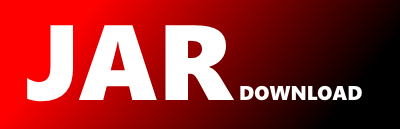
com.pulumi.aws.appstream.kotlin.UserStackAssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.appstream.kotlin
import com.pulumi.aws.appstream.UserStackAssociationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages an AppStream User Stack association.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.appstream.Stack("test", {name: "STACK NAME"});
* const testUser = new aws.appstream.User("test", {
* authenticationType: "USERPOOL",
* userName: "EMAIL",
* });
* const testUserStackAssociation = new aws.appstream.UserStackAssociation("test", {
* authenticationType: testUser.authenticationType,
* stackName: test.name,
* userName: testUser.userName,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test = aws.appstream.Stack("test", name="STACK NAME")
* test_user = aws.appstream.User("test",
* authentication_type="USERPOOL",
* user_name="EMAIL")
* test_user_stack_association = aws.appstream.UserStackAssociation("test",
* authentication_type=test_user.authentication_type,
* stack_name=test.name,
* user_name=test_user.user_name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.AppStream.Stack("test", new()
* {
* Name = "STACK NAME",
* });
* var testUser = new Aws.AppStream.User("test", new()
* {
* AuthenticationType = "USERPOOL",
* UserName = "EMAIL",
* });
* var testUserStackAssociation = new Aws.AppStream.UserStackAssociation("test", new()
* {
* AuthenticationType = testUser.AuthenticationType,
* StackName = test.Name,
* UserName = testUser.UserName,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/appstream"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* test, err := appstream.NewStack(ctx, "test", &appstream.StackArgs{
* Name: pulumi.String("STACK NAME"),
* })
* if err != nil {
* return err
* }
* testUser, err := appstream.NewUser(ctx, "test", &appstream.UserArgs{
* AuthenticationType: pulumi.String("USERPOOL"),
* UserName: pulumi.String("EMAIL"),
* })
* if err != nil {
* return err
* }
* _, err = appstream.NewUserStackAssociation(ctx, "test", &appstream.UserStackAssociationArgs{
* AuthenticationType: testUser.AuthenticationType,
* StackName: test.Name,
* UserName: testUser.UserName,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appstream.Stack;
* import com.pulumi.aws.appstream.StackArgs;
* import com.pulumi.aws.appstream.User;
* import com.pulumi.aws.appstream.UserArgs;
* import com.pulumi.aws.appstream.UserStackAssociation;
* import com.pulumi.aws.appstream.UserStackAssociationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new Stack("test", StackArgs.builder()
* .name("STACK NAME")
* .build());
* var testUser = new User("testUser", UserArgs.builder()
* .authenticationType("USERPOOL")
* .userName("EMAIL")
* .build());
* var testUserStackAssociation = new UserStackAssociation("testUserStackAssociation", UserStackAssociationArgs.builder()
* .authenticationType(testUser.authenticationType())
* .stackName(test.name())
* .userName(testUser.userName())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:appstream:Stack
* properties:
* name: STACK NAME
* testUser:
* type: aws:appstream:User
* name: test
* properties:
* authenticationType: USERPOOL
* userName: EMAIL
* testUserStackAssociation:
* type: aws:appstream:UserStackAssociation
* name: test
* properties:
* authenticationType: ${testUser.authenticationType}
* stackName: ${test.name}
* userName: ${testUser.userName}
* ```
*
* ## Import
* Using `pulumi import`, import AppStream User Stack Association using the `user_name`, `authentication_type`, and `stack_name`, separated by a slash (`/`). For example:
* ```sh
* $ pulumi import aws:appstream/userStackAssociation:UserStackAssociation example userName/auhtenticationType/stackName
* ```
* @property authenticationType Authentication type for the user.
* @property sendEmailNotification Whether a welcome email is sent to a user after the user is created in the user pool.
* @property stackName Name of the stack that is associated with the user.
* @property userName Email address of the user who is associated with the stack.
* The following arguments are optional:
*/
public data class UserStackAssociationArgs(
public val authenticationType: Output? = null,
public val sendEmailNotification: Output? = null,
public val stackName: Output? = null,
public val userName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.appstream.UserStackAssociationArgs =
com.pulumi.aws.appstream.UserStackAssociationArgs.builder()
.authenticationType(authenticationType?.applyValue({ args0 -> args0 }))
.sendEmailNotification(sendEmailNotification?.applyValue({ args0 -> args0 }))
.stackName(stackName?.applyValue({ args0 -> args0 }))
.userName(userName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UserStackAssociationArgs].
*/
@PulumiTagMarker
public class UserStackAssociationArgsBuilder internal constructor() {
private var authenticationType: Output? = null
private var sendEmailNotification: Output? = null
private var stackName: Output? = null
private var userName: Output? = null
/**
* @param value Authentication type for the user.
*/
@JvmName("vdkrbuptnxkurqgf")
public suspend fun authenticationType(`value`: Output) {
this.authenticationType = value
}
/**
* @param value Whether a welcome email is sent to a user after the user is created in the user pool.
*/
@JvmName("kkmwvyrctxauodkh")
public suspend fun sendEmailNotification(`value`: Output) {
this.sendEmailNotification = value
}
/**
* @param value Name of the stack that is associated with the user.
*/
@JvmName("qogpbssyevfiwkef")
public suspend fun stackName(`value`: Output) {
this.stackName = value
}
/**
* @param value Email address of the user who is associated with the stack.
* The following arguments are optional:
*/
@JvmName("tkeivkpnucnnwlov")
public suspend fun userName(`value`: Output) {
this.userName = value
}
/**
* @param value Authentication type for the user.
*/
@JvmName("glowdtbgflyuokgu")
public suspend fun authenticationType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authenticationType = mapped
}
/**
* @param value Whether a welcome email is sent to a user after the user is created in the user pool.
*/
@JvmName("oilbfihmijkmerev")
public suspend fun sendEmailNotification(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sendEmailNotification = mapped
}
/**
* @param value Name of the stack that is associated with the user.
*/
@JvmName("lkfgkslkksanvcnv")
public suspend fun stackName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stackName = mapped
}
/**
* @param value Email address of the user who is associated with the stack.
* The following arguments are optional:
*/
@JvmName("ieqmxifkayguyltr")
public suspend fun userName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userName = mapped
}
internal fun build(): UserStackAssociationArgs = UserStackAssociationArgs(
authenticationType = authenticationType,
sendEmailNotification = sendEmailNotification,
stackName = stackName,
userName = userName,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy