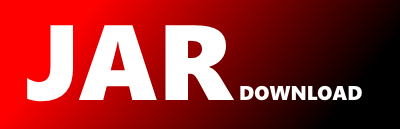
com.pulumi.aws.autoscaling.kotlin.TrafficSourceAttachmentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscaling.kotlin
import com.pulumi.aws.autoscaling.TrafficSourceAttachmentArgs.builder
import com.pulumi.aws.autoscaling.kotlin.inputs.TrafficSourceAttachmentTrafficSourceArgs
import com.pulumi.aws.autoscaling.kotlin.inputs.TrafficSourceAttachmentTrafficSourceArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Attaches a traffic source to an Auto Scaling group.
* > **NOTE on Auto Scaling Groups, Attachments and Traffic Source Attachments:** Pulumi provides standalone Attachment (for attaching Classic Load Balancers and Application Load Balancer, Gateway Load Balancer, or Network Load Balancer target groups) and Traffic Source Attachment (for attaching Load Balancers and VPC Lattice target groups) resources and an Auto Scaling Group resource with `load_balancers`, `target_group_arns` and `traffic_source` attributes. Do not use the same traffic source in more than one of these resources. Doing so will cause a conflict of attachments. A `lifecycle` configuration block can be used to suppress differences if necessary.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.autoscaling.TrafficSourceAttachment("example", {
* autoscalingGroupName: exampleAwsAutoscalingGroup.id,
* trafficSource: {
* identifier: exampleAwsLbTargetGroup.arn,
* type: "elbv2",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.autoscaling.TrafficSourceAttachment("example",
* autoscaling_group_name=example_aws_autoscaling_group["id"],
* traffic_source={
* "identifier": example_aws_lb_target_group["arn"],
* "type": "elbv2",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.AutoScaling.TrafficSourceAttachment("example", new()
* {
* AutoscalingGroupName = exampleAwsAutoscalingGroup.Id,
* TrafficSource = new Aws.AutoScaling.Inputs.TrafficSourceAttachmentTrafficSourceArgs
* {
* Identifier = exampleAwsLbTargetGroup.Arn,
* Type = "elbv2",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/autoscaling"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := autoscaling.NewTrafficSourceAttachment(ctx, "example", &autoscaling.TrafficSourceAttachmentArgs{
* AutoscalingGroupName: pulumi.Any(exampleAwsAutoscalingGroup.Id),
* TrafficSource: &autoscaling.TrafficSourceAttachmentTrafficSourceArgs{
* Identifier: pulumi.Any(exampleAwsLbTargetGroup.Arn),
* Type: pulumi.String("elbv2"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.autoscaling.TrafficSourceAttachment;
* import com.pulumi.aws.autoscaling.TrafficSourceAttachmentArgs;
* import com.pulumi.aws.autoscaling.inputs.TrafficSourceAttachmentTrafficSourceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new TrafficSourceAttachment("example", TrafficSourceAttachmentArgs.builder()
* .autoscalingGroupName(exampleAwsAutoscalingGroup.id())
* .trafficSource(TrafficSourceAttachmentTrafficSourceArgs.builder()
* .identifier(exampleAwsLbTargetGroup.arn())
* .type("elbv2")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:autoscaling:TrafficSourceAttachment
* properties:
* autoscalingGroupName: ${exampleAwsAutoscalingGroup.id}
* trafficSource:
* identifier: ${exampleAwsLbTargetGroup.arn}
* type: elbv2
* ```
*
* @property autoscalingGroupName The name of the Auto Scaling group.
* @property trafficSource The unique identifiers of a traffic sources.
*/
public data class TrafficSourceAttachmentArgs(
public val autoscalingGroupName: Output? = null,
public val trafficSource: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.autoscaling.TrafficSourceAttachmentArgs =
com.pulumi.aws.autoscaling.TrafficSourceAttachmentArgs.builder()
.autoscalingGroupName(autoscalingGroupName?.applyValue({ args0 -> args0 }))
.trafficSource(trafficSource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [TrafficSourceAttachmentArgs].
*/
@PulumiTagMarker
public class TrafficSourceAttachmentArgsBuilder internal constructor() {
private var autoscalingGroupName: Output? = null
private var trafficSource: Output? = null
/**
* @param value The name of the Auto Scaling group.
*/
@JvmName("ufvatpkyifreuxnq")
public suspend fun autoscalingGroupName(`value`: Output) {
this.autoscalingGroupName = value
}
/**
* @param value The unique identifiers of a traffic sources.
*/
@JvmName("ruekybblrkmdhvxq")
public suspend fun trafficSource(`value`: Output) {
this.trafficSource = value
}
/**
* @param value The name of the Auto Scaling group.
*/
@JvmName("cdsvgxkckdoqooqt")
public suspend fun autoscalingGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoscalingGroupName = mapped
}
/**
* @param value The unique identifiers of a traffic sources.
*/
@JvmName("mlarbordgmgqmdgt")
public suspend fun trafficSource(`value`: TrafficSourceAttachmentTrafficSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.trafficSource = mapped
}
/**
* @param argument The unique identifiers of a traffic sources.
*/
@JvmName("eheaesmaaccpqobg")
public suspend fun trafficSource(argument: suspend TrafficSourceAttachmentTrafficSourceArgsBuilder.() -> Unit) {
val toBeMapped = TrafficSourceAttachmentTrafficSourceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.trafficSource = mapped
}
internal fun build(): TrafficSourceAttachmentArgs = TrafficSourceAttachmentArgs(
autoscalingGroupName = autoscalingGroupName,
trafficSource = trafficSource,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy