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/apigatewayv2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/lambda"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := lambda.NewFunction(ctx, "example", &lambda.FunctionArgs{
* Code: pulumi.NewFileArchive("example.zip"),
* Name: pulumi.String("Example"),
* Role: pulumi.Any(exampleAwsIamRole.Arn),
* Handler: pulumi.String("index.handler"),
* Runtime: pulumi.String(lambda.RuntimeNodeJS20dX),
* })
* if err != nil {
* return err
* }
* _, err = apigatewayv2.NewIntegration(ctx, "example", &apigatewayv2.IntegrationArgs{
* ApiId: pulumi.Any(exampleAwsApigatewayv2Api.Id),
* IntegrationType: pulumi.String("AWS_PROXY"),
* ConnectionType: pulumi.String("INTERNET"),
* ContentHandlingStrategy: pulumi.String("CONVERT_TO_TEXT"),
* Description: pulumi.String("Lambda example"),
* IntegrationMethod: pulumi.String("POST"),
* IntegrationUri: example.InvokeArn,
* PassthroughBehavior: pulumi.String("WHEN_NO_MATCH"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lambda.Function;
* import com.pulumi.aws.lambda.FunctionArgs;
* import com.pulumi.aws.apigatewayv2.Integration;
* import com.pulumi.aws.apigatewayv2.IntegrationArgs;
* import com.pulumi.asset.FileArchive;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Function("example", FunctionArgs.builder()
* .code(new FileArchive("example.zip"))
* .name("Example")
* .role(exampleAwsIamRole.arn())
* .handler("index.handler")
* .runtime("nodejs20.x")
* .build());
* var exampleIntegration = new Integration("exampleIntegration", IntegrationArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .integrationType("AWS_PROXY")
* .connectionType("INTERNET")
* .contentHandlingStrategy("CONVERT_TO_TEXT")
* .description("Lambda example")
* .integrationMethod("POST")
* .integrationUri(example.invokeArn())
* .passthroughBehavior("WHEN_NO_MATCH")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:lambda:Function
* properties:
* code:
* fn::FileArchive: example.zip
* name: Example
* role: ${exampleAwsIamRole.arn}
* handler: index.handler
* runtime: nodejs20.x
* exampleIntegration:
* type: aws:apigatewayv2:Integration
* name: example
* properties:
* apiId: ${exampleAwsApigatewayv2Api.id}
* integrationType: AWS_PROXY
* connectionType: INTERNET
* contentHandlingStrategy: CONVERT_TO_TEXT
* description: Lambda example
* integrationMethod: POST
* integrationUri: ${example.invokeArn}
* passthroughBehavior: WHEN_NO_MATCH
* ```
*
* ### AWS Service Integration
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.apigatewayv2.Integration("example", {
* apiId: exampleAwsApigatewayv2Api.id,
* credentialsArn: exampleAwsIamRole.arn,
* description: "SQS example",
* integrationType: "AWS_PROXY",
* integrationSubtype: "SQS-SendMessage",
* requestParameters: {
* QueueUrl: "$request.header.queueUrl",
* MessageBody: "$request.body.message",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.apigatewayv2.Integration("example",
* api_id=example_aws_apigatewayv2_api["id"],
* credentials_arn=example_aws_iam_role["arn"],
* description="SQS example",
* integration_type="AWS_PROXY",
* integration_subtype="SQS-SendMessage",
* request_parameters={
* "QueueUrl": "$request.header.queueUrl",
* "MessageBody": "$request.body.message",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.ApiGatewayV2.Integration("example", new()
* {
* ApiId = exampleAwsApigatewayv2Api.Id,
* CredentialsArn = exampleAwsIamRole.Arn,
* Description = "SQS example",
* IntegrationType = "AWS_PROXY",
* IntegrationSubtype = "SQS-SendMessage",
* RequestParameters =
* {
* { "QueueUrl", "$request.header.queueUrl" },
* { "MessageBody", "$request.body.message" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/apigatewayv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := apigatewayv2.NewIntegration(ctx, "example", &apigatewayv2.IntegrationArgs{
* ApiId: pulumi.Any(exampleAwsApigatewayv2Api.Id),
* CredentialsArn: pulumi.Any(exampleAwsIamRole.Arn),
* Description: pulumi.String("SQS example"),
* IntegrationType: pulumi.String("AWS_PROXY"),
* IntegrationSubtype: pulumi.String("SQS-SendMessage"),
* RequestParameters: pulumi.StringMap{
* "QueueUrl": pulumi.String("$request.header.queueUrl"),
* "MessageBody": pulumi.String("$request.body.message"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigatewayv2.Integration;
* import com.pulumi.aws.apigatewayv2.IntegrationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Integration("example", IntegrationArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .credentialsArn(exampleAwsIamRole.arn())
* .description("SQS example")
* .integrationType("AWS_PROXY")
* .integrationSubtype("SQS-SendMessage")
* .requestParameters(Map.ofEntries(
* Map.entry("QueueUrl", "$request.header.queueUrl"),
* Map.entry("MessageBody", "$request.body.message")
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:apigatewayv2:Integration
* properties:
* apiId: ${exampleAwsApigatewayv2Api.id}
* credentialsArn: ${exampleAwsIamRole.arn}
* description: SQS example
* integrationType: AWS_PROXY
* integrationSubtype: SQS-SendMessage
* requestParameters:
* QueueUrl: $request.header.queueUrl
* MessageBody: $request.body.message
* ```
*
* ### Private Integration
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.apigatewayv2.Integration("example", {
* apiId: exampleAwsApigatewayv2Api.id,
* credentialsArn: exampleAwsIamRole.arn,
* description: "Example with a load balancer",
* integrationType: "HTTP_PROXY",
* integrationUri: exampleAwsLbListener.arn,
* integrationMethod: "ANY",
* connectionType: "VPC_LINK",
* connectionId: exampleAwsApigatewayv2VpcLink.id,
* tlsConfig: {
* serverNameToVerify: "example.com",
* },
* requestParameters: {
* "append:header.authforintegration": "$context.authorizer.authorizerResponse",
* "overwrite:path": "staticValueForIntegration",
* },
* responseParameters: [
* {
* statusCode: "403",
* mappings: {
* "append:header.auth": "$context.authorizer.authorizerResponse",
* },
* },
* {
* statusCode: "200",
* mappings: {
* "overwrite:statuscode": "204",
* },
* },
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.apigatewayv2.Integration("example",
* api_id=example_aws_apigatewayv2_api["id"],
* credentials_arn=example_aws_iam_role["arn"],
* description="Example with a load balancer",
* integration_type="HTTP_PROXY",
* integration_uri=example_aws_lb_listener["arn"],
* integration_method="ANY",
* connection_type="VPC_LINK",
* connection_id=example_aws_apigatewayv2_vpc_link["id"],
* tls_config={
* "server_name_to_verify": "example.com",
* },
* request_parameters={
* "append:header.authforintegration": "$context.authorizer.authorizerResponse",
* "overwrite:path": "staticValueForIntegration",
* },
* response_parameters=[
* {
* "status_code": "403",
* "mappings": {
* "append:header.auth": "$context.authorizer.authorizerResponse",
* },
* },
* {
* "status_code": "200",
* "mappings": {
* "overwrite:statuscode": "204",
* },
* },
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.ApiGatewayV2.Integration("example", new()
* {
* ApiId = exampleAwsApigatewayv2Api.Id,
* CredentialsArn = exampleAwsIamRole.Arn,
* Description = "Example with a load balancer",
* IntegrationType = "HTTP_PROXY",
* IntegrationUri = exampleAwsLbListener.Arn,
* IntegrationMethod = "ANY",
* ConnectionType = "VPC_LINK",
* ConnectionId = exampleAwsApigatewayv2VpcLink.Id,
* TlsConfig = new Aws.ApiGatewayV2.Inputs.IntegrationTlsConfigArgs
* {
* ServerNameToVerify = "example.com",
* },
* RequestParameters =
* {
* { "append:header.authforintegration", "$context.authorizer.authorizerResponse" },
* { "overwrite:path", "staticValueForIntegration" },
* },
* ResponseParameters = new[]
* {
* new Aws.ApiGatewayV2.Inputs.IntegrationResponseParameterArgs
* {
* StatusCode = "403",
* Mappings =
* {
* { "append:header.auth", "$context.authorizer.authorizerResponse" },
* },
* },
* new Aws.ApiGatewayV2.Inputs.IntegrationResponseParameterArgs
* {
* StatusCode = "200",
* Mappings =
* {
* { "overwrite:statuscode", "204" },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/apigatewayv2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := apigatewayv2.NewIntegration(ctx, "example", &apigatewayv2.IntegrationArgs{
* ApiId: pulumi.Any(exampleAwsApigatewayv2Api.Id),
* CredentialsArn: pulumi.Any(exampleAwsIamRole.Arn),
* Description: pulumi.String("Example with a load balancer"),
* IntegrationType: pulumi.String("HTTP_PROXY"),
* IntegrationUri: pulumi.Any(exampleAwsLbListener.Arn),
* IntegrationMethod: pulumi.String("ANY"),
* ConnectionType: pulumi.String("VPC_LINK"),
* ConnectionId: pulumi.Any(exampleAwsApigatewayv2VpcLink.Id),
* TlsConfig: &apigatewayv2.IntegrationTlsConfigArgs{
* ServerNameToVerify: pulumi.String("example.com"),
* },
* RequestParameters: pulumi.StringMap{
* "append:header.authforintegration": pulumi.String("$context.authorizer.authorizerResponse"),
* "overwrite:path": pulumi.String("staticValueForIntegration"),
* },
* ResponseParameters: apigatewayv2.IntegrationResponseParameterArray{
* &apigatewayv2.IntegrationResponseParameterArgs{
* StatusCode: pulumi.String("403"),
* Mappings: pulumi.StringMap{
* "append:header.auth": pulumi.String("$context.authorizer.authorizerResponse"),
* },
* },
* &apigatewayv2.IntegrationResponseParameterArgs{
* StatusCode: pulumi.String("200"),
* Mappings: pulumi.StringMap{
* "overwrite:statuscode": pulumi.String("204"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apigatewayv2.Integration;
* import com.pulumi.aws.apigatewayv2.IntegrationArgs;
* import com.pulumi.aws.apigatewayv2.inputs.IntegrationTlsConfigArgs;
* import com.pulumi.aws.apigatewayv2.inputs.IntegrationResponseParameterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Integration("example", IntegrationArgs.builder()
* .apiId(exampleAwsApigatewayv2Api.id())
* .credentialsArn(exampleAwsIamRole.arn())
* .description("Example with a load balancer")
* .integrationType("HTTP_PROXY")
* .integrationUri(exampleAwsLbListener.arn())
* .integrationMethod("ANY")
* .connectionType("VPC_LINK")
* .connectionId(exampleAwsApigatewayv2VpcLink.id())
* .tlsConfig(IntegrationTlsConfigArgs.builder()
* .serverNameToVerify("example.com")
* .build())
* .requestParameters(Map.ofEntries(
* Map.entry("append:header.authforintegration", "$context.authorizer.authorizerResponse"),
* Map.entry("overwrite:path", "staticValueForIntegration")
* ))
* .responseParameters(
* IntegrationResponseParameterArgs.builder()
* .statusCode(403)
* .mappings(Map.of("append:header.auth", "$context.authorizer.authorizerResponse"))
* .build(),
* IntegrationResponseParameterArgs.builder()
* .statusCode(200)
* .mappings(Map.of("overwrite:statuscode", "204"))
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:apigatewayv2:Integration
* properties:
* apiId: ${exampleAwsApigatewayv2Api.id}
* credentialsArn: ${exampleAwsIamRole.arn}
* description: Example with a load balancer
* integrationType: HTTP_PROXY
* integrationUri: ${exampleAwsLbListener.arn}
* integrationMethod: ANY
* connectionType: VPC_LINK
* connectionId: ${exampleAwsApigatewayv2VpcLink.id}
* tlsConfig:
* serverNameToVerify: example.com
* requestParameters:
* append:header.authforintegration: $context.authorizer.authorizerResponse
* overwrite:path: staticValueForIntegration
* responseParameters:
* - statusCode: 403
* mappings:
* append:header.auth: $context.authorizer.authorizerResponse
* - statusCode: 200
* mappings:
* overwrite:statuscode: '204'
* ```
*
* ## Import
* Using `pulumi import`, import `aws_apigatewayv2_integration` using the API identifier and integration identifier. For example:
* ```sh
* $ pulumi import aws:apigatewayv2/integration:Integration example aabbccddee/1122334
* ```
* -> __Note:__ The API Gateway managed integration created as part of [_quick_create_](https://docs.aws.amazon.com/apigateway/latest/developerguide/api-gateway-basic-concept.html#apigateway-definition-quick-create) cannot be imported.
*/
public class Integration internal constructor(
override val javaResource: com.pulumi.aws.apigatewayv2.Integration,
) : KotlinCustomResource(javaResource, IntegrationMapper) {
/**
* API identifier.
*/
public val apiId: Output
get() = javaResource.apiId().applyValue({ args0 -> args0 })
/**
* ID of the VPC link for a private integration. Supported only for HTTP APIs. Must be between 1 and 1024 characters in length.
*/
public val connectionId: Output?
get() = javaResource.connectionId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Type of the network connection to the integration endpoint. Valid values: `INTERNET`, `VPC_LINK`. Default is `INTERNET`.
*/
public val connectionType: Output?
get() = javaResource.connectionType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* How to handle response payload content type conversions. Valid values: `CONVERT_TO_BINARY`, `CONVERT_TO_TEXT`. Supported only for WebSocket APIs.
*/
public val contentHandlingStrategy: Output?
get() = javaResource.contentHandlingStrategy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Credentials required for the integration, if any.
*/
public val credentialsArn: Output?
get() = javaResource.credentialsArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Description of the integration.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Integration's HTTP method. Must be specified if `integration_type` is not `MOCK`.
*/
public val integrationMethod: Output?
get() = javaResource.integrationMethod().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The [integration response selection expression](https://docs.aws.amazon.com/apigateway/latest/developerguide/apigateway-websocket-api-selection-expressions.html#apigateway-websocket-api-integration-response-selection-expressions) for the integration.
*/
public val integrationResponseSelectionExpression: Output
get() = javaResource.integrationResponseSelectionExpression().applyValue({ args0 -> args0 })
/**
* AWS service action to invoke. Supported only for HTTP APIs when `integration_type` is `AWS_PROXY`. See the [AWS service integration reference](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-aws-services-reference.html) documentation for supported values. Must be between 1 and 128 characters in length.
*/
public val integrationSubtype: Output?
get() = javaResource.integrationSubtype().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Integration type of an integration.
* Valid values: `AWS` (supported only for WebSocket APIs), `AWS_PROXY`, `HTTP` (supported only for WebSocket APIs), `HTTP_PROXY`, `MOCK` (supported only for WebSocket APIs). For an HTTP API private integration, use `HTTP_PROXY`.
*/
public val integrationType: Output
get() = javaResource.integrationType().applyValue({ args0 -> args0 })
/**
* URI of the Lambda function for a Lambda proxy integration, when `integration_type` is `AWS_PROXY`.
* For an `HTTP` integration, specify a fully-qualified URL. For an HTTP API private integration, specify the ARN of an Application Load Balancer listener, Network Load Balancer listener, or AWS Cloud Map service.
*/
public val integrationUri: Output?
get() = javaResource.integrationUri().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Pass-through behavior for incoming requests based on the Content-Type header in the request, and the available mapping templates specified as the `request_templates` attribute.
* Valid values: `WHEN_NO_MATCH`, `WHEN_NO_TEMPLATES`, `NEVER`. Default is `WHEN_NO_MATCH`. Supported only for WebSocket APIs.
*/
public val passthroughBehavior: Output?
get() = javaResource.passthroughBehavior().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The [format of the payload](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-develop-integrations-lambda.html#http-api-develop-integrations-lambda.proxy-format) sent to an integration. Valid values: `1.0`, `2.0`. Default is `1.0`.
*/
public val payloadFormatVersion: Output?
get() = javaResource.payloadFormatVersion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* For WebSocket APIs, a key-value map specifying request parameters that are passed from the method request to the backend.
* For HTTP APIs with a specified `integration_subtype`, a key-value map specifying parameters that are passed to `AWS_PROXY` integrations.
* For HTTP APIs without a specified `integration_subtype`, a key-value map specifying how to transform HTTP requests before sending them to the backend.
* See the [Amazon API Gateway Developer Guide](https://docs.aws.amazon.com/apigateway/latest/developerguide/http-api-parameter-mapping.html) for details.
*/
public val requestParameters: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy