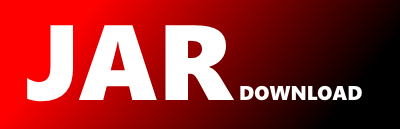
com.pulumi.aws.appstream.kotlin.Fleet.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.appstream.kotlin
import com.pulumi.aws.appstream.kotlin.outputs.FleetComputeCapacity
import com.pulumi.aws.appstream.kotlin.outputs.FleetDomainJoinInfo
import com.pulumi.aws.appstream.kotlin.outputs.FleetVpcConfig
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.aws.appstream.kotlin.outputs.FleetComputeCapacity.Companion.toKotlin as fleetComputeCapacityToKotlin
import com.pulumi.aws.appstream.kotlin.outputs.FleetDomainJoinInfo.Companion.toKotlin as fleetDomainJoinInfoToKotlin
import com.pulumi.aws.appstream.kotlin.outputs.FleetVpcConfig.Companion.toKotlin as fleetVpcConfigToKotlin
/**
* Builder for [Fleet].
*/
@PulumiTagMarker
public class FleetResourceBuilder internal constructor() {
public var name: String? = null
public var args: FleetArgs = FleetArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FleetArgsBuilder.() -> Unit) {
val builder = FleetArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Fleet {
val builtJavaResource = com.pulumi.aws.appstream.Fleet(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Fleet(builtJavaResource)
}
}
/**
* Provides an AppStream fleet.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const testFleet = new aws.appstream.Fleet("test_fleet", {
* name: "test-fleet",
* computeCapacity: {
* desiredInstances: 1,
* },
* description: "test fleet",
* idleDisconnectTimeoutInSeconds: 60,
* displayName: "test-fleet",
* enableDefaultInternetAccess: false,
* fleetType: "ON_DEMAND",
* imageName: "Amazon-AppStream2-Sample-Image-03-11-2023",
* instanceType: "stream.standard.large",
* maxUserDurationInSeconds: 600,
* vpcConfig: {
* subnetIds: ["subnet-06e9b13400c225127"],
* },
* tags: {
* TagName: "tag-value",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test_fleet = aws.appstream.Fleet("test_fleet",
* name="test-fleet",
* compute_capacity={
* "desired_instances": 1,
* },
* description="test fleet",
* idle_disconnect_timeout_in_seconds=60,
* display_name="test-fleet",
* enable_default_internet_access=False,
* fleet_type="ON_DEMAND",
* image_name="Amazon-AppStream2-Sample-Image-03-11-2023",
* instance_type="stream.standard.large",
* max_user_duration_in_seconds=600,
* vpc_config={
* "subnet_ids": ["subnet-06e9b13400c225127"],
* },
* tags={
* "TagName": "tag-value",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var testFleet = new Aws.AppStream.Fleet("test_fleet", new()
* {
* Name = "test-fleet",
* ComputeCapacity = new Aws.AppStream.Inputs.FleetComputeCapacityArgs
* {
* DesiredInstances = 1,
* },
* Description = "test fleet",
* IdleDisconnectTimeoutInSeconds = 60,
* DisplayName = "test-fleet",
* EnableDefaultInternetAccess = false,
* FleetType = "ON_DEMAND",
* ImageName = "Amazon-AppStream2-Sample-Image-03-11-2023",
* InstanceType = "stream.standard.large",
* MaxUserDurationInSeconds = 600,
* VpcConfig = new Aws.AppStream.Inputs.FleetVpcConfigArgs
* {
* SubnetIds = new[]
* {
* "subnet-06e9b13400c225127",
* },
* },
* Tags =
* {
* { "TagName", "tag-value" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/appstream"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := appstream.NewFleet(ctx, "test_fleet", &appstream.FleetArgs{
* Name: pulumi.String("test-fleet"),
* ComputeCapacity: &appstream.FleetComputeCapacityArgs{
* DesiredInstances: pulumi.Int(1),
* },
* Description: pulumi.String("test fleet"),
* IdleDisconnectTimeoutInSeconds: pulumi.Int(60),
* DisplayName: pulumi.String("test-fleet"),
* EnableDefaultInternetAccess: pulumi.Bool(false),
* FleetType: pulumi.String("ON_DEMAND"),
* ImageName: pulumi.String("Amazon-AppStream2-Sample-Image-03-11-2023"),
* InstanceType: pulumi.String("stream.standard.large"),
* MaxUserDurationInSeconds: pulumi.Int(600),
* VpcConfig: &appstream.FleetVpcConfigArgs{
* SubnetIds: pulumi.StringArray{
* pulumi.String("subnet-06e9b13400c225127"),
* },
* },
* Tags: pulumi.StringMap{
* "TagName": pulumi.String("tag-value"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appstream.Fleet;
* import com.pulumi.aws.appstream.FleetArgs;
* import com.pulumi.aws.appstream.inputs.FleetComputeCapacityArgs;
* import com.pulumi.aws.appstream.inputs.FleetVpcConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var testFleet = new Fleet("testFleet", FleetArgs.builder()
* .name("test-fleet")
* .computeCapacity(FleetComputeCapacityArgs.builder()
* .desiredInstances(1)
* .build())
* .description("test fleet")
* .idleDisconnectTimeoutInSeconds(60)
* .displayName("test-fleet")
* .enableDefaultInternetAccess(false)
* .fleetType("ON_DEMAND")
* .imageName("Amazon-AppStream2-Sample-Image-03-11-2023")
* .instanceType("stream.standard.large")
* .maxUserDurationInSeconds(600)
* .vpcConfig(FleetVpcConfigArgs.builder()
* .subnetIds("subnet-06e9b13400c225127")
* .build())
* .tags(Map.of("TagName", "tag-value"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* testFleet:
* type: aws:appstream:Fleet
* name: test_fleet
* properties:
* name: test-fleet
* computeCapacity:
* desiredInstances: 1
* description: test fleet
* idleDisconnectTimeoutInSeconds: 60
* displayName: test-fleet
* enableDefaultInternetAccess: false
* fleetType: ON_DEMAND
* imageName: Amazon-AppStream2-Sample-Image-03-11-2023
* instanceType: stream.standard.large
* maxUserDurationInSeconds: 600
* vpcConfig:
* subnetIds:
* - subnet-06e9b13400c225127
* tags:
* TagName: tag-value
* ```
*
* ## Import
* Using `pulumi import`, import `aws_appstream_fleet` using the id. For example:
* ```sh
* $ pulumi import aws:appstream/fleet:Fleet example fleetNameExample
* ```
*/
public class Fleet internal constructor(
override val javaResource: com.pulumi.aws.appstream.Fleet,
) : KotlinCustomResource(javaResource, FleetMapper) {
/**
* ARN of the appstream fleet.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Configuration block for the desired capacity of the fleet. See below.
*/
public val computeCapacity: Output
get() = javaResource.computeCapacity().applyValue({ args0 ->
args0.let({ args0 ->
fleetComputeCapacityToKotlin(args0)
})
})
/**
* Date and time, in UTC and extended RFC 3339 format, when the fleet was created.
*/
public val createdTime: Output
get() = javaResource.createdTime().applyValue({ args0 -> args0 })
/**
* Description to display.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* Amount of time that a streaming session remains active after users disconnect.
*/
public val disconnectTimeoutInSeconds: Output
get() = javaResource.disconnectTimeoutInSeconds().applyValue({ args0 -> args0 })
/**
* Human-readable friendly name for the AppStream fleet.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* Configuration block for the name of the directory and organizational unit (OU) to use to join the fleet to a Microsoft Active Directory domain. See below.
*/
public val domainJoinInfo: Output
get() = javaResource.domainJoinInfo().applyValue({ args0 ->
args0.let({ args0 ->
fleetDomainJoinInfoToKotlin(args0)
})
})
/**
* Enables or disables default internet access for the fleet.
*/
public val enableDefaultInternetAccess: Output
get() = javaResource.enableDefaultInternetAccess().applyValue({ args0 -> args0 })
/**
* Fleet type. Valid values are: `ON_DEMAND`, `ALWAYS_ON`
*/
public val fleetType: Output
get() = javaResource.fleetType().applyValue({ args0 -> args0 })
/**
* ARN of the IAM role to apply to the fleet.
*/
public val iamRoleArn: Output
get() = javaResource.iamRoleArn().applyValue({ args0 -> args0 })
/**
* Amount of time that users can be idle (inactive) before they are disconnected from their streaming session and the `disconnect_timeout_in_seconds` time interval begins. Defaults to `0`. Valid value is between `60` and `3600 `seconds.
*/
public val idleDisconnectTimeoutInSeconds: Output?
get() = javaResource.idleDisconnectTimeoutInSeconds().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* ARN of the public, private, or shared image to use.
*/
public val imageArn: Output
get() = javaResource.imageArn().applyValue({ args0 -> args0 })
/**
* Name of the image used to create the fleet.
*/
public val imageName: Output
get() = javaResource.imageName().applyValue({ args0 -> args0 })
/**
* Instance type to use when launching fleet instances.
*/
public val instanceType: Output
get() = javaResource.instanceType().applyValue({ args0 -> args0 })
/**
* The maximum number of user sessions on an instance. This only applies to multi-session fleets.
*/
public val maxSessionsPerInstance: Output?
get() = javaResource.maxSessionsPerInstance().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Maximum amount of time that a streaming session can remain active, in seconds.
*/
public val maxUserDurationInSeconds: Output
get() = javaResource.maxUserDurationInSeconds().applyValue({ args0 -> args0 })
/**
* Unique name for the fleet.
* The following arguments are optional:
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* State of the fleet. Can be `STARTING`, `RUNNING`, `STOPPING` or `STOPPED`
*/
public val state: Output
get() = javaResource.state().applyValue({ args0 -> args0 })
/**
* AppStream 2.0 view that is displayed to your users when they stream from the fleet. When `APP` is specified, only the windows of applications opened by users display. When `DESKTOP` is specified, the standard desktop that is provided by the operating system displays. If not specified, defaults to `APP`.
*/
public val streamView: Output
get() = javaResource.streamView().applyValue({ args0 -> args0 })
/**
* Map of tags to attach to AppStream instances.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy