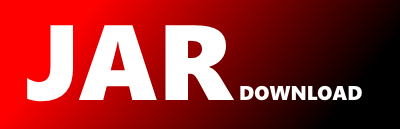
com.pulumi.aws.appsync.kotlin.GraphQLApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.appsync.kotlin
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiAdditionalAuthenticationProvider
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiEnhancedMetricsConfig
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiLambdaAuthorizerConfig
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiLogConfig
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiOpenidConnectConfig
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiUserPoolConfig
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiAdditionalAuthenticationProvider.Companion.toKotlin as graphQLApiAdditionalAuthenticationProviderToKotlin
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiEnhancedMetricsConfig.Companion.toKotlin as graphQLApiEnhancedMetricsConfigToKotlin
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiLambdaAuthorizerConfig.Companion.toKotlin as graphQLApiLambdaAuthorizerConfigToKotlin
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiLogConfig.Companion.toKotlin as graphQLApiLogConfigToKotlin
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiOpenidConnectConfig.Companion.toKotlin as graphQLApiOpenidConnectConfigToKotlin
import com.pulumi.aws.appsync.kotlin.outputs.GraphQLApiUserPoolConfig.Companion.toKotlin as graphQLApiUserPoolConfigToKotlin
/**
* Builder for [GraphQLApi].
*/
@PulumiTagMarker
public class GraphQLApiResourceBuilder internal constructor() {
public var name: String? = null
public var args: GraphQLApiArgs = GraphQLApiArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend GraphQLApiArgsBuilder.() -> Unit) {
val builder = GraphQLApiArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): GraphQLApi {
val builtJavaResource = com.pulumi.aws.appsync.GraphQLApi(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return GraphQLApi(builtJavaResource)
}
}
public class GraphQLApi internal constructor(
override val javaResource: com.pulumi.aws.appsync.GraphQLApi,
) : KotlinCustomResource(javaResource, GraphQLApiMapper) {
/**
* One or more additional authentication providers for the GraphQL API. See `additional_authentication_provider` Block for details.
*/
public val additionalAuthenticationProviders:
Output>?
get() = javaResource.additionalAuthenticationProviders().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
graphQLApiAdditionalAuthenticationProviderToKotlin(args0)
})
})
}).orElse(null)
})
/**
* API type. Valid values are `GRAPHQL` or `MERGED`. A `MERGED` type requires `merged_api_execution_role_arn` to be set.
*/
public val apiType: Output?
get() = javaResource.apiType().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* ARN
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Authentication type. Valid values: `API_KEY`, `AWS_IAM`, `AMAZON_COGNITO_USER_POOLS`, `OPENID_CONNECT`, `AWS_LAMBDA`
*/
public val authenticationType: Output
get() = javaResource.authenticationType().applyValue({ args0 -> args0 })
/**
* Enables and controls the enhanced metrics feature. See `enhanced_metrics_config` Block for details.
*/
public val enhancedMetricsConfig: Output?
get() = javaResource.enhancedMetricsConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> graphQLApiEnhancedMetricsConfigToKotlin(args0) })
}).orElse(null)
})
/**
* Sets the value of the GraphQL API to enable (`ENABLED`) or disable (`DISABLED`) introspection. If no value is provided, the introspection configuration will be set to ENABLED by default. This field will produce an error if the operation attempts to use the introspection feature while this field is disabled. For more information about introspection, see [GraphQL introspection](https://graphql.org/learn/introspection/).
*/
public val introspectionConfig: Output?
get() = javaResource.introspectionConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Nested argument containing Lambda authorizer configuration. See `lambda_authorizer_config` Block for details.
*/
public val lambdaAuthorizerConfig: Output?
get() = javaResource.lambdaAuthorizerConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> graphQLApiLambdaAuthorizerConfigToKotlin(args0) })
}).orElse(null)
})
/**
* Nested argument containing logging configuration. See `log_config` Block for details.
*/
public val logConfig: Output?
get() = javaResource.logConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
graphQLApiLogConfigToKotlin(args0)
})
}).orElse(null)
})
/**
* ARN of the execution role when `api_type` is set to `MERGED`.
*/
public val mergedApiExecutionRoleArn: Output?
get() = javaResource.mergedApiExecutionRoleArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* User-supplied name for the GraphQL API.
* The following arguments are optional:
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Nested argument containing OpenID Connect configuration. See `openid_connect_config` Block for details.
*/
public val openidConnectConfig: Output?
get() = javaResource.openidConnectConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> graphQLApiOpenidConnectConfigToKotlin(args0) })
}).orElse(null)
})
/**
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels allowed in the body of query. The default value is `0` (or unspecified), which indicates there's no depth limit. If you set a limit, it can be between `1` and `75` nested levels. This field will produce a limit error if the operation falls out of bounds.
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error, the error will be thrown upwards to the first nullable field available.
*/
public val queryDepthLimit: Output?
get() = javaResource.queryDepthLimit().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum number of resolvers that can be invoked in a single request. The default value is `0` (or unspecified), which will set the limit to `10000`. When specified, the limit value can be between `1` and `10000`. This field will produce a limit error if the operation falls out of bounds.
*/
public val resolverCountLimit: Output?
get() = javaResource.resolverCountLimit().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Schema definition, in GraphQL schema language format. This provider cannot perform drift detection of this configuration.
*/
public val schema: Output?
get() = javaResource.schema().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy