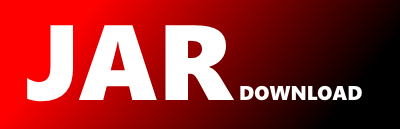
com.pulumi.aws.appautoscaling.kotlin.inputs.PolicyTargetTrackingScalingPolicyConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.appautoscaling.kotlin.inputs
import com.pulumi.aws.appautoscaling.inputs.PolicyTargetTrackingScalingPolicyConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property customizedMetricSpecification Custom CloudWatch metric. Documentation can be found at: [AWS Customized Metric Specification](https://docs.aws.amazon.com/autoscaling/ec2/APIReference/API_CustomizedMetricSpecification.html). See supported fields below.
* @property disableScaleIn Whether scale in by the target tracking policy is disabled. If the value is true, scale in is disabled and the target tracking policy won't remove capacity from the scalable resource. Otherwise, scale in is enabled and the target tracking policy can remove capacity from the scalable resource. The default value is `false`.
* @property predefinedMetricSpecification Predefined metric. See supported fields below.
* @property scaleInCooldown Amount of time, in seconds, after a scale in activity completes before another scale in activity can start.
* @property scaleOutCooldown Amount of time, in seconds, after a scale out activity completes before another scale out activity can start.
* @property targetValue Target value for the metric.
*/
public data class PolicyTargetTrackingScalingPolicyConfigurationArgs(
public val customizedMetricSpecification: Output? =
null,
public val disableScaleIn: Output? = null,
public val predefinedMetricSpecification: Output? =
null,
public val scaleInCooldown: Output? = null,
public val scaleOutCooldown: Output? = null,
public val targetValue: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.appautoscaling.inputs.PolicyTargetTrackingScalingPolicyConfigurationArgs =
com.pulumi.aws.appautoscaling.inputs.PolicyTargetTrackingScalingPolicyConfigurationArgs.builder()
.customizedMetricSpecification(
customizedMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.disableScaleIn(disableScaleIn?.applyValue({ args0 -> args0 }))
.predefinedMetricSpecification(
predefinedMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.scaleInCooldown(scaleInCooldown?.applyValue({ args0 -> args0 }))
.scaleOutCooldown(scaleOutCooldown?.applyValue({ args0 -> args0 }))
.targetValue(targetValue.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PolicyTargetTrackingScalingPolicyConfigurationArgs].
*/
@PulumiTagMarker
public class PolicyTargetTrackingScalingPolicyConfigurationArgsBuilder internal constructor() {
private var customizedMetricSpecification:
Output? =
null
private var disableScaleIn: Output? = null
private var predefinedMetricSpecification:
Output? =
null
private var scaleInCooldown: Output? = null
private var scaleOutCooldown: Output? = null
private var targetValue: Output? = null
/**
* @param value Custom CloudWatch metric. Documentation can be found at: [AWS Customized Metric Specification](https://docs.aws.amazon.com/autoscaling/ec2/APIReference/API_CustomizedMetricSpecification.html). See supported fields below.
*/
@JvmName("ayxiqyxnmbwpiekk")
public suspend fun customizedMetricSpecification(`value`: Output) {
this.customizedMetricSpecification = value
}
/**
* @param value Whether scale in by the target tracking policy is disabled. If the value is true, scale in is disabled and the target tracking policy won't remove capacity from the scalable resource. Otherwise, scale in is enabled and the target tracking policy can remove capacity from the scalable resource. The default value is `false`.
*/
@JvmName("ycqxuclsfppbakqh")
public suspend fun disableScaleIn(`value`: Output) {
this.disableScaleIn = value
}
/**
* @param value Predefined metric. See supported fields below.
*/
@JvmName("atvpnrbfahwbgjvn")
public suspend fun predefinedMetricSpecification(`value`: Output) {
this.predefinedMetricSpecification = value
}
/**
* @param value Amount of time, in seconds, after a scale in activity completes before another scale in activity can start.
*/
@JvmName("cgqgdsglldddffgn")
public suspend fun scaleInCooldown(`value`: Output) {
this.scaleInCooldown = value
}
/**
* @param value Amount of time, in seconds, after a scale out activity completes before another scale out activity can start.
*/
@JvmName("stjehaqkdrroshej")
public suspend fun scaleOutCooldown(`value`: Output) {
this.scaleOutCooldown = value
}
/**
* @param value Target value for the metric.
*/
@JvmName("ftvvndhwkrodglnp")
public suspend fun targetValue(`value`: Output) {
this.targetValue = value
}
/**
* @param value Custom CloudWatch metric. Documentation can be found at: [AWS Customized Metric Specification](https://docs.aws.amazon.com/autoscaling/ec2/APIReference/API_CustomizedMetricSpecification.html). See supported fields below.
*/
@JvmName("eyxidujwsvfgcnnn")
public suspend fun customizedMetricSpecification(`value`: PolicyTargetTrackingScalingPolicyConfigurationCustomizedMetricSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customizedMetricSpecification = mapped
}
/**
* @param argument Custom CloudWatch metric. Documentation can be found at: [AWS Customized Metric Specification](https://docs.aws.amazon.com/autoscaling/ec2/APIReference/API_CustomizedMetricSpecification.html). See supported fields below.
*/
@JvmName("ekopvrraylqxabuj")
public suspend fun customizedMetricSpecification(argument: suspend PolicyTargetTrackingScalingPolicyConfigurationCustomizedMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyTargetTrackingScalingPolicyConfigurationCustomizedMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customizedMetricSpecification = mapped
}
/**
* @param value Whether scale in by the target tracking policy is disabled. If the value is true, scale in is disabled and the target tracking policy won't remove capacity from the scalable resource. Otherwise, scale in is enabled and the target tracking policy can remove capacity from the scalable resource. The default value is `false`.
*/
@JvmName("blikbtkolymvqtdo")
public suspend fun disableScaleIn(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableScaleIn = mapped
}
/**
* @param value Predefined metric. See supported fields below.
*/
@JvmName("gvbagspanbjcrebw")
public suspend fun predefinedMetricSpecification(`value`: PolicyTargetTrackingScalingPolicyConfigurationPredefinedMetricSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.predefinedMetricSpecification = mapped
}
/**
* @param argument Predefined metric. See supported fields below.
*/
@JvmName("kownesgijtiliram")
public suspend fun predefinedMetricSpecification(argument: suspend PolicyTargetTrackingScalingPolicyConfigurationPredefinedMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyTargetTrackingScalingPolicyConfigurationPredefinedMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.predefinedMetricSpecification = mapped
}
/**
* @param value Amount of time, in seconds, after a scale in activity completes before another scale in activity can start.
*/
@JvmName("obwcwhsoxaqdoubu")
public suspend fun scaleInCooldown(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scaleInCooldown = mapped
}
/**
* @param value Amount of time, in seconds, after a scale out activity completes before another scale out activity can start.
*/
@JvmName("vcksiugmhpnjhmcb")
public suspend fun scaleOutCooldown(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scaleOutCooldown = mapped
}
/**
* @param value Target value for the metric.
*/
@JvmName("gafqummkkmymubyw")
public suspend fun targetValue(`value`: Double) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.targetValue = mapped
}
internal fun build(): PolicyTargetTrackingScalingPolicyConfigurationArgs =
PolicyTargetTrackingScalingPolicyConfigurationArgs(
customizedMetricSpecification = customizedMetricSpecification,
disableScaleIn = disableScaleIn,
predefinedMetricSpecification = predefinedMetricSpecification,
scaleInCooldown = scaleInCooldown,
scaleOutCooldown = scaleOutCooldown,
targetValue = targetValue ?: throw PulumiNullFieldException("targetValue"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy