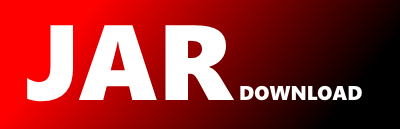
com.pulumi.aws.appmesh.kotlin.inputs.RouteSpecHttp2RouteRetryPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.appmesh.kotlin.inputs
import com.pulumi.aws.appmesh.inputs.RouteSpecHttp2RouteRetryPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property httpRetryEvents List of HTTP retry events.
* Valid values: `client-error` (HTTP status code 409), `gateway-error` (HTTP status codes 502, 503, and 504), `server-error` (HTTP status codes 500, 501, 502, 503, 504, 505, 506, 507, 508, 510, and 511), `stream-error` (retry on refused stream).
* @property maxRetries Maximum number of retries.
* @property perRetryTimeout Per-retry timeout.
* @property tcpRetryEvents List of TCP retry events. The only valid value is `connection-error`.
* You must specify at least one value for `http_retry_events`, or at least one value for `tcp_retry_events`.
*/
public data class RouteSpecHttp2RouteRetryPolicyArgs(
public val httpRetryEvents: Output>? = null,
public val maxRetries: Output,
public val perRetryTimeout: Output,
public val tcpRetryEvents: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.appmesh.inputs.RouteSpecHttp2RouteRetryPolicyArgs =
com.pulumi.aws.appmesh.inputs.RouteSpecHttp2RouteRetryPolicyArgs.builder()
.httpRetryEvents(httpRetryEvents?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.maxRetries(maxRetries.applyValue({ args0 -> args0 }))
.perRetryTimeout(perRetryTimeout.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tcpRetryEvents(tcpRetryEvents?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [RouteSpecHttp2RouteRetryPolicyArgs].
*/
@PulumiTagMarker
public class RouteSpecHttp2RouteRetryPolicyArgsBuilder internal constructor() {
private var httpRetryEvents: Output>? = null
private var maxRetries: Output? = null
private var perRetryTimeout: Output? = null
private var tcpRetryEvents: Output>? = null
/**
* @param value List of HTTP retry events.
* Valid values: `client-error` (HTTP status code 409), `gateway-error` (HTTP status codes 502, 503, and 504), `server-error` (HTTP status codes 500, 501, 502, 503, 504, 505, 506, 507, 508, 510, and 511), `stream-error` (retry on refused stream).
*/
@JvmName("rytvnhwutoxkrgli")
public suspend fun httpRetryEvents(`value`: Output>) {
this.httpRetryEvents = value
}
@JvmName("jgycgwyjgmbatorv")
public suspend fun httpRetryEvents(vararg values: Output) {
this.httpRetryEvents = Output.all(values.asList())
}
/**
* @param values List of HTTP retry events.
* Valid values: `client-error` (HTTP status code 409), `gateway-error` (HTTP status codes 502, 503, and 504), `server-error` (HTTP status codes 500, 501, 502, 503, 504, 505, 506, 507, 508, 510, and 511), `stream-error` (retry on refused stream).
*/
@JvmName("wangrygqflryicok")
public suspend fun httpRetryEvents(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy