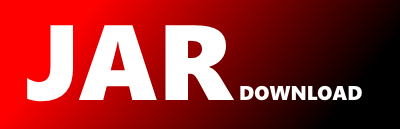
com.pulumi.aws.apigatewayv2.kotlin.inputs.GetExportPlainArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.apigatewayv2.kotlin.inputs
import com.pulumi.aws.apigatewayv2.inputs.GetExportPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getExport.
* @property apiId API identifier.
* @property exportVersion Version of the API Gateway export algorithm. API Gateway uses the latest version by default. Currently, the only supported version is `1.0`.
* @property includeExtensions Whether to include API Gateway extensions in the exported API definition. API Gateway extensions are included by default.
* @property outputType Output type of the exported definition file. Valid values are `JSON` and `YAML`.
* @property specification Version of the API specification to use. `OAS30`, for OpenAPI 3.0, is the only supported value.
* @property stageName Name of the API stage to export. If you don't specify this property, a representation of the latest API configuration is exported.
*/
public data class GetExportPlainArgs(
public val apiId: String,
public val exportVersion: String? = null,
public val includeExtensions: Boolean? = null,
public val outputType: String,
public val specification: String,
public val stageName: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.apigatewayv2.inputs.GetExportPlainArgs =
com.pulumi.aws.apigatewayv2.inputs.GetExportPlainArgs.builder()
.apiId(apiId.let({ args0 -> args0 }))
.exportVersion(exportVersion?.let({ args0 -> args0 }))
.includeExtensions(includeExtensions?.let({ args0 -> args0 }))
.outputType(outputType.let({ args0 -> args0 }))
.specification(specification.let({ args0 -> args0 }))
.stageName(stageName?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetExportPlainArgs].
*/
@PulumiTagMarker
public class GetExportPlainArgsBuilder internal constructor() {
private var apiId: String? = null
private var exportVersion: String? = null
private var includeExtensions: Boolean? = null
private var outputType: String? = null
private var specification: String? = null
private var stageName: String? = null
/**
* @param value API identifier.
*/
@JvmName("sxidoovssltjhchb")
public suspend fun apiId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.apiId = mapped
}
/**
* @param value Version of the API Gateway export algorithm. API Gateway uses the latest version by default. Currently, the only supported version is `1.0`.
*/
@JvmName("mapuvcgtpjnjafhp")
public suspend fun exportVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.exportVersion = mapped
}
/**
* @param value Whether to include API Gateway extensions in the exported API definition. API Gateway extensions are included by default.
*/
@JvmName("xawowxjegnrrpaao")
public suspend fun includeExtensions(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.includeExtensions = mapped
}
/**
* @param value Output type of the exported definition file. Valid values are `JSON` and `YAML`.
*/
@JvmName("doheloxmnhghhhga")
public suspend fun outputType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.outputType = mapped
}
/**
* @param value Version of the API specification to use. `OAS30`, for OpenAPI 3.0, is the only supported value.
*/
@JvmName("yendrowuxjofauyh")
public suspend fun specification(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.specification = mapped
}
/**
* @param value Name of the API stage to export. If you don't specify this property, a representation of the latest API configuration is exported.
*/
@JvmName("otwhabqkrsoiodwk")
public suspend fun stageName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.stageName = mapped
}
internal fun build(): GetExportPlainArgs = GetExportPlainArgs(
apiId = apiId ?: throw PulumiNullFieldException("apiId"),
exportVersion = exportVersion,
includeExtensions = includeExtensions,
outputType = outputType ?: throw PulumiNullFieldException("outputType"),
specification = specification ?: throw PulumiNullFieldException("specification"),
stageName = stageName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy