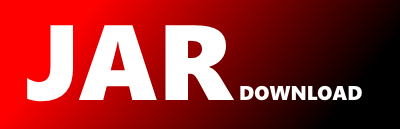
com.pulumi.aws.autoscaling.kotlin.inputs.PolicyPredictiveScalingConfigurationMetricSpecificationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscaling.kotlin.inputs
import com.pulumi.aws.autoscaling.inputs.PolicyPredictiveScalingConfigurationMetricSpecificationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property customizedCapacityMetricSpecification Customized capacity metric specification. The field is only valid when you use `customized_load_metric_specification`
* @property customizedLoadMetricSpecification Customized load metric specification.
* @property customizedScalingMetricSpecification Customized scaling metric specification.
* @property predefinedLoadMetricSpecification Predefined load metric specification.
* @property predefinedMetricPairSpecification Metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate scaling metric and load metric to use.
* @property predefinedScalingMetricSpecification Predefined scaling metric specification.
* @property targetValue Target value for the metric.
*/
public data class PolicyPredictiveScalingConfigurationMetricSpecificationArgs(
public val customizedCapacityMetricSpecification: Output? =
null,
public val customizedLoadMetricSpecification: Output? =
null,
public val customizedScalingMetricSpecification: Output? =
null,
public val predefinedLoadMetricSpecification: Output? =
null,
public val predefinedMetricPairSpecification: Output? =
null,
public val predefinedScalingMetricSpecification: Output? =
null,
public val targetValue: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.autoscaling.inputs.PolicyPredictiveScalingConfigurationMetricSpecificationArgs =
com.pulumi.aws.autoscaling.inputs.PolicyPredictiveScalingConfigurationMetricSpecificationArgs.builder()
.customizedCapacityMetricSpecification(
customizedCapacityMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.customizedLoadMetricSpecification(
customizedLoadMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.customizedScalingMetricSpecification(
customizedScalingMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.predefinedLoadMetricSpecification(
predefinedLoadMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.predefinedMetricPairSpecification(
predefinedMetricPairSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.predefinedScalingMetricSpecification(
predefinedScalingMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.targetValue(targetValue.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PolicyPredictiveScalingConfigurationMetricSpecificationArgs].
*/
@PulumiTagMarker
public class PolicyPredictiveScalingConfigurationMetricSpecificationArgsBuilder internal constructor() {
private var customizedCapacityMetricSpecification:
Output? =
null
private var customizedLoadMetricSpecification:
Output? =
null
private var customizedScalingMetricSpecification:
Output? =
null
private var predefinedLoadMetricSpecification:
Output? =
null
private var predefinedMetricPairSpecification:
Output? =
null
private var predefinedScalingMetricSpecification:
Output? =
null
private var targetValue: Output? = null
/**
* @param value Customized capacity metric specification. The field is only valid when you use `customized_load_metric_specification`
*/
@JvmName("yfbkipgavoastvav")
public suspend fun customizedCapacityMetricSpecification(`value`: Output) {
this.customizedCapacityMetricSpecification = value
}
/**
* @param value Customized load metric specification.
*/
@JvmName("plmuohxpfyclqfah")
public suspend fun customizedLoadMetricSpecification(`value`: Output) {
this.customizedLoadMetricSpecification = value
}
/**
* @param value Customized scaling metric specification.
*/
@JvmName("btdaqwlhqwxdncan")
public suspend fun customizedScalingMetricSpecification(`value`: Output) {
this.customizedScalingMetricSpecification = value
}
/**
* @param value Predefined load metric specification.
*/
@JvmName("rveutgogsikwhexu")
public suspend fun predefinedLoadMetricSpecification(`value`: Output) {
this.predefinedLoadMetricSpecification = value
}
/**
* @param value Metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate scaling metric and load metric to use.
*/
@JvmName("xiporynyrdtvvohx")
public suspend fun predefinedMetricPairSpecification(`value`: Output) {
this.predefinedMetricPairSpecification = value
}
/**
* @param value Predefined scaling metric specification.
*/
@JvmName("nbsvaaftvfbmhwek")
public suspend fun predefinedScalingMetricSpecification(`value`: Output) {
this.predefinedScalingMetricSpecification = value
}
/**
* @param value Target value for the metric.
*/
@JvmName("rpjwbfkegbibmuoc")
public suspend fun targetValue(`value`: Output) {
this.targetValue = value
}
/**
* @param value Customized capacity metric specification. The field is only valid when you use `customized_load_metric_specification`
*/
@JvmName("hjusjykxsnkldbil")
public suspend fun customizedCapacityMetricSpecification(`value`: PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedCapacityMetricSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customizedCapacityMetricSpecification = mapped
}
/**
* @param argument Customized capacity metric specification. The field is only valid when you use `customized_load_metric_specification`
*/
@JvmName("jrayxytdrxvoubrg")
public suspend fun customizedCapacityMetricSpecification(argument: suspend PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedCapacityMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedCapacityMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customizedCapacityMetricSpecification = mapped
}
/**
* @param value Customized load metric specification.
*/
@JvmName("lnriaamqwbrlnqpp")
public suspend fun customizedLoadMetricSpecification(`value`: PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customizedLoadMetricSpecification = mapped
}
/**
* @param argument Customized load metric specification.
*/
@JvmName("ichkailwydpghsow")
public suspend fun customizedLoadMetricSpecification(argument: suspend PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customizedLoadMetricSpecification = mapped
}
/**
* @param value Customized scaling metric specification.
*/
@JvmName("xnxqlsxectdfqthn")
public suspend fun customizedScalingMetricSpecification(`value`: PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedScalingMetricSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customizedScalingMetricSpecification = mapped
}
/**
* @param argument Customized scaling metric specification.
*/
@JvmName("qpyhbmvkbswnsaib")
public suspend fun customizedScalingMetricSpecification(argument: suspend PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedScalingMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedScalingMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customizedScalingMetricSpecification = mapped
}
/**
* @param value Predefined load metric specification.
*/
@JvmName("jkepmwounbcuwstt")
public suspend fun predefinedLoadMetricSpecification(`value`: PolicyPredictiveScalingConfigurationMetricSpecificationPredefinedLoadMetricSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.predefinedLoadMetricSpecification = mapped
}
/**
* @param argument Predefined load metric specification.
*/
@JvmName("ycnblypwlnvkohrq")
public suspend fun predefinedLoadMetricSpecification(argument: suspend PolicyPredictiveScalingConfigurationMetricSpecificationPredefinedLoadMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyPredictiveScalingConfigurationMetricSpecificationPredefinedLoadMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.predefinedLoadMetricSpecification = mapped
}
/**
* @param value Metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate scaling metric and load metric to use.
*/
@JvmName("nnwivkwljgowgrbg")
public suspend fun predefinedMetricPairSpecification(`value`: PolicyPredictiveScalingConfigurationMetricSpecificationPredefinedMetricPairSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.predefinedMetricPairSpecification = mapped
}
/**
* @param argument Metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate scaling metric and load metric to use.
*/
@JvmName("cetqgnruvmvchuwm")
public suspend fun predefinedMetricPairSpecification(argument: suspend PolicyPredictiveScalingConfigurationMetricSpecificationPredefinedMetricPairSpecificationArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyPredictiveScalingConfigurationMetricSpecificationPredefinedMetricPairSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.predefinedMetricPairSpecification = mapped
}
/**
* @param value Predefined scaling metric specification.
*/
@JvmName("nnwtwwyvghfjkheh")
public suspend fun predefinedScalingMetricSpecification(`value`: PolicyPredictiveScalingConfigurationMetricSpecificationPredefinedScalingMetricSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.predefinedScalingMetricSpecification = mapped
}
/**
* @param argument Predefined scaling metric specification.
*/
@JvmName("ivhwpfbsoxepumnf")
public suspend fun predefinedScalingMetricSpecification(argument: suspend PolicyPredictiveScalingConfigurationMetricSpecificationPredefinedScalingMetricSpecificationArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyPredictiveScalingConfigurationMetricSpecificationPredefinedScalingMetricSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.predefinedScalingMetricSpecification = mapped
}
/**
* @param value Target value for the metric.
*/
@JvmName("gfpnalxsoxltghbr")
public suspend fun targetValue(`value`: Double) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.targetValue = mapped
}
internal fun build(): PolicyPredictiveScalingConfigurationMetricSpecificationArgs =
PolicyPredictiveScalingConfigurationMetricSpecificationArgs(
customizedCapacityMetricSpecification = customizedCapacityMetricSpecification,
customizedLoadMetricSpecification = customizedLoadMetricSpecification,
customizedScalingMetricSpecification = customizedScalingMetricSpecification,
predefinedLoadMetricSpecification = predefinedLoadMetricSpecification,
predefinedMetricPairSpecification = predefinedMetricPairSpecification,
predefinedScalingMetricSpecification = predefinedScalingMetricSpecification,
targetValue = targetValue ?: throw PulumiNullFieldException("targetValue"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy