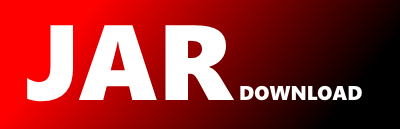
com.pulumi.aws.autoscaling.kotlin.inputs.PolicyPredictiveScalingConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscaling.kotlin.inputs
import com.pulumi.aws.autoscaling.inputs.PolicyPredictiveScalingConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property maxCapacityBreachBehavior Defines the behavior that should be applied if the forecast capacity approaches or exceeds the maximum capacity of the Auto Scaling group. Valid values are `HonorMaxCapacity` or `IncreaseMaxCapacity`. Default is `HonorMaxCapacity`.
* @property maxCapacityBuffer Size of the capacity buffer to use when the forecast capacity is close to or exceeds the maximum capacity. Valid range is `0` to `100`. If set to `0`, Amazon EC2 Auto Scaling may scale capacity higher than the maximum capacity to equal but not exceed forecast capacity.
* @property metricSpecification This structure includes the metrics and target utilization to use for predictive scaling.
* @property mode Predictive scaling mode. Valid values are `ForecastAndScale` and `ForecastOnly`. Default is `ForecastOnly`.
* @property schedulingBufferTime Amount of time, in seconds, by which the instance launch time can be advanced. Minimum is `0`.
*/
public data class PolicyPredictiveScalingConfigurationArgs(
public val maxCapacityBreachBehavior: Output? = null,
public val maxCapacityBuffer: Output? = null,
public val metricSpecification: Output,
public val mode: Output? = null,
public val schedulingBufferTime: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.autoscaling.inputs.PolicyPredictiveScalingConfigurationArgs = com.pulumi.aws.autoscaling.inputs.PolicyPredictiveScalingConfigurationArgs.builder()
.maxCapacityBreachBehavior(maxCapacityBreachBehavior?.applyValue({ args0 -> args0 }))
.maxCapacityBuffer(maxCapacityBuffer?.applyValue({ args0 -> args0 }))
.metricSpecification(
metricSpecification.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.mode(mode?.applyValue({ args0 -> args0 }))
.schedulingBufferTime(schedulingBufferTime?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PolicyPredictiveScalingConfigurationArgs].
*/
@PulumiTagMarker
public class PolicyPredictiveScalingConfigurationArgsBuilder internal constructor() {
private var maxCapacityBreachBehavior: Output? = null
private var maxCapacityBuffer: Output? = null
private var metricSpecification:
Output? = null
private var mode: Output? = null
private var schedulingBufferTime: Output? = null
/**
* @param value Defines the behavior that should be applied if the forecast capacity approaches or exceeds the maximum capacity of the Auto Scaling group. Valid values are `HonorMaxCapacity` or `IncreaseMaxCapacity`. Default is `HonorMaxCapacity`.
*/
@JvmName("xqpaqhxbiqadwdlo")
public suspend fun maxCapacityBreachBehavior(`value`: Output) {
this.maxCapacityBreachBehavior = value
}
/**
* @param value Size of the capacity buffer to use when the forecast capacity is close to or exceeds the maximum capacity. Valid range is `0` to `100`. If set to `0`, Amazon EC2 Auto Scaling may scale capacity higher than the maximum capacity to equal but not exceed forecast capacity.
*/
@JvmName("uobhakwaaibwpoch")
public suspend fun maxCapacityBuffer(`value`: Output) {
this.maxCapacityBuffer = value
}
/**
* @param value This structure includes the metrics and target utilization to use for predictive scaling.
*/
@JvmName("abxacsxswfpnqhiv")
public suspend fun metricSpecification(`value`: Output) {
this.metricSpecification = value
}
/**
* @param value Predictive scaling mode. Valid values are `ForecastAndScale` and `ForecastOnly`. Default is `ForecastOnly`.
*/
@JvmName("kupwuwbllnnoaiog")
public suspend fun mode(`value`: Output) {
this.mode = value
}
/**
* @param value Amount of time, in seconds, by which the instance launch time can be advanced. Minimum is `0`.
*/
@JvmName("koyaodnqdckrheys")
public suspend fun schedulingBufferTime(`value`: Output) {
this.schedulingBufferTime = value
}
/**
* @param value Defines the behavior that should be applied if the forecast capacity approaches or exceeds the maximum capacity of the Auto Scaling group. Valid values are `HonorMaxCapacity` or `IncreaseMaxCapacity`. Default is `HonorMaxCapacity`.
*/
@JvmName("lifrelyqsgcefipb")
public suspend fun maxCapacityBreachBehavior(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxCapacityBreachBehavior = mapped
}
/**
* @param value Size of the capacity buffer to use when the forecast capacity is close to or exceeds the maximum capacity. Valid range is `0` to `100`. If set to `0`, Amazon EC2 Auto Scaling may scale capacity higher than the maximum capacity to equal but not exceed forecast capacity.
*/
@JvmName("gxiuxpirbxrqhwfc")
public suspend fun maxCapacityBuffer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxCapacityBuffer = mapped
}
/**
* @param value This structure includes the metrics and target utilization to use for predictive scaling.
*/
@JvmName("uguenakwpcwgsswu")
public suspend fun metricSpecification(`value`: PolicyPredictiveScalingConfigurationMetricSpecificationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.metricSpecification = mapped
}
/**
* @param argument This structure includes the metrics and target utilization to use for predictive scaling.
*/
@JvmName("guudlyyaifshcuxy")
public suspend fun metricSpecification(argument: suspend PolicyPredictiveScalingConfigurationMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyPredictiveScalingConfigurationMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.metricSpecification = mapped
}
/**
* @param value Predictive scaling mode. Valid values are `ForecastAndScale` and `ForecastOnly`. Default is `ForecastOnly`.
*/
@JvmName("lvnvneddjvwftgwd")
public suspend fun mode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mode = mapped
}
/**
* @param value Amount of time, in seconds, by which the instance launch time can be advanced. Minimum is `0`.
*/
@JvmName("duqhinmyywlwgrbl")
public suspend fun schedulingBufferTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.schedulingBufferTime = mapped
}
internal fun build(): PolicyPredictiveScalingConfigurationArgs =
PolicyPredictiveScalingConfigurationArgs(
maxCapacityBreachBehavior = maxCapacityBreachBehavior,
maxCapacityBuffer = maxCapacityBuffer,
metricSpecification = metricSpecification ?: throw PulumiNullFieldException("metricSpecification"),
mode = mode,
schedulingBufferTime = schedulingBufferTime,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy