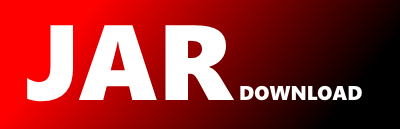
com.pulumi.aws.appsync.kotlin.inputs.GraphQLApiAdditionalAuthenticationProviderArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.appsync.kotlin.inputs
import com.pulumi.aws.appsync.inputs.GraphQLApiAdditionalAuthenticationProviderArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property authenticationType Authentication type. Valid values: `API_KEY`, `AWS_IAM`, `AMAZON_COGNITO_USER_POOLS`, `OPENID_CONNECT`, `AWS_LAMBDA`
* @property lambdaAuthorizerConfig Nested argument containing Lambda authorizer configuration. See `lambda_authorizer_config` Block for details.
* @property openidConnectConfig Nested argument containing OpenID Connect configuration. See `openid_connect_config` Block for details.
* @property userPoolConfig Amazon Cognito User Pool configuration. See `user_pool_config` Block for details.
*/
public data class GraphQLApiAdditionalAuthenticationProviderArgs(
public val authenticationType: Output,
public val lambdaAuthorizerConfig: Output? = null,
public val openidConnectConfig: Output? = null,
public val userPoolConfig: Output? =
null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.appsync.inputs.GraphQLApiAdditionalAuthenticationProviderArgs =
com.pulumi.aws.appsync.inputs.GraphQLApiAdditionalAuthenticationProviderArgs.builder()
.authenticationType(authenticationType.applyValue({ args0 -> args0 }))
.lambdaAuthorizerConfig(
lambdaAuthorizerConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.openidConnectConfig(
openidConnectConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.userPoolConfig(
userPoolConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [GraphQLApiAdditionalAuthenticationProviderArgs].
*/
@PulumiTagMarker
public class GraphQLApiAdditionalAuthenticationProviderArgsBuilder internal constructor() {
private var authenticationType: Output? = null
private var lambdaAuthorizerConfig:
Output? = null
private var openidConnectConfig:
Output? = null
private var userPoolConfig: Output? =
null
/**
* @param value Authentication type. Valid values: `API_KEY`, `AWS_IAM`, `AMAZON_COGNITO_USER_POOLS`, `OPENID_CONNECT`, `AWS_LAMBDA`
*/
@JvmName("bjuhoyjjaxoaxqvj")
public suspend fun authenticationType(`value`: Output) {
this.authenticationType = value
}
/**
* @param value Nested argument containing Lambda authorizer configuration. See `lambda_authorizer_config` Block for details.
*/
@JvmName("fcrnqgsfcdspdryp")
public suspend fun lambdaAuthorizerConfig(`value`: Output) {
this.lambdaAuthorizerConfig = value
}
/**
* @param value Nested argument containing OpenID Connect configuration. See `openid_connect_config` Block for details.
*/
@JvmName("gkyepgjybljxschl")
public suspend fun openidConnectConfig(`value`: Output) {
this.openidConnectConfig = value
}
/**
* @param value Amazon Cognito User Pool configuration. See `user_pool_config` Block for details.
*/
@JvmName("iqbkwyhsihjjivhs")
public suspend fun userPoolConfig(`value`: Output) {
this.userPoolConfig = value
}
/**
* @param value Authentication type. Valid values: `API_KEY`, `AWS_IAM`, `AMAZON_COGNITO_USER_POOLS`, `OPENID_CONNECT`, `AWS_LAMBDA`
*/
@JvmName("dupldihefmfoivnw")
public suspend fun authenticationType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authenticationType = mapped
}
/**
* @param value Nested argument containing Lambda authorizer configuration. See `lambda_authorizer_config` Block for details.
*/
@JvmName("soxywvreyitgprhm")
public suspend fun lambdaAuthorizerConfig(`value`: GraphQLApiAdditionalAuthenticationProviderLambdaAuthorizerConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lambdaAuthorizerConfig = mapped
}
/**
* @param argument Nested argument containing Lambda authorizer configuration. See `lambda_authorizer_config` Block for details.
*/
@JvmName("giaegptgigodouar")
public suspend fun lambdaAuthorizerConfig(argument: suspend GraphQLApiAdditionalAuthenticationProviderLambdaAuthorizerConfigArgsBuilder.() -> Unit) {
val toBeMapped =
GraphQLApiAdditionalAuthenticationProviderLambdaAuthorizerConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.lambdaAuthorizerConfig = mapped
}
/**
* @param value Nested argument containing OpenID Connect configuration. See `openid_connect_config` Block for details.
*/
@JvmName("qagffvsbvqmbyiqp")
public suspend fun openidConnectConfig(`value`: GraphQLApiAdditionalAuthenticationProviderOpenidConnectConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.openidConnectConfig = mapped
}
/**
* @param argument Nested argument containing OpenID Connect configuration. See `openid_connect_config` Block for details.
*/
@JvmName("ubyxaqwklmmkgydc")
public suspend fun openidConnectConfig(argument: suspend GraphQLApiAdditionalAuthenticationProviderOpenidConnectConfigArgsBuilder.() -> Unit) {
val toBeMapped =
GraphQLApiAdditionalAuthenticationProviderOpenidConnectConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.openidConnectConfig = mapped
}
/**
* @param value Amazon Cognito User Pool configuration. See `user_pool_config` Block for details.
*/
@JvmName("xibaabcycaakymri")
public suspend fun userPoolConfig(`value`: GraphQLApiAdditionalAuthenticationProviderUserPoolConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userPoolConfig = mapped
}
/**
* @param argument Amazon Cognito User Pool configuration. See `user_pool_config` Block for details.
*/
@JvmName("knvocxnkeqgvkeye")
public suspend fun userPoolConfig(argument: suspend GraphQLApiAdditionalAuthenticationProviderUserPoolConfigArgsBuilder.() -> Unit) {
val toBeMapped =
GraphQLApiAdditionalAuthenticationProviderUserPoolConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.userPoolConfig = mapped
}
internal fun build(): GraphQLApiAdditionalAuthenticationProviderArgs =
GraphQLApiAdditionalAuthenticationProviderArgs(
authenticationType = authenticationType ?: throw PulumiNullFieldException("authenticationType"),
lambdaAuthorizerConfig = lambdaAuthorizerConfig,
openidConnectConfig = openidConnectConfig,
userPoolConfig = userPoolConfig,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy