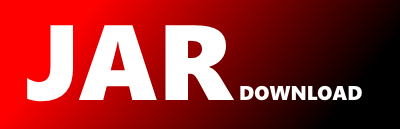
com.pulumi.aws.apprunner.kotlin.CustomDomainAssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.apprunner.kotlin
import com.pulumi.aws.apprunner.CustomDomainAssociationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages an App Runner Custom Domain association.
* > **NOTE:** After creation, you must use the information in the `certification_validation_records` attribute to add CNAME records to your Domain Name System (DNS). For each mapped domain name, add a mapping to the target App Runner subdomain (found in the `dns_target` attribute) and one or more certificate validation records. App Runner then performs DNS validation to verify that you own or control the domain name you associated. App Runner tracks domain validity in a certificate stored in AWS Certificate Manager (ACM).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.apprunner.CustomDomainAssociation("example", {
* domainName: "example.com",
* serviceArn: exampleAwsApprunnerService.arn,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.apprunner.CustomDomainAssociation("example",
* domain_name="example.com",
* service_arn=example_aws_apprunner_service["arn"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.AppRunner.CustomDomainAssociation("example", new()
* {
* DomainName = "example.com",
* ServiceArn = exampleAwsApprunnerService.Arn,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/apprunner"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := apprunner.NewCustomDomainAssociation(ctx, "example", &apprunner.CustomDomainAssociationArgs{
* DomainName: pulumi.String("example.com"),
* ServiceArn: pulumi.Any(exampleAwsApprunnerService.Arn),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apprunner.CustomDomainAssociation;
* import com.pulumi.aws.apprunner.CustomDomainAssociationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new CustomDomainAssociation("example", CustomDomainAssociationArgs.builder()
* .domainName("example.com")
* .serviceArn(exampleAwsApprunnerService.arn())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:apprunner:CustomDomainAssociation
* properties:
* domainName: example.com
* serviceArn: ${exampleAwsApprunnerService.arn}
* ```
*
* ## Import
* Using `pulumi import`, import App Runner Custom Domain Associations using the `domain_name` and `service_arn` separated by a comma (`,`). For example:
* ```sh
* $ pulumi import aws:apprunner/customDomainAssociation:CustomDomainAssociation example example.com,arn:aws:apprunner:us-east-1:123456789012:service/example-app/8fe1e10304f84fd2b0df550fe98a71fa
* ```
* @property domainName Custom domain endpoint to association. Specify a base domain e.g., `example.com` or a subdomain e.g., `subdomain.example.com`.
* @property enableWwwSubdomain Whether to associate the subdomain with the App Runner service in addition to the base domain. Defaults to `true`.
* @property serviceArn ARN of the App Runner service.
*/
public data class CustomDomainAssociationArgs(
public val domainName: Output? = null,
public val enableWwwSubdomain: Output? = null,
public val serviceArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.apprunner.CustomDomainAssociationArgs =
com.pulumi.aws.apprunner.CustomDomainAssociationArgs.builder()
.domainName(domainName?.applyValue({ args0 -> args0 }))
.enableWwwSubdomain(enableWwwSubdomain?.applyValue({ args0 -> args0 }))
.serviceArn(serviceArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CustomDomainAssociationArgs].
*/
@PulumiTagMarker
public class CustomDomainAssociationArgsBuilder internal constructor() {
private var domainName: Output? = null
private var enableWwwSubdomain: Output? = null
private var serviceArn: Output? = null
/**
* @param value Custom domain endpoint to association. Specify a base domain e.g., `example.com` or a subdomain e.g., `subdomain.example.com`.
*/
@JvmName("khmboklvnvvlanym")
public suspend fun domainName(`value`: Output) {
this.domainName = value
}
/**
* @param value Whether to associate the subdomain with the App Runner service in addition to the base domain. Defaults to `true`.
*/
@JvmName("pyksvsemgcnoghwm")
public suspend fun enableWwwSubdomain(`value`: Output) {
this.enableWwwSubdomain = value
}
/**
* @param value ARN of the App Runner service.
*/
@JvmName("dnuoscvidygnmeju")
public suspend fun serviceArn(`value`: Output) {
this.serviceArn = value
}
/**
* @param value Custom domain endpoint to association. Specify a base domain e.g., `example.com` or a subdomain e.g., `subdomain.example.com`.
*/
@JvmName("lvxpafluxiwphbry")
public suspend fun domainName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.domainName = mapped
}
/**
* @param value Whether to associate the subdomain with the App Runner service in addition to the base domain. Defaults to `true`.
*/
@JvmName("atykcskwhhcxlgqh")
public suspend fun enableWwwSubdomain(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableWwwSubdomain = mapped
}
/**
* @param value ARN of the App Runner service.
*/
@JvmName("rktcbdcadqkbphxv")
public suspend fun serviceArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceArn = mapped
}
internal fun build(): CustomDomainAssociationArgs = CustomDomainAssociationArgs(
domainName = domainName,
enableWwwSubdomain = enableWwwSubdomain,
serviceArn = serviceArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy