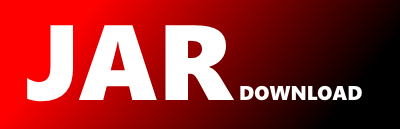
com.pulumi.aws.autoscaling.kotlin.inputs.PolicyStepAdjustmentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscaling.kotlin.inputs
import com.pulumi.aws.autoscaling.inputs.PolicyStepAdjustmentArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property metricIntervalLowerBound Lower bound for the
* difference between the alarm threshold and the CloudWatch metric.
* Without a value, AWS will treat this bound as negative infinity.
* @property metricIntervalUpperBound Upper bound for the
* difference between the alarm threshold and the CloudWatch metric.
* Without a value, AWS will treat this bound as positive infinity. The upper bound
* must be greater than the lower bound.
* Notice the bounds are **relative** to the alarm threshold, meaning that the starting point is not 0%, but the alarm threshold. Check the official [docs](https://docs.aws.amazon.com/autoscaling/ec2/userguide/as-scaling-simple-step.html#as-scaling-steps) for a detailed example.
* The following arguments are only available to "TargetTrackingScaling" type policies:
* @property scalingAdjustment Number of members by which to
* scale, when the adjustment bounds are breached. A positive value scales
* up. A negative value scales down.
*/
public data class PolicyStepAdjustmentArgs(
public val metricIntervalLowerBound: Output? = null,
public val metricIntervalUpperBound: Output? = null,
public val scalingAdjustment: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.autoscaling.inputs.PolicyStepAdjustmentArgs =
com.pulumi.aws.autoscaling.inputs.PolicyStepAdjustmentArgs.builder()
.metricIntervalLowerBound(metricIntervalLowerBound?.applyValue({ args0 -> args0 }))
.metricIntervalUpperBound(metricIntervalUpperBound?.applyValue({ args0 -> args0 }))
.scalingAdjustment(scalingAdjustment.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PolicyStepAdjustmentArgs].
*/
@PulumiTagMarker
public class PolicyStepAdjustmentArgsBuilder internal constructor() {
private var metricIntervalLowerBound: Output? = null
private var metricIntervalUpperBound: Output? = null
private var scalingAdjustment: Output? = null
/**
* @param value Lower bound for the
* difference between the alarm threshold and the CloudWatch metric.
* Without a value, AWS will treat this bound as negative infinity.
*/
@JvmName("ikoyiayvoqafbrxv")
public suspend fun metricIntervalLowerBound(`value`: Output) {
this.metricIntervalLowerBound = value
}
/**
* @param value Upper bound for the
* difference between the alarm threshold and the CloudWatch metric.
* Without a value, AWS will treat this bound as positive infinity. The upper bound
* must be greater than the lower bound.
* Notice the bounds are **relative** to the alarm threshold, meaning that the starting point is not 0%, but the alarm threshold. Check the official [docs](https://docs.aws.amazon.com/autoscaling/ec2/userguide/as-scaling-simple-step.html#as-scaling-steps) for a detailed example.
* The following arguments are only available to "TargetTrackingScaling" type policies:
*/
@JvmName("ujuqdeagwndeosqj")
public suspend fun metricIntervalUpperBound(`value`: Output) {
this.metricIntervalUpperBound = value
}
/**
* @param value Number of members by which to
* scale, when the adjustment bounds are breached. A positive value scales
* up. A negative value scales down.
*/
@JvmName("bpixlwbcuphpfgaf")
public suspend fun scalingAdjustment(`value`: Output) {
this.scalingAdjustment = value
}
/**
* @param value Lower bound for the
* difference between the alarm threshold and the CloudWatch metric.
* Without a value, AWS will treat this bound as negative infinity.
*/
@JvmName("riwutpkdycvivfbg")
public suspend fun metricIntervalLowerBound(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricIntervalLowerBound = mapped
}
/**
* @param value Upper bound for the
* difference between the alarm threshold and the CloudWatch metric.
* Without a value, AWS will treat this bound as positive infinity. The upper bound
* must be greater than the lower bound.
* Notice the bounds are **relative** to the alarm threshold, meaning that the starting point is not 0%, but the alarm threshold. Check the official [docs](https://docs.aws.amazon.com/autoscaling/ec2/userguide/as-scaling-simple-step.html#as-scaling-steps) for a detailed example.
* The following arguments are only available to "TargetTrackingScaling" type policies:
*/
@JvmName("lykokxgnvtsiemps")
public suspend fun metricIntervalUpperBound(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricIntervalUpperBound = mapped
}
/**
* @param value Number of members by which to
* scale, when the adjustment bounds are breached. A positive value scales
* up. A negative value scales down.
*/
@JvmName("kjaaocjverbreetr")
public suspend fun scalingAdjustment(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.scalingAdjustment = mapped
}
internal fun build(): PolicyStepAdjustmentArgs = PolicyStepAdjustmentArgs(
metricIntervalLowerBound = metricIntervalLowerBound,
metricIntervalUpperBound = metricIntervalUpperBound,
scalingAdjustment = scalingAdjustment ?: throw PulumiNullFieldException("scalingAdjustment"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy