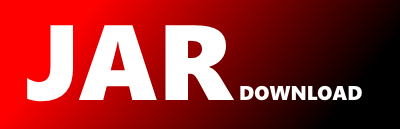
com.pulumi.aws.auditmanager.kotlin.inputs.ControlControlMappingSourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.auditmanager.kotlin.inputs
import com.pulumi.aws.auditmanager.inputs.ControlControlMappingSourceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property sourceDescription Description of the source.
* @property sourceFrequency Frequency of evidence collection. Valid values are `DAILY`, `WEEKLY`, or `MONTHLY`.
* @property sourceId
* @property sourceKeyword The keyword to search for in CloudTrail logs, Config rules, Security Hub checks, and Amazon Web Services API names. See `source_keyword` below.
* @property sourceName Name of the source.
* @property sourceSetUpOption The setup option for the data source. This option reflects if the evidence collection is automated or manual. Valid values are `System_Controls_Mapping` (automated) and `Procedural_Controls_Mapping` (manual).
* @property sourceType Type of data source for evidence collection. If `source_set_up_option` is manual, the only valid value is `MANUAL`. If `source_set_up_option` is automated, valid values are `AWS_Cloudtrail`, `AWS_Config`, `AWS_Security_Hub`, or `AWS_API_Call`.
* The following arguments are optional:
* @property troubleshootingText Instructions for troubleshooting the control.
*/
public data class ControlControlMappingSourceArgs(
public val sourceDescription: Output? = null,
public val sourceFrequency: Output? = null,
public val sourceId: Output? = null,
public val sourceKeyword: Output? = null,
public val sourceName: Output,
public val sourceSetUpOption: Output,
public val sourceType: Output,
public val troubleshootingText: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.auditmanager.inputs.ControlControlMappingSourceArgs =
com.pulumi.aws.auditmanager.inputs.ControlControlMappingSourceArgs.builder()
.sourceDescription(sourceDescription?.applyValue({ args0 -> args0 }))
.sourceFrequency(sourceFrequency?.applyValue({ args0 -> args0 }))
.sourceId(sourceId?.applyValue({ args0 -> args0 }))
.sourceKeyword(sourceKeyword?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sourceName(sourceName.applyValue({ args0 -> args0 }))
.sourceSetUpOption(sourceSetUpOption.applyValue({ args0 -> args0 }))
.sourceType(sourceType.applyValue({ args0 -> args0 }))
.troubleshootingText(troubleshootingText?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ControlControlMappingSourceArgs].
*/
@PulumiTagMarker
public class ControlControlMappingSourceArgsBuilder internal constructor() {
private var sourceDescription: Output? = null
private var sourceFrequency: Output? = null
private var sourceId: Output? = null
private var sourceKeyword: Output? = null
private var sourceName: Output? = null
private var sourceSetUpOption: Output? = null
private var sourceType: Output? = null
private var troubleshootingText: Output? = null
/**
* @param value Description of the source.
*/
@JvmName("rcuhbgfspmaeixqh")
public suspend fun sourceDescription(`value`: Output) {
this.sourceDescription = value
}
/**
* @param value Frequency of evidence collection. Valid values are `DAILY`, `WEEKLY`, or `MONTHLY`.
*/
@JvmName("dvihgigboqnismib")
public suspend fun sourceFrequency(`value`: Output) {
this.sourceFrequency = value
}
/**
* @param value
*/
@JvmName("pircmalqwcuhofir")
public suspend fun sourceId(`value`: Output) {
this.sourceId = value
}
/**
* @param value The keyword to search for in CloudTrail logs, Config rules, Security Hub checks, and Amazon Web Services API names. See `source_keyword` below.
*/
@JvmName("geeqiwtjgrslhurq")
public suspend fun sourceKeyword(`value`: Output) {
this.sourceKeyword = value
}
/**
* @param value Name of the source.
*/
@JvmName("pdtsmhgfsuykqxwn")
public suspend fun sourceName(`value`: Output) {
this.sourceName = value
}
/**
* @param value The setup option for the data source. This option reflects if the evidence collection is automated or manual. Valid values are `System_Controls_Mapping` (automated) and `Procedural_Controls_Mapping` (manual).
*/
@JvmName("kfjjkqiretudhana")
public suspend fun sourceSetUpOption(`value`: Output) {
this.sourceSetUpOption = value
}
/**
* @param value Type of data source for evidence collection. If `source_set_up_option` is manual, the only valid value is `MANUAL`. If `source_set_up_option` is automated, valid values are `AWS_Cloudtrail`, `AWS_Config`, `AWS_Security_Hub`, or `AWS_API_Call`.
* The following arguments are optional:
*/
@JvmName("qfluhrtyfovdteew")
public suspend fun sourceType(`value`: Output) {
this.sourceType = value
}
/**
* @param value Instructions for troubleshooting the control.
*/
@JvmName("uswfwnsjkxwsbwli")
public suspend fun troubleshootingText(`value`: Output) {
this.troubleshootingText = value
}
/**
* @param value Description of the source.
*/
@JvmName("dwbgufkocotvnayh")
public suspend fun sourceDescription(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceDescription = mapped
}
/**
* @param value Frequency of evidence collection. Valid values are `DAILY`, `WEEKLY`, or `MONTHLY`.
*/
@JvmName("oaabnhkdmmdloysy")
public suspend fun sourceFrequency(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceFrequency = mapped
}
/**
* @param value
*/
@JvmName("naodncbgywutotsy")
public suspend fun sourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceId = mapped
}
/**
* @param value The keyword to search for in CloudTrail logs, Config rules, Security Hub checks, and Amazon Web Services API names. See `source_keyword` below.
*/
@JvmName("gpqsvqtresihhopo")
public suspend fun sourceKeyword(`value`: ControlControlMappingSourceSourceKeywordArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceKeyword = mapped
}
/**
* @param argument The keyword to search for in CloudTrail logs, Config rules, Security Hub checks, and Amazon Web Services API names. See `source_keyword` below.
*/
@JvmName("nuntfuyxiufkujoy")
public suspend fun sourceKeyword(argument: suspend ControlControlMappingSourceSourceKeywordArgsBuilder.() -> Unit) {
val toBeMapped = ControlControlMappingSourceSourceKeywordArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sourceKeyword = mapped
}
/**
* @param value Name of the source.
*/
@JvmName("ibcahlhliusbrmku")
public suspend fun sourceName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceName = mapped
}
/**
* @param value The setup option for the data source. This option reflects if the evidence collection is automated or manual. Valid values are `System_Controls_Mapping` (automated) and `Procedural_Controls_Mapping` (manual).
*/
@JvmName("taniivvvvprdsnsy")
public suspend fun sourceSetUpOption(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceSetUpOption = mapped
}
/**
* @param value Type of data source for evidence collection. If `source_set_up_option` is manual, the only valid value is `MANUAL`. If `source_set_up_option` is automated, valid values are `AWS_Cloudtrail`, `AWS_Config`, `AWS_Security_Hub`, or `AWS_API_Call`.
* The following arguments are optional:
*/
@JvmName("oikjokufarbhtkeo")
public suspend fun sourceType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceType = mapped
}
/**
* @param value Instructions for troubleshooting the control.
*/
@JvmName("bublbxcvxjjtmlpg")
public suspend fun troubleshootingText(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.troubleshootingText = mapped
}
internal fun build(): ControlControlMappingSourceArgs = ControlControlMappingSourceArgs(
sourceDescription = sourceDescription,
sourceFrequency = sourceFrequency,
sourceId = sourceId,
sourceKeyword = sourceKeyword,
sourceName = sourceName ?: throw PulumiNullFieldException("sourceName"),
sourceSetUpOption = sourceSetUpOption ?: throw PulumiNullFieldException("sourceSetUpOption"),
sourceType = sourceType ?: throw PulumiNullFieldException("sourceType"),
troubleshootingText = troubleshootingText,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy