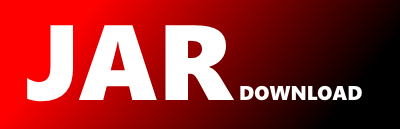
com.pulumi.aws.apprunner.kotlin.inputs.ServiceSourceConfigurationCodeRepositoryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.apprunner.kotlin.inputs
import com.pulumi.aws.apprunner.inputs.ServiceSourceConfigurationCodeRepositoryArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property codeConfiguration Configuration for building and running the service from a source code repository. See Code Configuration below for more details.
* @property repositoryUrl Location of the repository that contains the source code.
* @property sourceCodeVersion Version that should be used within the source code repository. See Source Code Version below for more details.
* @property sourceDirectory The path of the directory that stores source code and configuration files. The build and start commands also execute from here. The path is absolute from root and, if not specified, defaults to the repository root.
*/
public data class ServiceSourceConfigurationCodeRepositoryArgs(
public val codeConfiguration: Output? = null,
public val repositoryUrl: Output,
public val sourceCodeVersion: Output,
public val sourceDirectory: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.apprunner.inputs.ServiceSourceConfigurationCodeRepositoryArgs =
com.pulumi.aws.apprunner.inputs.ServiceSourceConfigurationCodeRepositoryArgs.builder()
.codeConfiguration(codeConfiguration?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.repositoryUrl(repositoryUrl.applyValue({ args0 -> args0 }))
.sourceCodeVersion(sourceCodeVersion.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sourceDirectory(sourceDirectory?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServiceSourceConfigurationCodeRepositoryArgs].
*/
@PulumiTagMarker
public class ServiceSourceConfigurationCodeRepositoryArgsBuilder internal constructor() {
private var codeConfiguration:
Output? = null
private var repositoryUrl: Output? = null
private var sourceCodeVersion:
Output? = null
private var sourceDirectory: Output? = null
/**
* @param value Configuration for building and running the service from a source code repository. See Code Configuration below for more details.
*/
@JvmName("htagmmnbvwxcuvab")
public suspend fun codeConfiguration(`value`: Output) {
this.codeConfiguration = value
}
/**
* @param value Location of the repository that contains the source code.
*/
@JvmName("rpurqfguvobofppp")
public suspend fun repositoryUrl(`value`: Output) {
this.repositoryUrl = value
}
/**
* @param value Version that should be used within the source code repository. See Source Code Version below for more details.
*/
@JvmName("acpwrtndjxvaepku")
public suspend fun sourceCodeVersion(`value`: Output) {
this.sourceCodeVersion = value
}
/**
* @param value The path of the directory that stores source code and configuration files. The build and start commands also execute from here. The path is absolute from root and, if not specified, defaults to the repository root.
*/
@JvmName("cielgyhyorfofbip")
public suspend fun sourceDirectory(`value`: Output) {
this.sourceDirectory = value
}
/**
* @param value Configuration for building and running the service from a source code repository. See Code Configuration below for more details.
*/
@JvmName("qhhhbbgfgbvltoeo")
public suspend fun codeConfiguration(`value`: ServiceSourceConfigurationCodeRepositoryCodeConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.codeConfiguration = mapped
}
/**
* @param argument Configuration for building and running the service from a source code repository. See Code Configuration below for more details.
*/
@JvmName("xipkdbkhbmwpchxu")
public suspend fun codeConfiguration(argument: suspend ServiceSourceConfigurationCodeRepositoryCodeConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
ServiceSourceConfigurationCodeRepositoryCodeConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.codeConfiguration = mapped
}
/**
* @param value Location of the repository that contains the source code.
*/
@JvmName("txqpctfqlmyoicgo")
public suspend fun repositoryUrl(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.repositoryUrl = mapped
}
/**
* @param value Version that should be used within the source code repository. See Source Code Version below for more details.
*/
@JvmName("klvqbivqgrqfxuav")
public suspend fun sourceCodeVersion(`value`: ServiceSourceConfigurationCodeRepositorySourceCodeVersionArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceCodeVersion = mapped
}
/**
* @param argument Version that should be used within the source code repository. See Source Code Version below for more details.
*/
@JvmName("dhitnpkchsymfvsq")
public suspend fun sourceCodeVersion(argument: suspend ServiceSourceConfigurationCodeRepositorySourceCodeVersionArgsBuilder.() -> Unit) {
val toBeMapped =
ServiceSourceConfigurationCodeRepositorySourceCodeVersionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sourceCodeVersion = mapped
}
/**
* @param value The path of the directory that stores source code and configuration files. The build and start commands also execute from here. The path is absolute from root and, if not specified, defaults to the repository root.
*/
@JvmName("raqbuyvrumylwwfd")
public suspend fun sourceDirectory(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceDirectory = mapped
}
internal fun build(): ServiceSourceConfigurationCodeRepositoryArgs =
ServiceSourceConfigurationCodeRepositoryArgs(
codeConfiguration = codeConfiguration,
repositoryUrl = repositoryUrl ?: throw PulumiNullFieldException("repositoryUrl"),
sourceCodeVersion = sourceCodeVersion ?: throw PulumiNullFieldException("sourceCodeVersion"),
sourceDirectory = sourceDirectory,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy