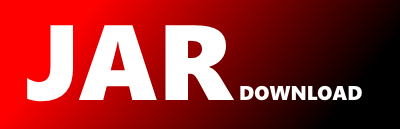
com.pulumi.aws.autoscaling.kotlin.inputs.PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscaling.kotlin.inputs
import com.pulumi.aws.autoscaling.inputs.PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property expression Math expression used on the returned metric. You must specify either `expression` or `metric_stat`, but not both.
* @property id Short name for the metric used in predictive scaling policy.
* @property label Human-readable label for this metric or expression.
* @property metricStat Structure that defines CloudWatch metric to be used in predictive scaling policy. You must specify either `expression` or `metric_stat`, but not both.
* @property returnData Boolean that indicates whether to return the timestamps and raw data values of this metric, the default is true
*/
public data class
PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryArgs(
public val expression: Output? = null,
public val id: Output,
public val label: Output? = null,
public val metricStat: Output? =
null,
public val returnData: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.autoscaling.inputs.PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryArgs =
com.pulumi.aws.autoscaling.inputs.PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryArgs.builder()
.expression(expression?.applyValue({ args0 -> args0 }))
.id(id.applyValue({ args0 -> args0 }))
.label(label?.applyValue({ args0 -> args0 }))
.metricStat(metricStat?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.returnData(returnData?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryArgs].
*/
@PulumiTagMarker
public class
PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryArgsBuilder
internal constructor() {
private var expression: Output? = null
private var id: Output? = null
private var label: Output? = null
private var metricStat:
Output? =
null
private var returnData: Output? = null
/**
* @param value Math expression used on the returned metric. You must specify either `expression` or `metric_stat`, but not both.
*/
@JvmName("xwbfahsgfjnhvsip")
public suspend fun expression(`value`: Output) {
this.expression = value
}
/**
* @param value Short name for the metric used in predictive scaling policy.
*/
@JvmName("nfrmxcrrjjapunlp")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value Human-readable label for this metric or expression.
*/
@JvmName("fongtjtqiopikcfi")
public suspend fun label(`value`: Output) {
this.label = value
}
/**
* @param value Structure that defines CloudWatch metric to be used in predictive scaling policy. You must specify either `expression` or `metric_stat`, but not both.
*/
@JvmName("cylhlfjckdesirey")
public suspend fun metricStat(`value`: Output) {
this.metricStat = value
}
/**
* @param value Boolean that indicates whether to return the timestamps and raw data values of this metric, the default is true
*/
@JvmName("rffsrxkglqvykmmr")
public suspend fun returnData(`value`: Output) {
this.returnData = value
}
/**
* @param value Math expression used on the returned metric. You must specify either `expression` or `metric_stat`, but not both.
*/
@JvmName("dfsvclmdppxsxpvs")
public suspend fun expression(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expression = mapped
}
/**
* @param value Short name for the metric used in predictive scaling policy.
*/
@JvmName("bxpfemogsbcfykmo")
public suspend fun id(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value Human-readable label for this metric or expression.
*/
@JvmName("itkxeqouoayqjjxd")
public suspend fun label(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.label = mapped
}
/**
* @param value Structure that defines CloudWatch metric to be used in predictive scaling policy. You must specify either `expression` or `metric_stat`, but not both.
*/
@JvmName("nfewclkouncnnrmj")
public suspend fun metricStat(`value`: PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryMetricStatArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricStat = mapped
}
/**
* @param argument Structure that defines CloudWatch metric to be used in predictive scaling policy. You must specify either `expression` or `metric_stat`, but not both.
*/
@JvmName("vrnilrwbvebtlyyd")
public suspend fun metricStat(argument: suspend PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryMetricStatArgsBuilder.() -> Unit) {
val toBeMapped =
PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryMetricStatArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.metricStat = mapped
}
/**
* @param value Boolean that indicates whether to return the timestamps and raw data values of this metric, the default is true
*/
@JvmName("jrpxakxujyqghvsu")
public suspend fun returnData(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.returnData = mapped
}
internal fun build(): PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryArgs =
PolicyPredictiveScalingConfigurationMetricSpecificationCustomizedLoadMetricSpecificationMetricDataQueryArgs(
expression = expression,
id = id ?: throw PulumiNullFieldException("id"),
label = label,
metricStat = metricStat,
returnData = returnData,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy