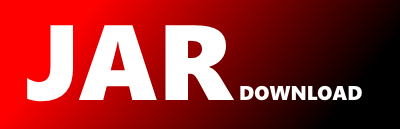
com.pulumi.aws.autoscaling.kotlin.Attachment.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscaling.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [Attachment].
*/
@PulumiTagMarker
public class AttachmentResourceBuilder internal constructor() {
public var name: String? = null
public var args: AttachmentArgs = AttachmentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AttachmentArgsBuilder.() -> Unit) {
val builder = AttachmentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Attachment {
val builtJavaResource = com.pulumi.aws.autoscaling.Attachment(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Attachment(builtJavaResource)
}
}
/**
* Attaches a load balancer to an Auto Scaling group.
* > **NOTE on Auto Scaling Groups, Attachments and Traffic Source Attachments:** Pulumi provides standalone Attachment (for attaching Classic Load Balancers and Application Load Balancer, Gateway Load Balancer, or Network Load Balancer target groups) and Traffic Source Attachment (for attaching Load Balancers and VPC Lattice target groups) resources and an Auto Scaling Group resource with `load_balancers`, `target_group_arns` and `traffic_source` attributes. Do not use the same traffic source in more than one of these resources. Doing so will cause a conflict of attachments. A `lifecycle` configuration block can be used to suppress differences if necessary.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* // Create a new load balancer attachment
* const example = new aws.autoscaling.Attachment("example", {
* autoscalingGroupName: exampleAwsAutoscalingGroup.id,
* elb: exampleAwsElb.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* # Create a new load balancer attachment
* example = aws.autoscaling.Attachment("example",
* autoscaling_group_name=example_aws_autoscaling_group["id"],
* elb=example_aws_elb["id"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* // Create a new load balancer attachment
* var example = new Aws.AutoScaling.Attachment("example", new()
* {
* AutoscalingGroupName = exampleAwsAutoscalingGroup.Id,
* Elb = exampleAwsElb.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/autoscaling"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* // Create a new load balancer attachment
* _, err := autoscaling.NewAttachment(ctx, "example", &autoscaling.AttachmentArgs{
* AutoscalingGroupName: pulumi.Any(exampleAwsAutoscalingGroup.Id),
* Elb: pulumi.Any(exampleAwsElb.Id),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.autoscaling.Attachment;
* import com.pulumi.aws.autoscaling.AttachmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* // Create a new load balancer attachment
* var example = new Attachment("example", AttachmentArgs.builder()
* .autoscalingGroupName(exampleAwsAutoscalingGroup.id())
* .elb(exampleAwsElb.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* # Create a new load balancer attachment
* example:
* type: aws:autoscaling:Attachment
* properties:
* autoscalingGroupName: ${exampleAwsAutoscalingGroup.id}
* elb: ${exampleAwsElb.id}
* ```
*
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* // Create a new ALB Target Group attachment
* const example = new aws.autoscaling.Attachment("example", {
* autoscalingGroupName: exampleAwsAutoscalingGroup.id,
* lbTargetGroupArn: exampleAwsLbTargetGroup.arn,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* # Create a new ALB Target Group attachment
* example = aws.autoscaling.Attachment("example",
* autoscaling_group_name=example_aws_autoscaling_group["id"],
* lb_target_group_arn=example_aws_lb_target_group["arn"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* // Create a new ALB Target Group attachment
* var example = new Aws.AutoScaling.Attachment("example", new()
* {
* AutoscalingGroupName = exampleAwsAutoscalingGroup.Id,
* LbTargetGroupArn = exampleAwsLbTargetGroup.Arn,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/autoscaling"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* // Create a new ALB Target Group attachment
* _, err := autoscaling.NewAttachment(ctx, "example", &autoscaling.AttachmentArgs{
* AutoscalingGroupName: pulumi.Any(exampleAwsAutoscalingGroup.Id),
* LbTargetGroupArn: pulumi.Any(exampleAwsLbTargetGroup.Arn),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.autoscaling.Attachment;
* import com.pulumi.aws.autoscaling.AttachmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* // Create a new ALB Target Group attachment
* var example = new Attachment("example", AttachmentArgs.builder()
* .autoscalingGroupName(exampleAwsAutoscalingGroup.id())
* .lbTargetGroupArn(exampleAwsLbTargetGroup.arn())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* # Create a new ALB Target Group attachment
* example:
* type: aws:autoscaling:Attachment
* properties:
* autoscalingGroupName: ${exampleAwsAutoscalingGroup.id}
* lbTargetGroupArn: ${exampleAwsLbTargetGroup.arn}
* ```
*
*/
public class Attachment internal constructor(
override val javaResource: com.pulumi.aws.autoscaling.Attachment,
) : KotlinCustomResource(javaResource, AttachmentMapper) {
/**
* Name of ASG to associate with the ELB.
*/
public val autoscalingGroupName: Output
get() = javaResource.autoscalingGroupName().applyValue({ args0 -> args0 })
/**
* Name of the ELB.
*/
public val elb: Output?
get() = javaResource.elb().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* ARN of a load balancer target group.
*/
public val lbTargetGroupArn: Output?
get() = javaResource.lbTargetGroupArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object AttachmentMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.aws.autoscaling.Attachment::class == javaResource::class
override fun map(javaResource: Resource): Attachment = Attachment(
javaResource as
com.pulumi.aws.autoscaling.Attachment,
)
}
/**
* @see [Attachment].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Attachment].
*/
public suspend fun attachment(name: String, block: suspend AttachmentResourceBuilder.() -> Unit): Attachment {
val builder = AttachmentResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Attachment].
* @param name The _unique_ name of the resulting resource.
*/
public fun attachment(name: String): Attachment {
val builder = AttachmentResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy