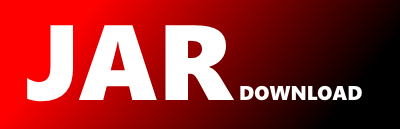
com.pulumi.aws.appflow.kotlin.Flow.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.appflow.kotlin
import com.pulumi.aws.appflow.kotlin.outputs.FlowDestinationFlowConfig
import com.pulumi.aws.appflow.kotlin.outputs.FlowMetadataCatalogConfig
import com.pulumi.aws.appflow.kotlin.outputs.FlowSourceFlowConfig
import com.pulumi.aws.appflow.kotlin.outputs.FlowTask
import com.pulumi.aws.appflow.kotlin.outputs.FlowTriggerConfig
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.appflow.kotlin.outputs.FlowDestinationFlowConfig.Companion.toKotlin as flowDestinationFlowConfigToKotlin
import com.pulumi.aws.appflow.kotlin.outputs.FlowMetadataCatalogConfig.Companion.toKotlin as flowMetadataCatalogConfigToKotlin
import com.pulumi.aws.appflow.kotlin.outputs.FlowSourceFlowConfig.Companion.toKotlin as flowSourceFlowConfigToKotlin
import com.pulumi.aws.appflow.kotlin.outputs.FlowTask.Companion.toKotlin as flowTaskToKotlin
import com.pulumi.aws.appflow.kotlin.outputs.FlowTriggerConfig.Companion.toKotlin as flowTriggerConfigToKotlin
/**
* Builder for [Flow].
*/
@PulumiTagMarker
public class FlowResourceBuilder internal constructor() {
public var name: String? = null
public var args: FlowArgs = FlowArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FlowArgsBuilder.() -> Unit) {
val builder = FlowArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Flow {
val builtJavaResource = com.pulumi.aws.appflow.Flow(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Flow(builtJavaResource)
}
}
/**
* Provides an AppFlow flow resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const exampleSourceBucketV2 = new aws.s3.BucketV2("example_source", {bucket: "example-source"});
* const exampleSource = aws.iam.getPolicyDocument({
* statements: [{
* sid: "AllowAppFlowSourceActions",
* effect: "Allow",
* principals: [{
* type: "Service",
* identifiers: ["appflow.amazonaws.com"],
* }],
* actions: [
* "s3:ListBucket",
* "s3:GetObject",
* ],
* resources: [
* "arn:aws:s3:::example-source",
* "arn:aws:s3:::example-source/*",
* ],
* }],
* });
* const exampleSourceBucketPolicy = new aws.s3.BucketPolicy("example_source", {
* bucket: exampleSourceBucketV2.id,
* policy: exampleSource.then(exampleSource => exampleSource.json),
* });
* const example = new aws.s3.BucketObjectv2("example", {
* bucket: exampleSourceBucketV2.id,
* key: "example_source.csv",
* source: new pulumi.asset.FileAsset("example_source.csv"),
* });
* const exampleDestinationBucketV2 = new aws.s3.BucketV2("example_destination", {bucket: "example-destination"});
* const exampleDestination = aws.iam.getPolicyDocument({
* statements: [{
* sid: "AllowAppFlowDestinationActions",
* effect: "Allow",
* principals: [{
* type: "Service",
* identifiers: ["appflow.amazonaws.com"],
* }],
* actions: [
* "s3:PutObject",
* "s3:AbortMultipartUpload",
* "s3:ListMultipartUploadParts",
* "s3:ListBucketMultipartUploads",
* "s3:GetBucketAcl",
* "s3:PutObjectAcl",
* ],
* resources: [
* "arn:aws:s3:::example-destination",
* "arn:aws:s3:::example-destination/*",
* ],
* }],
* });
* const exampleDestinationBucketPolicy = new aws.s3.BucketPolicy("example_destination", {
* bucket: exampleDestinationBucketV2.id,
* policy: exampleDestination.then(exampleDestination => exampleDestination.json),
* });
* const exampleFlow = new aws.appflow.Flow("example", {
* name: "example",
* sourceFlowConfig: {
* connectorType: "S3",
* sourceConnectorProperties: {
* s3: {
* bucketName: exampleSourceBucketPolicy.bucket,
* bucketPrefix: "example",
* },
* },
* },
* destinationFlowConfigs: [{
* connectorType: "S3",
* destinationConnectorProperties: {
* s3: {
* bucketName: exampleDestinationBucketPolicy.bucket,
* s3OutputFormatConfig: {
* prefixConfig: {
* prefixType: "PATH",
* },
* },
* },
* },
* }],
* tasks: [{
* sourceFields: ["exampleField"],
* destinationField: "exampleField",
* taskType: "Map",
* connectorOperators: [{
* s3: "NO_OP",
* }],
* }],
* triggerConfig: {
* triggerType: "OnDemand",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example_source_bucket_v2 = aws.s3.BucketV2("example_source", bucket="example-source")
* example_source = aws.iam.get_policy_document(statements=[{
* "sid": "AllowAppFlowSourceActions",
* "effect": "Allow",
* "principals": [{
* "type": "Service",
* "identifiers": ["appflow.amazonaws.com"],
* }],
* "actions": [
* "s3:ListBucket",
* "s3:GetObject",
* ],
* "resources": [
* "arn:aws:s3:::example-source",
* "arn:aws:s3:::example-source/*",
* ],
* }])
* example_source_bucket_policy = aws.s3.BucketPolicy("example_source",
* bucket=example_source_bucket_v2.id,
* policy=example_source.json)
* example = aws.s3.BucketObjectv2("example",
* bucket=example_source_bucket_v2.id,
* key="example_source.csv",
* source=pulumi.FileAsset("example_source.csv"))
* example_destination_bucket_v2 = aws.s3.BucketV2("example_destination", bucket="example-destination")
* example_destination = aws.iam.get_policy_document(statements=[{
* "sid": "AllowAppFlowDestinationActions",
* "effect": "Allow",
* "principals": [{
* "type": "Service",
* "identifiers": ["appflow.amazonaws.com"],
* }],
* "actions": [
* "s3:PutObject",
* "s3:AbortMultipartUpload",
* "s3:ListMultipartUploadParts",
* "s3:ListBucketMultipartUploads",
* "s3:GetBucketAcl",
* "s3:PutObjectAcl",
* ],
* "resources": [
* "arn:aws:s3:::example-destination",
* "arn:aws:s3:::example-destination/*",
* ],
* }])
* example_destination_bucket_policy = aws.s3.BucketPolicy("example_destination",
* bucket=example_destination_bucket_v2.id,
* policy=example_destination.json)
* example_flow = aws.appflow.Flow("example",
* name="example",
* source_flow_config={
* "connector_type": "S3",
* "source_connector_properties": {
* "s3": {
* "bucket_name": example_source_bucket_policy.bucket,
* "bucket_prefix": "example",
* },
* },
* },
* destination_flow_configs=[{
* "connector_type": "S3",
* "destination_connector_properties": {
* "s3": {
* "bucket_name": example_destination_bucket_policy.bucket,
* "s3_output_format_config": {
* "prefix_config": {
* "prefix_type": "PATH",
* },
* },
* },
* },
* }],
* tasks=[{
* "source_fields": ["exampleField"],
* "destination_field": "exampleField",
* "task_type": "Map",
* "connector_operators": [{
* "s3": "NO_OP",
* }],
* }],
* trigger_config={
* "trigger_type": "OnDemand",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var exampleSourceBucketV2 = new Aws.S3.BucketV2("example_source", new()
* {
* Bucket = "example-source",
* });
* var exampleSource = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Sid = "AllowAppFlowSourceActions",
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "appflow.amazonaws.com",
* },
* },
* },
* Actions = new[]
* {
* "s3:ListBucket",
* "s3:GetObject",
* },
* Resources = new[]
* {
* "arn:aws:s3:::example-source",
* "arn:aws:s3:::example-source/*",
* },
* },
* },
* });
* var exampleSourceBucketPolicy = new Aws.S3.BucketPolicy("example_source", new()
* {
* Bucket = exampleSourceBucketV2.Id,
* Policy = exampleSource.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var example = new Aws.S3.BucketObjectv2("example", new()
* {
* Bucket = exampleSourceBucketV2.Id,
* Key = "example_source.csv",
* Source = new FileAsset("example_source.csv"),
* });
* var exampleDestinationBucketV2 = new Aws.S3.BucketV2("example_destination", new()
* {
* Bucket = "example-destination",
* });
* var exampleDestination = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Sid = "AllowAppFlowDestinationActions",
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "appflow.amazonaws.com",
* },
* },
* },
* Actions = new[]
* {
* "s3:PutObject",
* "s3:AbortMultipartUpload",
* "s3:ListMultipartUploadParts",
* "s3:ListBucketMultipartUploads",
* "s3:GetBucketAcl",
* "s3:PutObjectAcl",
* },
* Resources = new[]
* {
* "arn:aws:s3:::example-destination",
* "arn:aws:s3:::example-destination/*",
* },
* },
* },
* });
* var exampleDestinationBucketPolicy = new Aws.S3.BucketPolicy("example_destination", new()
* {
* Bucket = exampleDestinationBucketV2.Id,
* Policy = exampleDestination.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var exampleFlow = new Aws.AppFlow.Flow("example", new()
* {
* Name = "example",
* SourceFlowConfig = new Aws.AppFlow.Inputs.FlowSourceFlowConfigArgs
* {
* ConnectorType = "S3",
* SourceConnectorProperties = new Aws.AppFlow.Inputs.FlowSourceFlowConfigSourceConnectorPropertiesArgs
* {
* S3 = new Aws.AppFlow.Inputs.FlowSourceFlowConfigSourceConnectorPropertiesS3Args
* {
* BucketName = exampleSourceBucketPolicy.Bucket,
* BucketPrefix = "example",
* },
* },
* },
* DestinationFlowConfigs = new[]
* {
* new Aws.AppFlow.Inputs.FlowDestinationFlowConfigArgs
* {
* ConnectorType = "S3",
* DestinationConnectorProperties = new Aws.AppFlow.Inputs.FlowDestinationFlowConfigDestinationConnectorPropertiesArgs
* {
* S3 = new Aws.AppFlow.Inputs.FlowDestinationFlowConfigDestinationConnectorPropertiesS3Args
* {
* BucketName = exampleDestinationBucketPolicy.Bucket,
* S3OutputFormatConfig = new Aws.AppFlow.Inputs.FlowDestinationFlowConfigDestinationConnectorPropertiesS3S3OutputFormatConfigArgs
* {
* PrefixConfig = new Aws.AppFlow.Inputs.FlowDestinationFlowConfigDestinationConnectorPropertiesS3S3OutputFormatConfigPrefixConfigArgs
* {
* PrefixType = "PATH",
* },
* },
* },
* },
* },
* },
* Tasks = new[]
* {
* new Aws.AppFlow.Inputs.FlowTaskArgs
* {
* SourceFields = new[]
* {
* "exampleField",
* },
* DestinationField = "exampleField",
* TaskType = "Map",
* ConnectorOperators = new[]
* {
* new Aws.AppFlow.Inputs.FlowTaskConnectorOperatorArgs
* {
* S3 = "NO_OP",
* },
* },
* },
* },
* TriggerConfig = new Aws.AppFlow.Inputs.FlowTriggerConfigArgs
* {
* TriggerType = "OnDemand",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/appflow"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleSourceBucketV2, err := s3.NewBucketV2(ctx, "example_source", &s3.BucketV2Args{
* Bucket: pulumi.String("example-source"),
* })
* if err != nil {
* return err
* }
* exampleSource, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Sid: pulumi.StringRef("AllowAppFlowSourceActions"),
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* "appflow.amazonaws.com",
* },
* },
* },
* Actions: []string{
* "s3:ListBucket",
* "s3:GetObject",
* },
* Resources: []string{
* "arn:aws:s3:::example-source",
* "arn:aws:s3:::example-source/*",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* exampleSourceBucketPolicy, err := s3.NewBucketPolicy(ctx, "example_source", &s3.BucketPolicyArgs{
* Bucket: exampleSourceBucketV2.ID(),
* Policy: pulumi.String(exampleSource.Json),
* })
* if err != nil {
* return err
* }
* _, err = s3.NewBucketObjectv2(ctx, "example", &s3.BucketObjectv2Args{
* Bucket: exampleSourceBucketV2.ID(),
* Key: pulumi.String("example_source.csv"),
* Source: pulumi.NewFileAsset("example_source.csv"),
* })
* if err != nil {
* return err
* }
* exampleDestinationBucketV2, err := s3.NewBucketV2(ctx, "example_destination", &s3.BucketV2Args{
* Bucket: pulumi.String("example-destination"),
* })
* if err != nil {
* return err
* }
* exampleDestination, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Sid: pulumi.StringRef("AllowAppFlowDestinationActions"),
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* "appflow.amazonaws.com",
* },
* },
* },
* Actions: []string{
* "s3:PutObject",
* "s3:AbortMultipartUpload",
* "s3:ListMultipartUploadParts",
* "s3:ListBucketMultipartUploads",
* "s3:GetBucketAcl",
* "s3:PutObjectAcl",
* },
* Resources: []string{
* "arn:aws:s3:::example-destination",
* "arn:aws:s3:::example-destination/*",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* exampleDestinationBucketPolicy, err := s3.NewBucketPolicy(ctx, "example_destination", &s3.BucketPolicyArgs{
* Bucket: exampleDestinationBucketV2.ID(),
* Policy: pulumi.String(exampleDestination.Json),
* })
* if err != nil {
* return err
* }
* _, err = appflow.NewFlow(ctx, "example", &appflow.FlowArgs{
* Name: pulumi.String("example"),
* SourceFlowConfig: &appflow.FlowSourceFlowConfigArgs{
* ConnectorType: pulumi.String("S3"),
* SourceConnectorProperties: &appflow.FlowSourceFlowConfigSourceConnectorPropertiesArgs{
* S3: &appflow.FlowSourceFlowConfigSourceConnectorPropertiesS3Args{
* BucketName: exampleSourceBucketPolicy.Bucket,
* BucketPrefix: pulumi.String("example"),
* },
* },
* },
* DestinationFlowConfigs: appflow.FlowDestinationFlowConfigArray{
* &appflow.FlowDestinationFlowConfigArgs{
* ConnectorType: pulumi.String("S3"),
* DestinationConnectorProperties: &appflow.FlowDestinationFlowConfigDestinationConnectorPropertiesArgs{
* S3: &appflow.FlowDestinationFlowConfigDestinationConnectorPropertiesS3Args{
* BucketName: exampleDestinationBucketPolicy.Bucket,
* S3OutputFormatConfig: &appflow.FlowDestinationFlowConfigDestinationConnectorPropertiesS3S3OutputFormatConfigArgs{
* PrefixConfig: &appflow.FlowDestinationFlowConfigDestinationConnectorPropertiesS3S3OutputFormatConfigPrefixConfigArgs{
* PrefixType: pulumi.String("PATH"),
* },
* },
* },
* },
* },
* },
* Tasks: appflow.FlowTaskArray{
* &appflow.FlowTaskArgs{
* SourceFields: pulumi.StringArray{
* pulumi.String("exampleField"),
* },
* DestinationField: pulumi.String("exampleField"),
* TaskType: pulumi.String("Map"),
* ConnectorOperators: appflow.FlowTaskConnectorOperatorArray{
* &appflow.FlowTaskConnectorOperatorArgs{
* S3: pulumi.String("NO_OP"),
* },
* },
* },
* },
* TriggerConfig: &appflow.FlowTriggerConfigArgs{
* TriggerType: pulumi.String("OnDemand"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.s3.BucketPolicy;
* import com.pulumi.aws.s3.BucketPolicyArgs;
* import com.pulumi.aws.s3.BucketObjectv2;
* import com.pulumi.aws.s3.BucketObjectv2Args;
* import com.pulumi.aws.appflow.Flow;
* import com.pulumi.aws.appflow.FlowArgs;
* import com.pulumi.aws.appflow.inputs.FlowSourceFlowConfigArgs;
* import com.pulumi.aws.appflow.inputs.FlowSourceFlowConfigSourceConnectorPropertiesArgs;
* import com.pulumi.aws.appflow.inputs.FlowSourceFlowConfigSourceConnectorPropertiesS3Args;
* import com.pulumi.aws.appflow.inputs.FlowDestinationFlowConfigArgs;
* import com.pulumi.aws.appflow.inputs.FlowDestinationFlowConfigDestinationConnectorPropertiesArgs;
* import com.pulumi.aws.appflow.inputs.FlowDestinationFlowConfigDestinationConnectorPropertiesS3Args;
* import com.pulumi.aws.appflow.inputs.FlowDestinationFlowConfigDestinationConnectorPropertiesS3S3OutputFormatConfigArgs;
* import com.pulumi.aws.appflow.inputs.FlowDestinationFlowConfigDestinationConnectorPropertiesS3S3OutputFormatConfigPrefixConfigArgs;
* import com.pulumi.aws.appflow.inputs.FlowTaskArgs;
* import com.pulumi.aws.appflow.inputs.FlowTriggerConfigArgs;
* import com.pulumi.asset.FileAsset;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleSourceBucketV2 = new BucketV2("exampleSourceBucketV2", BucketV2Args.builder()
* .bucket("example-source")
* .build());
* final var exampleSource = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .sid("AllowAppFlowSourceActions")
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("appflow.amazonaws.com")
* .build())
* .actions(
* "s3:ListBucket",
* "s3:GetObject")
* .resources(
* "arn:aws:s3:::example-source",
* "arn:aws:s3:::example-source/*")
* .build())
* .build());
* var exampleSourceBucketPolicy = new BucketPolicy("exampleSourceBucketPolicy", BucketPolicyArgs.builder()
* .bucket(exampleSourceBucketV2.id())
* .policy(exampleSource.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var example = new BucketObjectv2("example", BucketObjectv2Args.builder()
* .bucket(exampleSourceBucketV2.id())
* .key("example_source.csv")
* .source(new FileAsset("example_source.csv"))
* .build());
* var exampleDestinationBucketV2 = new BucketV2("exampleDestinationBucketV2", BucketV2Args.builder()
* .bucket("example-destination")
* .build());
* final var exampleDestination = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .sid("AllowAppFlowDestinationActions")
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("appflow.amazonaws.com")
* .build())
* .actions(
* "s3:PutObject",
* "s3:AbortMultipartUpload",
* "s3:ListMultipartUploadParts",
* "s3:ListBucketMultipartUploads",
* "s3:GetBucketAcl",
* "s3:PutObjectAcl")
* .resources(
* "arn:aws:s3:::example-destination",
* "arn:aws:s3:::example-destination/*")
* .build())
* .build());
* var exampleDestinationBucketPolicy = new BucketPolicy("exampleDestinationBucketPolicy", BucketPolicyArgs.builder()
* .bucket(exampleDestinationBucketV2.id())
* .policy(exampleDestination.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var exampleFlow = new Flow("exampleFlow", FlowArgs.builder()
* .name("example")
* .sourceFlowConfig(FlowSourceFlowConfigArgs.builder()
* .connectorType("S3")
* .sourceConnectorProperties(FlowSourceFlowConfigSourceConnectorPropertiesArgs.builder()
* .s3(FlowSourceFlowConfigSourceConnectorPropertiesS3Args.builder()
* .bucketName(exampleSourceBucketPolicy.bucket())
* .bucketPrefix("example")
* .build())
* .build())
* .build())
* .destinationFlowConfigs(FlowDestinationFlowConfigArgs.builder()
* .connectorType("S3")
* .destinationConnectorProperties(FlowDestinationFlowConfigDestinationConnectorPropertiesArgs.builder()
* .s3(FlowDestinationFlowConfigDestinationConnectorPropertiesS3Args.builder()
* .bucketName(exampleDestinationBucketPolicy.bucket())
* .s3OutputFormatConfig(FlowDestinationFlowConfigDestinationConnectorPropertiesS3S3OutputFormatConfigArgs.builder()
* .prefixConfig(FlowDestinationFlowConfigDestinationConnectorPropertiesS3S3OutputFormatConfigPrefixConfigArgs.builder()
* .prefixType("PATH")
* .build())
* .build())
* .build())
* .build())
* .build())
* .tasks(FlowTaskArgs.builder()
* .sourceFields("exampleField")
* .destinationField("exampleField")
* .taskType("Map")
* .connectorOperators(FlowTaskConnectorOperatorArgs.builder()
* .s3("NO_OP")
* .build())
* .build())
* .triggerConfig(FlowTriggerConfigArgs.builder()
* .triggerType("OnDemand")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleSourceBucketV2:
* type: aws:s3:BucketV2
* name: example_source
* properties:
* bucket: example-source
* exampleSourceBucketPolicy:
* type: aws:s3:BucketPolicy
* name: example_source
* properties:
* bucket: ${exampleSourceBucketV2.id}
* policy: ${exampleSource.json}
* example:
* type: aws:s3:BucketObjectv2
* properties:
* bucket: ${exampleSourceBucketV2.id}
* key: example_source.csv
* source:
* fn::FileAsset: example_source.csv
* exampleDestinationBucketV2:
* type: aws:s3:BucketV2
* name: example_destination
* properties:
* bucket: example-destination
* exampleDestinationBucketPolicy:
* type: aws:s3:BucketPolicy
* name: example_destination
* properties:
* bucket: ${exampleDestinationBucketV2.id}
* policy: ${exampleDestination.json}
* exampleFlow:
* type: aws:appflow:Flow
* name: example
* properties:
* name: example
* sourceFlowConfig:
* connectorType: S3
* sourceConnectorProperties:
* s3:
* bucketName: ${exampleSourceBucketPolicy.bucket}
* bucketPrefix: example
* destinationFlowConfigs:
* - connectorType: S3
* destinationConnectorProperties:
* s3:
* bucketName: ${exampleDestinationBucketPolicy.bucket}
* s3OutputFormatConfig:
* prefixConfig:
* prefixType: PATH
* tasks:
* - sourceFields:
* - exampleField
* destinationField: exampleField
* taskType: Map
* connectorOperators:
* - s3: NO_OP
* triggerConfig:
* triggerType: OnDemand
* variables:
* exampleSource:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - sid: AllowAppFlowSourceActions
* effect: Allow
* principals:
* - type: Service
* identifiers:
* - appflow.amazonaws.com
* actions:
* - s3:ListBucket
* - s3:GetObject
* resources:
* - arn:aws:s3:::example-source
* - arn:aws:s3:::example-source/*
* exampleDestination:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - sid: AllowAppFlowDestinationActions
* effect: Allow
* principals:
* - type: Service
* identifiers:
* - appflow.amazonaws.com
* actions:
* - s3:PutObject
* - s3:AbortMultipartUpload
* - s3:ListMultipartUploadParts
* - s3:ListBucketMultipartUploads
* - s3:GetBucketAcl
* - s3:PutObjectAcl
* resources:
* - arn:aws:s3:::example-destination
* - arn:aws:s3:::example-destination/*
* ```
*
* ## Import
* Using `pulumi import`, import AppFlow flows using the `arn`. For example:
* ```sh
* $ pulumi import aws:appflow/flow:Flow example arn:aws:appflow:us-west-2:123456789012:flow/example-flow
* ```
* */*/*/*/*/*/*/*/*/*/*/*/
*/
public class Flow internal constructor(
override val javaResource: com.pulumi.aws.appflow.Flow,
) : KotlinCustomResource(javaResource, FlowMapper) {
/**
* Flow's ARN.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Description of the flow you want to create.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A Destination Flow Config that controls how Amazon AppFlow places data in the destination connector.
*/
public val destinationFlowConfigs: Output>
get() = javaResource.destinationFlowConfigs().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> flowDestinationFlowConfigToKotlin(args0) })
})
})
/**
* The current status of the flow.
*/
public val flowStatus: Output
get() = javaResource.flowStatus().applyValue({ args0 -> args0 })
/**
* ARN (Amazon Resource Name) of the Key Management Service (KMS) key you provide for encryption. This is required if you do not want to use the Amazon AppFlow-managed KMS key. If you don't provide anything here, Amazon AppFlow uses the Amazon AppFlow-managed KMS key.
*/
public val kmsArn: Output
get() = javaResource.kmsArn().applyValue({ args0 -> args0 })
/**
* A Catalog that determines the configuration that Amazon AppFlow uses when it catalogs the data that’s transferred by the associated flow. When Amazon AppFlow catalogs the data from a flow, it stores metadata in a data catalog.
*/
public val metadataCatalogConfig: Output
get() = javaResource.metadataCatalogConfig().applyValue({ args0 ->
args0.let({ args0 ->
flowMetadataCatalogConfigToKotlin(args0)
})
})
/**
* Name of the flow.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The Source Flow Config that controls how Amazon AppFlow retrieves data from the source connector.
*/
public val sourceFlowConfig: Output
get() = javaResource.sourceFlowConfig().applyValue({ args0 ->
args0.let({ args0 ->
flowSourceFlowConfigToKotlin(args0)
})
})
/**
* Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy